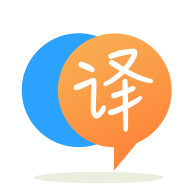
[英]How to add a custom HTTP header to ajax request with js or jQuery
[英]How can I add a custom HTTP header to ajax request with js or jQuery?
有谁知道如何使用JavaScript或jQuery添加或创建自定义HTTP标头?
根据您的需要,有几种解决方案......
如果要将自定义标头(或标头集)添加到单个请求,则只需添加headers
属性:
// Request with custom header
$.ajax({
url: 'foo/bar',
headers: { 'x-my-custom-header': 'some value' }
});
如果要为每个请求添加默认标头(或标头集),请使用$.ajaxSetup()
:
$.ajaxSetup({
headers: { 'x-my-custom-header': 'some value' }
});
// Sends your custom header
$.ajax({ url: 'foo/bar' });
// Overwrites the default header with a new header
$.ajax({ url: 'foo/bar', headers: { 'x-some-other-header': 'some value' } });
如果你想添加一个头(或套头)的每一个请求,然后使用beforeSend
用钩$.ajaxSetup()
$.ajaxSetup({
beforeSend: function(xhr) {
xhr.setRequestHeader('x-my-custom-header', 'some value');
}
});
// Sends your custom header
$.ajax({ url: 'foo/bar' });
// Sends both custom headers
$.ajax({ url: 'foo/bar', headers: { 'x-some-other-header': 'some value' } });
编辑(更多信息):需要注意的一点是,使用ajaxSetup
您只能定义一组默认标头,并且只能定义一个beforeSend
。 如果多次调用ajaxSetup
,则只会发送最后一组标题,并且只会执行最后一个before-send回调。
或者,如果要为每个将来的请求发送自定义标头,则可以使用以下内容:
$.ajaxSetup({
headers: { "CustomHeader": "myValue" }
});
这样,除非被请求的选项明确覆盖,否则每个未来的ajax请求都将包含自定义标头。 你可以在这里找到关于ajaxSetup
更多信息
以下是使用XHR2的示例:
function xhrToSend(){
// Attempt to creat the XHR2 object
var xhr;
try{
xhr = new XMLHttpRequest();
}catch (e){
try{
xhr = new XDomainRequest();
} catch (e){
try{
xhr = new ActiveXObject('Msxml2.XMLHTTP');
}catch (e){
try{
xhr = new ActiveXObject('Microsoft.XMLHTTP');
}catch (e){
statusField('\nYour browser is not' +
' compatible with XHR2');
}
}
}
}
xhr.open('POST', 'startStopResume.aspx', true);
xhr.setRequestHeader("chunk", numberOfBLObsSent + 1);
xhr.onreadystatechange = function (e) {
if (xhr.readyState == 4 && xhr.status == 200) {
receivedChunks++;
}
};
xhr.send(chunk);
numberOfBLObsSent++;
};
希望有所帮助。
如果创建对象,则可以使用setRequestHeader函数在发送请求之前指定名称和值。
假设JQuery ajax,您可以添加自定义标头,如 -
$.ajax({
url: url,
beforeSend: function(xhr) {
xhr.setRequestHeader("custom_header", "value");
},
success: function(data) {
}
});
您也可以在不使用jQuery的情况下执行此操作。 覆盖XMLHttpRequest的send方法并在那里添加标题:
XMLHttpRequest.prototype.realSend = XMLHttpRequest.prototype.send;
var newSend = function(vData) {
this.setRequestHeader('x-my-custom-header', 'some value');
this.realSend(vData);
};
XMLHttpRequest.prototype.send = newSend;
您应该避免使用文档中描述的$.ajaxSetup()
。 请改用以下内容:
$(document).ajaxSend(function(event, jqXHR, ajaxOptions) {
jqXHR.setRequestHeader('my-custom-header', 'my-value');
});
假设您的意思是“当使用ajax”和“一个HTTP 请求标头”时,请使用您传递给ajax()
的对象中的headers
属性
标题(添加1.5)
默认值:
{}
要与请求一起发送的其他标头键/值对的映射。 在调用beforeSend函数之前设置此设置; 因此,可以从beforeSend函数中覆盖标题设置中的任何值。
应该使用XMLHttpRequest对象的“setRequestHeader”方法
你可以使用js fetch
async function send(url,data) { let r= await fetch(url, { method: "POST", headers: { "My-header": "abc" }, body: JSON.stringify(data), }) return await r.json() } // Example usage let url='https://server.test-cors.org/server?enable=true&status=200&methods=POST&headers=my-header'; async function run() { let jsonObj = await send(url,{ some: 'testdata' }); console.log(jsonObj[0].request.httpMethod + ' was send - open chrome console > network to see it'); } run();
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.