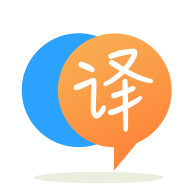
[英]JFrame + JOGL only displays content when resized or minimised + maximised
[英]GUI not displaying content till resized or minimised
好的...我的问题很简单,因此我怀疑是否需要添加任何代码,但是如果需要的话,我会添加。
每当我创建一个GUI框架并向其中添加几个面板并运行我的应用程序时,在我调整窗口大小或在工具栏上最小化窗口或将其最小化然后还原它之前,内容都不会显示。 这可能是什么原因,我该如何解决?
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import java.awt.GridLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
public final class Calculator extends JFrame
{
//initialise various variables for use within the program
//BUTTONS
private final JButton additionButton = new JButton("+");
private final JButton subtractionButton = new JButton("-");
private final JButton divisionButton = new JButton("/");
private final JButton multiplicationButton = new JButton("*");
//PANELS
private JPanel operatorPanel;
private JPanel operandPanel;
//LABELS
private JLabel operationLabel;
//constructor to initialise the frame and add components into it
public Calculator()
{
super("Clancy's Calculator");
setLayout(new BorderLayout(5, 10));
setSize(370, 200);
setResizable(false);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setVisible(true);
//create a message label to display the operation that has just taken place
operationLabel = new JLabel("YOU HAVE PERFORMED SOME OPERATION",SwingConstants.CENTER);
add(getOperatorPanel(), BorderLayout.NORTH);
add(getOperandPanel(), BorderLayout.CENTER);
add(operationLabel, BorderLayout.SOUTH);
}
//setter method for the operator panel
public void setOperatorPanel()
{
operatorPanel = new JPanel();
operatorPanel.setLayout(new FlowLayout());
operatorPanel.add(additionButton);
operatorPanel.add(subtractionButton);
operatorPanel.add(multiplicationButton);
operatorPanel.add(divisionButton);
}
//getter method for the operator panel
public JPanel getOperatorPanel()
{
setOperatorPanel();
return operatorPanel;
}
//setter method for operands panel
public void setOperandPanel()
{
operandPanel = new JPanel();
operandPanel.setLayout(new GridLayout(3, 2, 5, 5));
//LABELS
JLabel operandOneLabel = new JLabel("Enter the first Operand: ");
JLabel operandTwoLabel = new JLabel("Enter the second Operand: ");
JLabel answerLabel = new JLabel("ANSWER: ");
//TEXT FIELDS
JTextField operandOneText = new JTextField(); //retrieves one operand
JTextField operandTwoText = new JTextField(); //retrieves another operand
JTextField answerText = new JTextField(); //displays answer
answerText.setEditable(false); //ensure the answer field is not editable
operandPanel.add(operandOneLabel);
operandPanel.add(operandOneText);
operandPanel.add(operandTwoLabel);
operandPanel.add(operandTwoText);
operandPanel.add(answerLabel);
operandPanel.add(answerText);
}
//getter method for operand panel
public JPanel getOperandPanel()
{
setOperandPanel();
return operandPanel;
}
/** main method */
public static void main(String[] args)
{
new Calculator();
}
}
我注意到您正在以编程方式设置新的布局管理器。 每当添加,删除或更改Java gui时,都需要调用invalidate()
或revalidate()
来使Java重新创建GUI。 查看是否在setVisible(true)
之前调用invalidate()
setVisible(true)
解决问题
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.