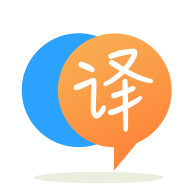
[英]How to format decimal in c# asp-format tag to 2 decimal points and without trailing zeros if it is an int?
[英]How to format a decimal without trailing zeros
我刚刚了解到小数会以某种方式记住存储数字需要多少尾随零。 换句话说:它记住分数的大小。
例如:
123M.ToString() ==> resuls in: 123
123.00M.ToString() ==> resuls in: 123.00
123.450M.ToString() ==> resuls in: 123.450
我正在寻找格式化字符串或其他技巧来摆脱那些“不需要的”尾随零,但保留有效数字。 所以:
123M.ToString() ==> resuls in: 123
123.00M.ToString() ==> resuls in: 123
123.450M.ToString() ==> resuls in: 123.45
删除新字符串末尾的零对我来说不是一个真正的选择,因为那时我必须找出字符串是否包含分数,如果是,还必须删除可选的 '.' 或 ',' 取决于文化等。
有几种方法可以做到这一点,但由于您无论如何都要转换为 String 对象,我想您可以尝试这样的事情:
myDecimalVariable.ToString("G29");
或者,使用上面的代码,假设123.00M
是您的小数:
123.00M.ToString("G29");
下面是对这个简洁示例如何工作的解释:
带数字的 G 格式意味着格式化那么多有效数字。 因为 29 是 Decimal 可以具有的最高有效数字,所以这将有效地截断尾随零而不舍入。
只需应用格式说明符零并删除尾随零:
string test = (1.23M * 100M).ToString("0");
//prints 123.
string test2 = 123.450M.ToString(".00");
//prints 123.45.
string test3 = 123.450M.ToString().Trim('0');
下面的方法 (🦍) 处理以下边缘情况:
private static string SlowButStrong(decimal v)
{
if( v % 1 == 0) return v.ToString(); // If no decimal digits, let's leave it alone
var withNoZeroes = v.ToString().TrimEnd('0');
return withNoZeroes.EndsWith('.') ? withNoZeroes + "00" : withNoZeroes;
}
Input 123M, expecting 123
✅ G29: 123
✅ 🦍: 123
✅ ⛵: 123
Input 123.00M, expecting 123.00
❌ G29: 123
✅ 🦍: 123.00
❌ ⛵: 123
Input 123.45M, expecting 123.45
✅ G29: 123.45
✅ 🦍: 123.45
✅ ⛵: 123.45
Input 123.450M, expecting 123.45
✅ G29: 123.45
✅ 🦍: 123.45
✅ ⛵: 123.45
Input 5.00000001M, expecting 5.00000001
✅ G29: 5.00000001
✅ 🦍: 5.00000001
✅ ⛵: 5.00000001
Input -0.00000000001M, expecting -0.00000000001
❌ G29: -1E-11
✅ 🦍: -0.00000000001
✅ ⛵: -0.00000000001
Input 10000000000000000000000M, expecting 10000000000000000000000
✅ G29: 10000000000000000000000
✅ 🦍: 10000000000000000000000
✅ ⛵: 10000000000000000000000
public static void Main(string[] args)
{
Test("123M", 123M, "123");
Test("123.00M", 123.00M, "123.00");
Test("123.45M", 123.45M, "123.45");
Test("123.450M", 123.450M, "123.45");
Test("5.00000001M", 5.00000001M, "5.00000001");
Test("-0.00000000001M", -0.00000000001M, "-0.00000000001");
Test("10000000000000000000000M", 10000000000000000000000M, "10000000000000000000000");
}
private static void Test(string vs, decimal v, string expected)
{
Console.OutputEncoding = System.Text.Encoding.UTF8;
Console.WriteLine($"Input {vs}, expecting {expected}");
Print("G29", v.ToString("G29"), expected);
Print("🦍", SlowButStrong(v), expected);
Print("⛵", LessSlowButStrong(v), expected);
Console.WriteLine();
}
private static void Print(string prefix, string formatted, string original)
{
var mark = formatted == original ? "✅" : "❌";
Console.WriteLine($"{mark} {prefix:10}: {formatted}");
}
private static string SlowButStrong(decimal v)
{
if( v % 1 == 0) return v.ToString(); // If no decimal digits, let's leave it alone
var withNoZeroes = v.ToString().TrimEnd('0');
return withNoZeroes.EndsWith('.') ? withNoZeroes + "00" : withNoZeroes;
}
private static string LessSlowButStrong(decimal v)
{
return v.ToString((v < 1) ? "" : "G29");
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.