[英]Check/Uncheck checkbox with JavaScript
如何使用 JavaScript 选中/取消选中复选框?
Javascript: Javascript:
// Check
document.getElementById("checkbox").checked = true;
// Uncheck
document.getElementById("checkbox").checked = false;
jQuery (1.6+): jQuery (1.6+):
// Check
$("#checkbox").prop("checked", true);
// Uncheck
$("#checkbox").prop("checked", false);
jQuery (1.5-): jQuery (1.5-):
// Check
$("#checkbox").attr("checked", true);
// Uncheck
$("#checkbox").attr("checked", false);
Important behaviour that has not yet been mentioned:尚未提及的重要行为:
Programmatically setting the checked attribute, does not fire the change
event of the checkbox .以编程方式设置选中的属性,不会触发复选框的
change
事件。
See for yourself in this fiddle:在这个小提琴中自己看看:
http://jsfiddle.net/fjaeger/L9z9t04p/4/ http://jsfiddle.net/fjaeger/L9z9t04p/4/
(Fiddle tested in Chrome 46, Firefox 41 and IE 11) (小提琴在 Chrome 46、Firefox 41 和 IE 11 中测试)
click()
method click()
方法Some day you might find yourself writing code, which relies on the event being fired.有一天,您可能会发现自己在编写代码,这依赖于被触发的事件。 To make sure the event fires, call the
click()
method of the checkbox element, like this:要确保事件触发,请调用复选框元素的
click()
方法,如下所示:
document.getElementById('checkbox').click();
However, this toggles the checked status of the checkbox, instead of specifically setting it to true
or false
.但是,这会切换复选框的选中状态,而不是专门将其设置为
true
或false
。 Remember that the change
event should only fire, when the checked attribute actually changes.请记住,当Checked属性实际更改时,
change
事件应仅启动。
It also applies to the jQuery way: setting the attribute using prop
or attr
, does not fire the change
event.它也适用于 jQuery 方式:使用
prop
或attr
设置属性,不会触发change
事件。
checked
to a specific valuechecked
为特定值You could test the checked
attribute, before calling the click()
method.您可以在调用
click()
方法之前测试checked
的属性。 Example:例子:
function toggle(checked) {
var elm = document.getElementById('checkbox');
if (checked != elm.checked) {
elm.click();
}
}
Read more about the click method here:在此处阅读有关单击方法的更多信息:
https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/click https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/click
to check:去检查:
document.getElementById("id-of-checkbox").checked = true;
We can checked a particulate checkbox as,我们可以检查一个特定的复选框,
$('id of the checkbox')[0].checked = true
and uncheck by ,并取消选中,
$('id of the checkbox')[0].checked = false
Try This:试试这个:
//Check
document.getElementById('checkbox').setAttribute('checked', 'checked');
//UnCheck
document.getElementById('chk').removeAttribute('checked');
I would like to note, that setting the 'checked' attribute to a non-empty string leads to a checked box.我想指出,将 'checked' 属性设置为非空字符串会导致选中框。
So if you set the 'checked' attribute to "false"<\/em><\/strong> , the checkbox will be checked.因此,如果您将 'checked' 属性设置为"false"<\/em><\/strong> ,复选框将被选中。 I had to set the value to the empty string, null<\/em><\/strong> or the boolean value false<\/em><\/strong> in order to make sure the checkbox was not checked.
我必须将该值设置为空字符串、 null<\/em><\/strong>或布尔值false<\/em><\/strong> ,以确保未选中复选框。
function setCheckboxValue(checkbox,value) {
if (checkbox.checked!=value)
checkbox.click();
}
<script type="text/javascript">
$(document).ready(function () {
$('.selecctall').click(function (event) {
if (this.checked) {
$('.checkbox1').each(function () {
this.checked = true;
});
} else {
$('.checkbox1').each(function () {
this.checked = false;
});
}
});
});
</script>
For single check try对于单次检查尝试
![]()
myCheckBox.checked=1<\/code><\/pre>
<input type="checkbox" id="myCheckBox"> Call to her<\/code><\/pre>
for multi try多次尝试
document.querySelectorAll('.imChecked').forEach(c=> c.checked=1)<\/code><\/pre> Buy wine: <input type="checkbox" class="imChecked"><br> Play smooth-jazz music: <input type="checkbox"><br> Shave: <input type="checkbox" class="imChecked"><br><\/code><\/pre>
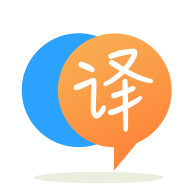
"
If, for some reason, you don't want to (or can't) run a .click()<\/code><\/a> on the checkbox element, you can simply change its value directly via its .checked property (an
IDL attribute<\/a> of
<input type="checkbox"><\/code><\/a> ).
如果出于某种原因,您不想(或不能)在复选框元素上运行
.click()<\/code><\/a> ,您可以直接通过其 .checked 属性(
<input type="checkbox"><\/code><\/a>的
IDL 属性)<\/a>直接更改其值
<input type="checkbox"><\/code><\/a> )。
Note<\/strong> that doing so does not fire the normally related event (
change<\/a> ) so you'll need to manually fire it to have a complete solution that works with any related event handlers.请注意<\/strong>,这样做不会触发通常相关的事件 (
change<\/a> ),因此您需要手动触发它以获得可与任何相关事件处理程序一起使用的完整解决方案。
Here's a functional example in raw javascript (ES6):这是原始 javascript (ES6) 中的一个功能示例:
"
Using vanilla js:使用香草js:
//for one element:
document.querySelector('.myCheckBox').checked = true //will select the first matched element
document.querySelector('.myCheckBox').checked = false//will unselect the first matched element
//for multiple elements:
for (const checkbox of document.querySelectorAll('.myCheckBox')) {
//iterating over all matched elements
checkbox.checked = true //for selection
checkbox.checked = false //for unselection
}
I agree with the current answers, but in my case it does not work, I hope this code help someone in the future:我同意当前的答案,但在我的情况下它不起作用,我希望这段代码将来能对某人有所帮助:
// check
$('#checkbox_id').click()
If you want to use the TypeScript, you can do like this: 如果要使用TypeScript,可以这样做:
function defaultCheckedFirst(checkGroup: any): void { for (let i = 0; i < checkGroup.length; i++) { (<HTMLInputElement>radionGroups[i]).checked = (i === 0 ? true : false); } }
vanilla (PHP) will check box on and off and store result. vanilla (PHP) 将打开和关闭复选框并存储结果。
<?php
if($serverVariable=="checked")
$checked = "checked";
else
$checked = "";
?>
<input type="checkbox" name="deadline" value="yes" <?= $checked
?> >
# on server
<?php
if(isset($_POST['deadline']) and
$_POST['deadline']=='yes')
$serverVariable = checked;
else
$serverVariable = "";
?>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.