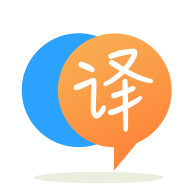
[英]How can I deserialize any xml element to a list via the XmlSerializer?
[英]How can I deserialize an XML response to an object when I only need a child element of the XML?
我从WebRequest中获取以下XML:
<?xml version="1.0" encoding="UTF-8"?>
<search>
<total_items>5</total_items>
<page_size>10</page_size>
<page_count>1</page_count>
<page_number>1</page_number>
<page_items></page_items>
<first_item></first_item>
<last_item></last_item>
<search_time>0.044</search_time>
<events>
<event id="E0-001-053379749-0">
<title>Antibalas</title>
<url>http://test.com</url>
<description>stuff</description>
<start_time>2013-01-30 20:00:00</start_time>
<stop_time></stop_time>
<tz_id></tz_id>
<tz_olson_path></tz_olson_path>
<tz_country></tz_country>
<tz_city></tz_city>
<venue_id>V0-001-000189211-5</venue_id>
<venue_url>http://blah.com</venue_url>
<venue_name>Troubadour</venue_name>
<venue_display>1</venue_display>
<venue_address>9081 Santa Monica Boulevard</venue_address>
<city_name>West Hollywood</city_name>
<region_name>California</region_name>
<region_abbr>CA</region_abbr>
<postal_code>90069</postal_code>
<country_name>United States</country_name>
<country_abbr2>US</country_abbr2>
<country_abbr>USA</country_abbr>
<latitude>34.0815917</latitude>
<longitude>-118.3892462</longitude>
<geocode_type>EVDB Geocoder</geocode_type>
<all_day>0</all_day>
<recur_string></recur_string>
<calendar_count></calendar_count>
<comment_count></comment_count>
<link_count></link_count>
<going_count></going_count>
<watching_count></watching_count>
<created>2012-12-24 11:40:43</created>
<owner>evdb</owner>
<modified>2013-01-14 21:08:04</modified>
<performers>
<performer>
<id>P0-001-000000517-4</id>
<url>http://test.com</url>
<name>Antibalas</name>
<short_bio>Afro-beat / Funk / Experimental</short_bio>
<creator>jfisher</creator>
<linker>evdb</linker>
</performer>
</performers>
<image>
<url>http://s4.evcdn.com/images/small/I0-001/000/070/311-5.jpeg_/antibalas-11.jpeg</url>
<width>48</width>
<height>48</height>
<caption></caption>
<thumb>
<url>http://s4.evcdn.com/images/thumb/I0-001/000/070/311-5.jpeg_/antibalas-11.jpeg</url>
<width>48</width>
<height>48</height>
</thumb>
<small>
<url>http://s4.evcdn.com/images/small/I0-001/000/070/311-5.jpeg_/antibalas-11.jpeg</url>
<width>48</width>
<height>48</height>
</small>
<medium>
<url>http://s4.evcdn.com/images/medium/I0-001/000/070/311-5.jpeg_/antibalas-11.jpeg</url>
<width>128</width>
<height>128</height>
</medium>
</image>
<privacy>1</privacy>
<calendars></calendars>
<groups></groups>
<going></going>
</event>
</events>
</search>
我创建了以下类:
public class EventSearch
{
public int total_items { get; set; }
public int page_size { get; set; }
public int page_count { get; set; }
public int page_number { get; set; }
public int page_items { get; set; }
public string first_item { get; set; }
public string last_item { get; set; }
public string search_time { get; set; }
public List<Event> events { get; set; }
}
public class Event
{
public string id { get; set; }
public string title { get; set; }
public string url { get; set; }
public string description { get; set; }
public DateTime start_time { get; set; }
public string venue_id { get; set; }
public string venue_url { get; set; }
public string venue_name { get; set; }
public string venue_address { get; set; }
public string city_name { get; set; }
public string region_name { get; set; }
public string region_abbr { get; set; }
public string postal_code { get; set; }
public string country_name { get; set; }
public string country_abbr { get; set; }
public string country_abbr2 { get; set; }
public double latitude { get; set; }
public double longitude { get; set; }
public List<Performer> performers { get; set; }
public EventImage image { get; set; }
}
public class Performer
{
public string id { get; set; }
public string url { get; set; }
public string name { get; set; }
public string short_bio { get; set; }
}
public class EventImage
{
public string url { get; set; }
public int width { get; set; }
public int height { get; set; }
public Image thumb { get; set; }
public Image small { get; set; }
public Image medium { get; set; }
}
public class Image
{
public string url { get; set; }
public int width { get; set; }
public int height { get; set; }
}
在我要调用的方法中,我想返回事件列表。 到目前为止,这是给我的错误:
没想到。
码:
var request = WebRequest.Create(url);
var response = request.GetResponse();
if (((HttpWebResponse)response).StatusCode == HttpStatusCode.OK)
{
// Get the stream containing content returned by the server.
Stream dataStream = response.GetResponseStream();
// Open the stream using a StreamReader.
StreamReader reader = new StreamReader(dataStream);
XmlSerializer serializer = new XmlSerializer(typeof(EventSearch));
EventSearch deserialized = (EventSearch)serializer.Deserialize(reader);
return deserialized.events;
}
我不确定下一步该怎么做。 我需要注释我的课程吗?
是的,你将需要添加一些Attributes
到您的classes
的XML元素到你的对象映射。
这应该可以工作,但是我不得不更改一些东西,Xml中的DateTime是错误的fromat,所以我将其设置为字符串(可以根据需要进行解析), page_items
也是一个int
但是它是一个空元素xml,您将无法反序列化它,如果它有可能为空,则必须更改为字符串。
[XmlType("search")]
public class EventSearch
{
public int total_items { get; set; }
public int page_size { get; set; }
public int page_count { get; set; }
public int page_number { get; set; }
// public int? page_items { get; set; }
public string first_item { get; set; }
public string last_item { get; set; }
public string search_time { get; set; }
public List<Event> events { get; set; }
}
[XmlType("event")]
public class Event
{
[XmlAttribute("id")]
public string id { get; set; }
public string title { get; set; }
public string url { get; set; }
public string description { get; set; }
public string start_time { get; set; }
public string venue_id { get; set; }
public string venue_url { get; set; }
public string venue_name { get; set; }
public string venue_address { get; set; }
public string city_name { get; set; }
public string region_name { get; set; }
public string region_abbr { get; set; }
public string postal_code { get; set; }
public string country_name { get; set; }
public string country_abbr { get; set; }
public string country_abbr2 { get; set; }
public double latitude { get; set; }
public double longitude { get; set; }
public List<Performer> performers { get; set; }
public EventImage image { get; set; }
}
[XmlType("performer")]
public class Performer
{
public string id { get; set; }
public string url { get; set; }
public string name { get; set; }
public string short_bio { get; set; }
}
[XmlType("image")]
public class EventImage
{
public string url { get; set; }
public int width { get; set; }
public int height { get; set; }
public Image thumb { get; set; }
public Image small { get; set; }
public Image medium { get; set; }
}
[XmlType("thumb")]
public class Image
{
public string url { get; set; }
public int width { get; set; }
public int height { get; set; }
}
我直接使用以下文件从文件中进行了测试:
using (FileStream stream = new FileStream("C:\\Test.xml", FileMode.Open))
{
XmlSerializer serializer = new XmlSerializer(typeof(EventSearch));
EventSearch deserialized = (EventSearch)serializer.Deserialize(stream);
}
但是您来自Webresponce
的Stream
应与FileStream
相同
希望这可以帮助 :)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.