[英]Uncaught SyntaxError: Unexpected token with JSON.parse
what causes this error on the third line?是什么导致第三行出现此错误?
![]()
var products = [{ "name": "Pizza", "price": "10", "quantity": "7" }, { "name": "Cerveja", "price": "12", "quantity": "5" }, { "name": "Hamburguer", "price": "10", "quantity": "2" }, { "name": "Fraldas", "price": "6", "quantity": "2" }]; console.log(products); var b = JSON.parse(products); \/\/unexpected token o<\/code><\/pre>
Open console to view error<\/sup>打开控制台查看错误<\/sup>
"
products<\/code> is an object.
products<\/code>是一个对象。
(creating from an object literal)
(从对象字面量创建)
JSON.parse()<\/code> is used to convert a string<\/strong> containing JSON notation into a Javascript object.
JSON.parse()<\/code>用于将包含 JSON 表示法的字符串<\/strong>转换为 Javascript 对象。
Your code turns the object into a string (by calling
.toString()<\/code> ) in order to try to parse it as JSON text.
您的代码将对象转换为字符串(通过调用
.toString()<\/code> ),以便尝试将其解析为 JSON 文本。
The default .toString()<\/code> returns
"[object Object]"<\/code> , which is not valid JSON;
默认的
.toString()<\/code>返回
"[object Object]"<\/code> ,这是无效的 JSON;
hence the error.
因此错误。
"
Let's say you know it's valid JSON but your are still getting this...假设你知道它是有效的 JSON,但你仍然得到这个......
In that case it's likely that there are hidden\/special characters in the string from whatever source your getting them.在这种情况下,字符串中可能有隐藏\/特殊字符来自您获取它们的任何来源。 When you paste into a validator, they are lost - but in the string they are still there.
当您粘贴到验证器中时,它们会丢失 - 但在字符串中它们仍然存在。 Those chars, while invisible, will break
JSON.parse()<\/code>
这些字符虽然不可见,但会破坏
JSON.parse()<\/code>
If
s<\/code> is your raw JSON, then clean it up with:
如果
s<\/code>是您的原始 JSON,则使用以下命令对其进行清理:
// preserve newlines, etc - use valid JSON
s = s.replace(/\\n/g, "\\n")
.replace(/\\'/g, "\\'")
.replace(/\\"/g, '\\"')
.replace(/\\&/g, "\\&")
.replace(/\\r/g, "\\r")
.replace(/\\t/g, "\\t")
.replace(/\\b/g, "\\b")
.replace(/\\f/g, "\\f");
// remove non-printable and other non-valid JSON chars
s = s.replace(/[\u0000-\u0019]+/g,"");
var o = JSON.parse(s);
It seems you want to stringify<\/strong> the object, not parse.似乎您想对对象进行字符串化<\/strong>,而不是解析。 So do this:
所以这样做:
JSON.stringify(products);
I found the same issue with JSON.parse(inputString)<\/code> .
我发现与
JSON.parse(inputString)<\/code>相同的问题。
In my case the input string is coming from my server page [return of a page method]<\/em> .在我的情况下,输入字符串来自我的服务器页面[return of a page method]<\/em> 。
I printed the
typeof(inputString)<\/code> - it was string, still the error occurs.
我打印了
typeof(inputString)<\/code> - 它是字符串,仍然发生错误。
I also tried
JSON.stringify(inputString)<\/code> , but it did not help.
我也试过
JSON.stringify(inputString)<\/code> ,但没有帮助。
Later I found this to be an issue with the new line operator
[\\n]<\/code> , inside a field value.
后来我发现这是字段值内的换行符
[\\n]<\/code>的问题。
I did a replace [with some other character, put the new line back after parse]<\/em> and everything is working fine.我做了一个替换[用其他字符,在解析后放回新行]<\/em> ,一切正常。
"
JSON.parse is waiting for a String in parameter. JSON.parse 正在等待参数中的字符串。 You need to stringify your JSON object to solve the problem.
您需要对 JSON 对象进行字符串化以解决问题。
products = [{"name":"Pizza","price":"10","quantity":"7"}, {"name":"Cerveja","price":"12","quantity":"5"}, {"name":"Hamburguer","price":"10","quantity":"2"}, {"name":"Fraldas","price":"6","quantity":"2"}];
console.log(products);
var b = JSON.parse(JSON.stringify(products)); //solves the problem
You should validate your JSON string here<\/a> .您应该
在此处<\/a>验证您的 JSON 字符串。
A valid JSON string must have double quotes around the keys:有效的 JSON 字符串的键必须有双引号:
JSON.parse({"u1":1000,"u2":1100}) // will be ok
products = [{"name":"Pizza","price":"10","quantity":"7"}, {"name":"Cerveja","price":"12","quantity":"5"}, {"name":"Hamburguer","price":"10","quantity":"2"}, {"name":"Fraldas","price":"6","quantity":"2"}];
If there are leading or trailing spaces, it'll be invalid.如果有前导或尾随空格,则无效。 Trailing\/Leading spaces can be removed as
尾随\/前导空格可以删除为
mystring = mystring.replace(/^\s+|\s+$/g, "");
Here's a function I made based on previous replies: it works on my machine but YMMV.这是我根据以前的回复制作的一个功能:它可以在我的机器上运行,但是 YMMV。
/**
* @description Converts a string response to an array of objects.
* @param {string} string - The string you want to convert.
* @returns {array} - an array of objects.
*/
function stringToJson(input) {
var result = [];
//replace leading and trailing [], if present
input = input.replace(/^\[/,'');
input = input.replace(/\]$/,'');
//change the delimiter to
input = input.replace(/},{/g,'};;;{');
// preserve newlines, etc - use valid JSON
//https://stackoverflow.com/questions/14432165/uncaught-syntaxerror-unexpected-token-with-json-parse
input = input.replace(/\\n/g, "\\n")
.replace(/\\'/g, "\\'")
.replace(/\\"/g, '\\"')
.replace(/\\&/g, "\\&")
.replace(/\\r/g, "\\r")
.replace(/\\t/g, "\\t")
.replace(/\\b/g, "\\b")
.replace(/\\f/g, "\\f");
// remove non-printable and other non-valid JSON chars
input = input.replace(/[\u0000-\u0019]+/g,"");
input = input.split(';;;');
input.forEach(function(element) {
// console.log(JSON.stringify(element));
result.push(JSON.parse(element));
}, this);
return result;
}
One other gotcha that can result in "SyntaxError: Unexpected token"<\/code> exception when calling
JSON.parse()<\/code> is using any of the following in the string values:
调用
JSON.parse()<\/code>时可能导致
"SyntaxError: Unexpected token"<\/code>异常的另一个问题是在字符串值中使用以下任何内容:
New-line characters.换行符。
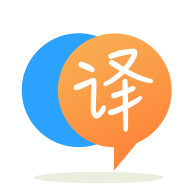
<\/li>
Tabs (yes, tabs that you can produce with the Tab key!)选项卡(是的,您可以使用 Tab 键生成的选项卡!)
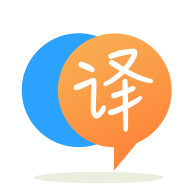
<\/li>
Any stand-alone slash
\\<\/code> (but for some reason not
\/<\/code> , at least not on Chrome.)
任何独立的斜线
\\<\/code> (但由于某种原因不是
\/<\/code> ,至少不是在 Chrome 上。)
<\/li><\/ol>
(For a full list see the String section here<\/a> .) (有关完整列表,请参见
此处的字符串部分<\/a>。)
For instance the following will get you this exception:例如,以下内容将为您提供此异常:
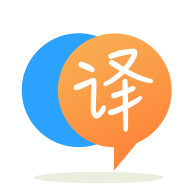
{ "msg" : { "message": "It cannot contain a new-line", "description": "Some discription with a tabbed space is also bad", "value": "It cannot have 3\\4 un-escaped" } }<\/code><\/pre> So it should be changed to:所以应该改为:
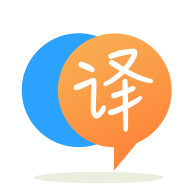
{ "msg" : { "message": "It cannot\\ncontain a new-line", "description": "Some discription with a\\t\\ttabbed space", "value": "It cannot have 3\\\\4 un-escaped" } }<\/code><\/pre> Which, I should say, makes it quite unreadable in JSON-only format with larger amount of text.我应该说,这使得它在带有大量文本的纯 JSON 格式中非常不可读。
"
[
{
"name": "Pizza",
"price": "10",
"quantity": "7"
},
{
"name": "Cerveja",
"price": "12",
"quantity": "5"
},
{
"name": "Hamburguer",
"price": "10",
"quantity": "2"
},
{
"name": "Fraldas",
"price": "6",
"quantity": "2"
}
]
Hopefully this helps someone else.希望这对其他人有帮助。
My issue was that I had commented HTML in a PHP callback function via AJAX that was parsing the comments and return invalid JSON.我的问题是我通过 AJAX 在 PHP 回调函数中对 HTML 进行了注释,该函数正在解析注释并返回无效的 JSON。
Once I removed the commented HTML, all was good and the JSON was parsed with no issues.一旦我删除了注释的 HTML,一切都很好,JSON 被解析没有问题。
When you are using POST or PUT method, make sure to stringify the body part.当您使用 POST 或 PUT 方法时,请确保对正文部分进行字符串化。
I have documented an example here https:\/\/gist.github.com\/manju16832003\/4a92a2be693a8fda7ca84b58b8fa7154<\/a>我在这里记录了一个示例
https:\/\/gist.github.com\/manju16832003\/4a92a2be693a8fda7ca84b58b8fa7154<\/a>
The only mistake you are doing is, you are parsing already parsed object so it's throwing error, use this and you will be good to go.您正在做的唯一错误是,您正在解析已经解析的对象,所以它会抛出错误,使用它,您会很高兴。
![]()
var products = [{ "name": "Pizza", "price": "10", "quantity": "7" }, { "name": "Cerveja", "price": "12", "quantity": "5" }, { "name": "Hamburguer", "price": "10", "quantity": "2" }, { "name": "Fraldas", "price": "6", "quantity": "2" }]; console.log(products[0].name); \/\/name of item at 0th index<\/code><\/pre>
if you want to print entire json then use JSON.stringify()如果要打印整个 json,请使用 JSON.stringify()
"
products is an array which can be used directly: products 是一个可以直接使用的数组:
var i, j;
for(i=0;i<products.length;i++)
for(j in products[i])
console.log("property name: " + j,"value: "+products[i][j]);
Now apparently \\r<\/code> ,
\\b<\/code> ,
\\t<\/code> ,
\\f<\/code> , etc aren't the only problematic chars that can give you this error.
现在显然
\\r<\/code> ,
\\b<\/code> ,
\\t<\/code> ,
\\f<\/code>等不是唯一可以给你这个错误的有问题的字符。
Note that some browsers may have additional<\/em> requirements for the input of
JSON.parse<\/code> .
请注意,某些浏览器可能对
JSON.parse<\/code>的输入有额外<\/em>的要求。
Run this test code on your browser:在浏览器上运行此测试代码:
var arr = [];
for(var x=0; x < 0xffff; ++x){
try{
JSON.parse(String.fromCharCode(0x22, x, 0x22));
}catch(e){
arr.push(x);
}
}
console.log(arr);
Oh man, solutions in all above answers provided so far didn't work for me.哦,伙计,到目前为止提供的所有上述答案中的解决方案都对我不起作用。 I had a similar problem just now.
我刚才也有类似的问题。 I managed to solve it with wrapping with the quote.
我设法用引号来解决它。 See the screenshot.
请参阅屏幕截图。 Whoo.
哇。
Original:<\/strong>原来的:<\/strong>
"
The error you are getting ie "unexpected token o" is because json is expected but object is obtained while parsing.您得到的错误,即“意外的令牌 o”是因为 json 是预期的,但在解析时获取了对象。 That "o" is the first letter of word "object".
那个“o”是单词“object”的第一个字母。
It can happen for a lot of reasons, but probably for an invalid char, so you can use JSON.stringify(obj);<\/code>
发生这种情况的原因有很多,但可能是因为字符无效,因此您可以使用
JSON.stringify(obj);<\/code>
that will turn your object into a JSON but remember that it is a JQUERY expression.
这会将您的对象转换为 JSON,但请记住它是 JQUERY 表达式。
"
I have this error BECAUSE the API that returned the json object was giving AN ERROR (in my case Code Igniter, return an html when the php code fails) so IT'S NOT AN JSON OBJECT. 我有这个错误,因为返回json对象的API给出了一个错误(在我的情况下Code Igniter,当php代码失败时返回一个html)所以它不是JSON OBJECT。
Check the SQL Sentences and the PHP code, and test it with Postman (or some other API tester) 检查SQL Sentences和PHP代码,并使用Postman(或其他一些API测试人员)进行测试
In my case there is following character problems in my JSON<\/code> string
在我的情况下,我的
JSON<\/code>字符串中存在以下字符问题
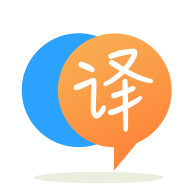
\\r \\r<\/li>
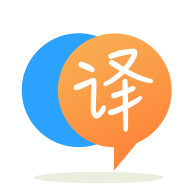
\\t \\t<\/li>
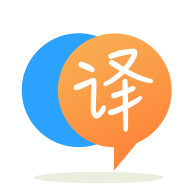
\\r\\n \\r\\n<\/li>
\\n \\n<\/li>
: :<\/li>
" "<\/li><\/ol> I have replaced them with another characters or symbols, and then revert back again from coding.
我已将它们替换为其他字符或符号,然后再次从编码中恢复。
"
This is now a JavaScript Array of Object, not JSON format.现在这是一个 JavaScript 对象数组,而不是 JSON 格式。 TO convert it into JSON format you need to use a function called JSON.stringify()<\/strong>
要将其转换为 JSON 格式,您需要使用名为JSON.stringify()<\/strong>的函数
JSON.stringify(products)
Why you need JSON.parse?为什么需要 JSON.parse? It's already in array of object format.
它已经在对象格式的数组中。
Better use JSON.stringify as below :
var b = JSON.stringify(products);<\/code>
更好地使用 JSON.stringify 如下:
var b = JSON.stringify(products);<\/code>
This may help you.这可能会对您有所帮助。
"
The mistake I was doing was passing null<\/code> (unknowingly) into JSON.parse().
我正在做的错误是将
null<\/code> (在不知不觉中)传递给 JSON.parse()。
So it threw
Unexpected token n in JSON at position 0<\/code>
所以它
Unexpected token n in JSON at position 0<\/code>
But this happens whenever you pass something which is not JS Object in JSON.parse()但是,每当您在 JSON.parse() 中传递不是 JS 对象的东西时,就会发生这种情况
"
what causes this error on the third line?是什么导致第三行出现此错误?
var products = [{ "name": "Pizza", "price": "10", "quantity": "7" }, { "name": "Cerveja", "price": "12", "quantity": "5" }, { "name": "Hamburguer", "price": "10", "quantity": "2" }, { "name": "Fraldas", "price": "6", "quantity": "2" }]; console.log(products); var b = JSON.parse(products); //unexpected token o
Open console to view error打开控制台以查看错误
what causes this error on the third line?是什么导致第三行出现此错误?
var products = [{ "name": "Pizza", "price": "10", "quantity": "7" }, { "name": "Cerveja", "price": "12", "quantity": "5" }, { "name": "Hamburguer", "price": "10", "quantity": "2" }, { "name": "Fraldas", "price": "6", "quantity": "2" }]; console.log(products); var b = JSON.parse(products); //unexpected token o
Open console to view error打开控制台以查看错误
Use eval<\/code> .
使用
eval<\/code> 。
It takes JavaScript expression\/code as string and evaluates\/executes it.
它将 JavaScript 表达式\/代码作为字符串并评估\/执行它。
eval(inputString);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.