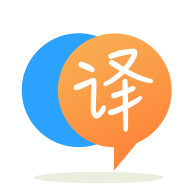
[英]How to use ContinueWith with condition on existing Task<TResult>
[英]return Task.ContinueWith<TResult>() without knowing TResult
如果我只能访问任务,该如何使用反射或任何其他方式创建和返回延续任务?
我需要一种让延续中的异常返回原始调用者的方法。 据我所知,这只能通过返回延续任务而不是原始任务来完成。 问题在于我不知道任务的结果类型,因此无法创建适当的延续任务。
编辑:我不能更改签名类型。 我有许多返回Task <TResult>对象的接口,不能期望客户端获得Task <Object>结果。 这些接口是WCF合同。 我想在完成“核心”逻辑方法后做一些额外的验证逻辑,并在需要时抛出异常。 此异常必须回传给客户端,但当前不会回传,因为我还没有返回继续任务。 另外我事先也不知道类型,因为我正在应用postharp方面并使用OnExit()覆盖,这使我可以访问一个我知道是Task的返回值,但是它可以是任意数量的Task对象TResult仅在运行时已知。
using System;
using System.Threading.Tasks;
namespace TaskContinueWith
{
internal class Program
{
private static void Main(string[] args)
{
try
{
Task<string> myTask = Interceptor();
myTask.Wait();
}
catch (Exception ex)
{
Console.WriteLine(ex);
}
Console.ReadLine();
}
private static Task<string> Interceptor()
{
Task<string> task = CoreLogic(); //Ignore
Task unknownReturnType = task; //This is what I have access to. A Task object which can be one of numerous Task<TResult> types only known at runtime.
Task continuation = unknownReturnType.ContinueWith(
t =>
{
if(someCondition)
{
throw new Exception("Error");
}
return t.Result; //This obviously does not work since we don't know the result.
});
return continuation;
}
private static async Task<string> CoreLogic()
{
return "test";
}
}
}
表达问题的另一种方法。
如何更改DoExtraValidation以使其适用于任何Task <TResult>返回类型?
using System;
using System.Threading.Tasks;
namespace TaskContinueWith
{
interface IServiceContract
{
Task<string> DoWork();
}
public class Servce : IServiceContract
{
public Task<string> DoWork()
{
var task = Task.FromResult("Hello");
return (Task<string>) DoExtraValidation(task);
}
private static Task DoExtraValidation(Task task)
{
Task returnTask = null;
if (task.GetType() == typeof(Task<string>))
{
var knownType = task as Task<string>;
returnTask = task.ContinueWith(
t =>
{
if(new Random().Next(100) > 50)
{
throw new Exception("Error");
}
return knownType.Result;
});
}
return returnTask;
}
}
internal class Program
{
private static void Main(string[] args)
{
try
{
IServiceContract myService = new Servce();
Task<string> myTask = myService.DoWork();
myTask.Wait();
}
catch (Exception ex)
{
Console.WriteLine(ex);
}
Console.ReadLine();
}
}
}
听起来像是dynamic
案例。 我将尝试使用尽可能少的dynamic
。 首先,我们定义一个强类型的助手:
static Task<TResult> SetContinuation<TResult>(Task<TResult> task)
{
return task.ContinueWith(
t =>
{
if(someCondition)
{
throw new Exception("Error");
}
return t.Result;
});
}
该函数显然有效,但是需要知道TResult
。 dynamic
可以填写:
Task continuation = SetContinuation((dynamic)unknownReturnType);
我刚刚测试了绑定在运行时有效。 另外,您也可以使用反射来调用帮助程序(使用MethodInfo.MakeGenericMethod
等)。
您可以使用泛型(拦截器在这里是扩展方法):
public static class ProjectExtensions
{
public static Task<T> Interceptor<T>(this Task<T> task)
{
Task<T> continuation = task.ContinueWith<T>(t =>
{
if(someCondition)
{
throw new Exception("Error");
}
return t.Result;
});
return continuation;
}
}
这样,呼叫可以是任何类型:
var t1 = new Task<string>(() => /* return string */).Interceptor();
var t2 = new Task<int>(() => /* return int */).Interceptor();
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.