[英]JavaScript hard refresh of current page
How can I force the web browser to do a hard refresh of the page via JavaScript?如何通过 JavaScript 强制 Web 浏览器硬刷新页面?
Hard refresh means getting a fresh copy of the page AND refresh all the external resources (images, JavaScript, CSS, etc.).硬刷新意味着获取页面的新副本并刷新所有外部资源(图像、JavaScript、CSS 等)。
Try to use:尝试使用:
location.reload(true);
When this method receives a true
value as argument, it will cause the page to always be reloaded from the server.当这个方法接收到一个
true
值作为参数时,它会导致页面总是从服务器重新加载。 If it is false or not specified, the browser may reload the page from its cache.如果为 false 或未指定,浏览器可能会从其缓存中重新加载页面。
More info:更多信息:
window.location.href = window.location.href
I was working on django , but nothing was working so this bypass worked for me , it's forwarding to the next so whenever you just want to come back it's Hard refreshing the page !我在 django 上工作,但没有任何效果,所以这个旁路对我有用,它正在转发到下一个,所以每当你想回来时,很难刷新页面! it's maybe not the proper way but can solve the issue , try it out and let me know !
这可能不是正确的方法,但可以解决问题,请尝试并告诉我!
window.history.forward(1);
For angular users and as found here , you can do the following:对于 angular 用户,如在此处找到的,您可以执行以下操作:
<form [action]="myAppURL" method="POST" #refreshForm></form>
import { Component, OnInit, ViewChild } from '@angular/core';
@Component({
// ...
})
export class FooComponent {
@ViewChild('refreshForm', { static: false }) refreshForm;
forceReload() {
this.refreshForm.nativeElement.submit();
}
}
The reason why it worked was explained on this website: https://www.xspdf.com/resolution/52192666.html这个网站上解释了它工作的原因: https : //www.xspdf.com/resolution/52192666.html
You'll also find how the hard reload works for every framework and more in this article您还将在本文中找到硬重新加载如何适用于每个框架以及更多内容
Location: reload(), The Location.reload() method reloads the current URL, like the Refresh button.
位置:reload(),Location.reload() 方法重新加载当前 URL,就像刷新按钮一样。 Using only location.reload();
仅使用 location.reload(); is not a solution if you want to perform a force-reload (as done with eg Ctrl + F5) in order to reload all resources from the server and not from the browser cache.
如果您想执行强制重新加载(例如使用 Ctrl + F5 完成)以便从服务器而不是浏览器缓存重新加载所有资源,这不是一个解决方案。 The solution to this issue is, to execute a POST request to the current location as this always makes the browser to reload everything.
此问题的解决方案是,对当前位置执行 POST 请求,因为这总是会使浏览器重新加载所有内容。
Accepted answer above no longer does anything except just a normal reloading on mostly new version of web browsers today.上面接受的答案不再做任何事情,只是在今天大多数新版本的网络浏览器上正常重新加载。 I've tried on my recently updated Chrome all those, including
location.reload(true)<\/code> ,
location.href = location.href<\/code> , and
<meta http-equiv="Cache-Control" content="no-cache, no-store, must-revalidate" \/><\/code> .
我已经在我最近更新的 Chrome 上尝试了所有这些,包括
location.reload(true)<\/code> 、
location.href = location.href<\/code>和
<meta http-equiv="Cache-Control" content="no-cache, no-store, must-revalidate" \/><\/code> 。
None of them worked.
他们都没有工作。
My solution is by using server-side capability to append non-repeating query string to all included source files reference as like below example.我的解决方案是使用服务器端功能将非重复查询字符串附加到所有包含的源文件引用,如下例所示。
<script src="script.js?t=<?=time();?>"><\/script><\/code>
So you also need to control it dynamically when to keep previous file and when to update it.因此,您还需要动态控制它何时保留以前的文件以及何时更新它。 The only issue is when files inclusion is performed via script by plugins you have no control to modify it.
唯一的问题是,当插件通过脚本执行文件包含时,您无法控制对其进行修改。 Don't worry about source files flooding.
不用担心源文件泛滥。 When older files are unlinked it will be automatically garbage collected.
当旧文件被取消链接时,它将自动被垃圾收集。
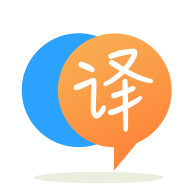
"
Changing the current URL with a search parameter will cause browsers to pass that same parameter to the server, which in other words, forces a refresh.使用搜索参数更改当前 URL 将导致浏览器将相同的参数传递给服务器,换句话说,强制刷新。
(No guarantees if you use intercept with a Service Worker though.) (不过,如果您使用 Service Worker 拦截,则无法保证。)
const url = new URL(window.location.href);
url.searchParams.set('reloadTime', Date.now().toString());
window.location.href = url.toString();
Hard refresh of current page.当前页面的硬刷新。 Put this at end of
<body>
:把它放在
<body>
的末尾:
<script>
var t = parseInt(Date.now() / 10000)
function addQuery(tag) {
const url = new URL(tag.href || tag.src);
url.searchParams.set('r', t.toString());
tag.href = url.toString();
}
function refresh() {
var x = localStorage.getItem("t");
localStorage.setItem("t", t);
if (x != t) addQuery(window.location)
else {
var a = document.querySelectorAll("a")
var n = a.length
while(n--) addQuery(a[n])
}
}
refresh()
</script>
It is a working code from my homepage that do a forced refresh for every visitor so that any update will show up without a cashing problem.这是我主页上的一个工作代码,它为每个访问者强制刷新,这样任何更新都会显示出来而不会出现兑现问题。
Extending to querySelectorAll("script, style, img, a") has a problem: The tags after querySelectorAll is not parsed and the script, style and img tags before querySelectorAll has already loaded or is loading.扩展到querySelectorAll("script, style, img, a") 有一个问题:querySelectorAll之后的标签没有被解析,而querySelectorAll之前的script、style和img标签已经加载或者正在加载。 Only the
a
tags is not yet loaded.只有
a
标签尚未加载。
The last time x
a refresh is done is stored in localStorage.上次完成刷新的
x
存储在 localStorage 中。 It is compared to the current time t
to prevent a page refresh within 10 seconds.它与当前时间
t
进行比较,以防止在 10 秒内刷新页面。 Assuming a parse not take more than 10 sec I managed to stop a page refresh loop.假设解析时间不超过 10 秒,我设法停止了页面刷新循环。 Here is how it works for the visitor:
以下是它对访问者的工作方式:
The time x != t
is true so the addQuery(window.location)
will make a hard refresh of page because a query string is added.时间
x != t
为真,因此addQuery(window.location)
将硬刷新页面,因为添加了查询字符串。
Because the hard refresh will be within 10 seconds the next time x != t
is false.因为下次
x != t
为 false 时,硬刷新将在 10 秒内进行。 In that case the addQuery
is used to add query strings to href
and src
.在这种情况下,
addQuery
用于将查询字符串添加到href
和src
。
This is a 2022 update with 2 methods, considering SPA's with #
in url:这是 2022 年的更新,有 2 种方法,考虑到在 url 中带有
#
的 SPA:
METHOD 1:方法一:
As mentioned in other answers one solution would be to put a random parameter to query string.如其他答案所述,一种解决方案是将随机参数放入查询字符串。 In javascript it could be achieved with this:
在 javascript 中,可以通过以下方式实现:
function urlWithRndQueryParam(url, paramName) {
const ulrArr = url.split('#');
const urlQry = ulrArr[0].split('?');
const usp = new URLSearchParams(urlQry[1] || '');
usp.set(paramName || '_z', `${Date.now()}`);
urlQry[1] = usp.toString();
ulrArr[0] = urlQry.join('?');
return ulrArr.join('#');
}
function handleHardReload(url) {
window.location.href = urlWithRndQueryParam(url);
// This is to ensure reload with url's having '#'
window.location.reload();
}
handleHardReload(window.location.href);
The bad part is that it changes the current url and sometimes, in clean url's, it could seem little bit ugly for users.不好的部分是它改变了当前的 url ,有时,在干净的 url 中,它对用户来说似乎有点难看。
METHOD 2:方法二:
Taking the idea from https://splunktool.com/force-a-reload-of-page-in-chrome-using-javascript-no-cache , the process could be to get the url without cache first and then reload the page: 借鉴 https://splunktool.com/force-a-reload-of-page-in-chrome-using-javascript-no-cache的想法,该过程可能是先获取没有缓存的 url 然后重新加载页面:
async function handleHardReload(url) {
await fetch(url, {
headers: {
Pragma: 'no-cache',
Expires: '-1',
'Cache-Control': 'no-cache',
},
});
window.location.href = url;
// This is to ensure reload with url's having '#'
window.location.reload();
}
handleHardReload(window.location.href);
Could be even combined with method 1, but I think that with headers should be enought:甚至可以与方法1结合使用,但我认为使用标题应该足够了:
async function handleHardReload(url) {
const newUrl = urlWithRndQueryParam(url);
await fetch(newUrl, {
headers: {
Pragma: 'no-cache',
Expires: '-1',
'Cache-Control': 'no-cache',
},
});
window.location.href = url;
// This is to ensure reload with url's having '#'
window.location.reload();
}
handleHardReload(window.location.href);
The most reliable way I've found is to use a chache buster by adding a value to the querystring.我发现的最可靠的方法是通过向查询字符串添加一个值来使用 chache buster。
Here's a generic routine that I use:这是我使用的通用例程:
function reloadUrl() {
// cache busting: Reliable but modifies URL
var queryParams = new URLSearchParams(window.location.search);
queryParams.set("lr", new Date().getTime());
var query = queryParams.toString();
window.location.search = query; // navigates
}
Calling this will produce something like this:调用它会产生这样的东西:
https://somesite.com/page?lr=1665958485293
after a reload.重新加载后。
This works to force reload every time, but the caveat is that the URL changes.这每次都强制重新加载,但需要注意的是 URL 会更改。 In most applications this won't matter, but if the server relies on specific parameters this can cause potential side effects.
在大多数应用程序中,这无关紧要,但如果服务器依赖于特定参数,这可能会导致潜在的副作用。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.