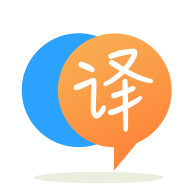
[英]How to pass same String value from adapter to two different activities
[英]How do you pass String value using button from one activity to two different activities?
我想通过单击按钮将从EditText获得的值传递给两个活动。 我设法将值传递给一个活动,将其显示在textview中,我想对另一个活动进行同样的操作,但由于只有一个按钮,我不确定切换大小写是否是正确的使用方法。
这是第一个活动的代码:
public class ProfInfo1 extends Activity {
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.profinfo1);
final EditText et0 = (EditText) findViewById (R.id.et_name);
final EditText et1 = (EditText) findViewById (R.id.et_age);
final EditText et2 = (EditText) findViewById (R.id.et_height);
final EditText et3 = (EditText) findViewById (R.id.et_weight);
Button back = (Button) findViewById(R.id.btn_back1);
back.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
Intent a = new Intent(ProfInfo1.this, WelcomeActivity.class);
startActivity(a);
}
});
Button next = (Button) findViewById(R.id.btn_next1);
next.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(ProfInfo1.this, ViewProf1.class);
intent.putExtra("name", et0.getText().toString());
intent.putExtra("age", et1.getText().toString());
intent.putExtra("height", et2.getText().toString());
intent.putExtra("weight", et3.getText().toString());
startActivity(intent);
}
});
}
}
这是第二个显示值的活动:
public class ViewProf1 extends Activity {
EditText age, height, weight;
String a, h, w;
Float age1, height1, weight1;
float bmi, cal;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.profinfo1view);
TextView nameView = (TextView) findViewById (R.id.view_name);
nameView.setText("Hello " + getIntent().getExtras().getString("name") + ",");
TextView ageView = (TextView) findViewById (R.id.view_age);
ageView.setText("Age: " + getIntent().getExtras().getString("age"));
TextView heightView = (TextView) findViewById (R.id.view_height);
heightView.setText("Your current height is " + getIntent().getExtras().getString("height") + " m.");
TextView weightView = (TextView) findViewById (R.id.view_weight);
weightView.setText("Your current weight is " + getIntent().getExtras().getString("weight") + " kg.");
a = getIntent().getExtras().getString("age");
h = getIntent().getExtras().getString("height");
w = getIntent().getExtras().getString("weight");
age1 = Float.valueOf(a).floatValue();
height1 = Float.valueOf(h).floatValue();
weight1 = Float.valueOf(w).floatValue();
//bmi calculation
bmi = (float) (weight1/Math.pow(height1, 2));
TextView showresult = (TextView) findViewById (R.id.view_bmi);
showresult.setText("Your BMI is " + Float.valueOf(bmi) + ".");
//cal intake calculation
cal = (float) (((655 + (9.6 * weight1) + (1.8 * height1) - (4.7 * age1)) * 1.2) - 550);
TextView showcal = (TextView) findViewById (R.id.view_cal);
showcal.setText("Your ideal daily calories intake is " + Float.valueOf(cal) + ".");
//next button
Button next2 = (Button) findViewById (R.id.btn_next2);
next2.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
Intent a = new Intent(ViewProf1.this, FoodDiary.class);
startActivity(a);
}
});
//back button
Button back2 = (Button) findViewById (R.id.btn_back2);
back2.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
Intent a = new Intent(ViewProf1.this, ProfInfo1.class);
startActivity(a);
}
});
}
第三项活动:
public class CompareWeight extends Activity {
EditText weight;
String w;
Float weight1;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.compareweight);
TextView weightView = (TextView) findViewById (R.id.test);
weightView.setText("Your previous weight is " + getIntent().getExtras().getString("weight") + " kg.");
w = getIntent().getExtras().getString("weight");
weight1 = Float.valueOf(w).floatValue();
}
}
我只想将权重值传递给第三项活动。
您一次只能将值传递给一个活动。但是,如果要将权重值传递给第三个活动,则可以在第一个活动中设置其值,然后在第三个活动中进行检索。 所以基本上我的意思是
您可以将值保存在“共享首选项”中,然后更新值。o单击“按钮”。这样,其他活动将可以使用这些值。
在onPause
中将SharedPreferences
用于当前Activity
,如下所示,并将数据发送到其他活动,如下所示:
@Override
protected void onPause()
{
super.onPause();
SharedPreferences preferences = getSharedPreferences("sharedPrefs", 0);
SharedPreferences.Editor editor = preferences.edit();
editor.putString("SearchText",edt.getText().toString());
editor.commit();
}
然后在其他两个活动中,从“共享首选项”中获取数据,如下所示:
SharedPreferences preferences = getSharedPreferences("sharedPrefs", 0);
String edtText = preferences.getString("SearchText","");
您还可以像这样在第二个活动中获取字符串:
Bundle extras = getIntent().getExtras();
if(extras != null) {
item = extras.getString("myitempassed");
}
我看不到您在代码中调用第三个活动(CompareWeight)的位置。
当您启动CompareWeight活动时,您可以像使用第二个活动一样使用意向附加值传递体重值。
如果此时没有权重值,则需要将其保存-@Avadhani Y对于使用SharedPreferences有很好的建议。 或者,您可以使用SQLite数据库来存储您的值。
在startActivity之前使用PutExtra。
这是因为在您的Second Activity
您只是在调用Intent而不传递任何值,
在第二活动中
替换意图
Intent a = new Intent(ViewProf1.this, FoodDiary.class);
startActivity(a);
有了这个
Intent a = new Intent(ViewProf1.this, FoodDiary.class);
a.putExtra("weight", weight1);
startActivity(a);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.