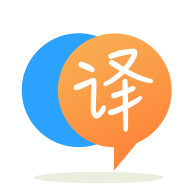
[英]Read in a paragraph witht getchar() and print it with a loop and putchar()
[英]print multiple lines by getchar and putchar
我是初学者,学习C编程语言并使用Microsoft Visual C ++编写和测试代码。
下面的C语言程序(第1.5.1节)通过putchar()和getchar()将其输入复制到其输出:
#include <stdio.h>
int main(void)
{ int c;
while ((c = getchar()) != EOF)
putchar(c);
return 0;}
每次按ENTER键时,程序打印由键盘输入的字符。因此,我只能在打印前输入一行。 在打印之前,我找不到通过键盘输入多行文本的方法。
有什么方法以及如何让这个程序从键盘输入和输出多行文字?
对不起,如果这是一个基本的,无知的问题。
提前感谢您的关注和感谢。
一些聪明的指针算法用来做你想要的:
#include <stdio.h> /* this is for printf and fgets */
#include <string.h> /* this is for strcpy and strlen */
#define SIZE 255 /* using something like SIZE is nicer than just magic numbers */
int main()
{
char input_buffer[SIZE]; /* this will take user input */
char output_buffer[SIZE * 4]; /* as we will be storing multiple lines let's make this big enough */
int offset = 0; /* we will be storing the input at different offsets in the output buffer */
/* NULL is for error checking, if user enters only a new line, input is terminated */
while(fgets(input_buffer, SIZE, stdin) != NULL && input_buffer[0] != '\n')
{
strcpy(output_buffer + offset, input_buffer); /* copy input at offset into output */
offset += strlen(input_buffer); /* advance the offset by the length of the string */
}
printf("%s", output_buffer); /* print our input */
return 0;
}
这就是我使用它的方式:
$ ./a.out
adas
asdasdsa
adsa
adas
asdasdsa
adsa
一切都是鹦鹉学舌:)
我用过fgets , strcpy和strlen 。 请查看它们,因为它们是非常有用的功能(并且fgets
是获取用户输入的推荐方式)。
在您输入“+”并按下输入后,输入您输入的所有数据,然后打印。 您可以将数组的大小增加到100以上
#include <stdio.h>
int main(void)
{ int c='\0';
char ch[100];
int i=0;
while (c != EOF){
c = getchar();
ch[i]=c;
i++;
if(c=='+'){
for(int j=0;j<i;j++){
printf("%c",ch[j]);
}
}
}
return 0;
}
你可以在'+'字符或任何你想要表示打印操作的字符上放置一个条件,这样这个字符就不会存储在数组中(我现在没有在'+'上添加任何这样的条件)
使用setbuffer()
使stdout
完全缓冲(最大为缓冲区的大小)。
#include <stdio.h>
#define BUFSIZE 8192
#define LINES 3
char buf[BUFSIZE];
int main(void)
{ int c;
int lines = 0;
setbuffer(stdout, buf, sizeof(buf));
while ((c = getchar()) != EOF) {
lines += (c == '\n');
putchar(c);
if (lines == LINES) {
fflush(stdout);
lines = 0;
}}
return 0;}
您可以使用GetKeyState函数检查按下Enter键时是否按住SHIFT键吗? 那就是你可以使用SHIFT / ENTER输入多行,并使用普通的ENTER键发送整个行。 就像是:
#include <stdio.h>
int main(void)
{ int c;
while (true){
c = getChar();
if (c == EOF && GetKeyState(VK_LSHIFT) {
putchar("\n");
continue;
else if(c == EOF) break;
else {
putchar(c);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.