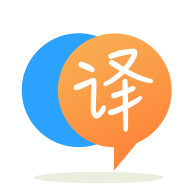
[英]How can I write a definition of function declared in template class returning pointer to struct object?
[英]How to write a template function that can handle object or pointer function calls ?
我希望能够编写一个可以在容器的所有元素上调用函数调用的模板函数。 我们可以假定函数名称始终相同。 但是,还不知道容器是保存对象还是指针。 即,我是否应该取消引用。
template< typename TContainer >
void ProcessKeyedContainer( TContainer &keyedContainer )
{
for ( auto it = keyedContainer.begin(); it != keyedContainer.end(); ++it )
{
// do some random stuff here.
// ...
auto value = it->second;
value.Process(); // or value->Process() if the container has pointers
}
}
...
std::map< int, CMyObject > containerOfObjects;
containerOfObjects[0] = CMyObject();
std::map< int, CMyObject* > containerOfPointers;
containerOfPointers[0] = new CMyObject();
// I would like both calls to look near identical
ProcessKeyedContainer( containerOfObjects );
ProcessKeyedContainer( containerOfPointers );
有没有一种整洁的方法可以在ProcessKeyedContainer内部进行Process调用,而又不给调用方造成负担(即,调用方不必知道以一种方式将其用于指针,而将另一种方式用于对象),并且没有不必重复太多代码?
重载的函数模板是救星:
template<typename T>
void invoke(T * obj) //when object is pointer
{
obj->Process();
}
template<typename T>
void invoke(T & obj) //when object is non-pointer
{
obj.Process();
}
然后将其用作:
auto value = it->second;
invoke(value); //correct invoke() will be selected by the compiler!
但这还不够好,因为您可能还想利用您编写的其余函数中的value
来做其他事情。 因此,如果您遵循上述方法,将会有代码重复,因为两个invoke()
都将具有几乎相似的代码。
因此,这是一个改进:不用使用invoke()
,而是将指针变成引用,以便可以在函数中统一使用它。
template<typename T>
T& ensure_ref(T * obj) //when object is pointer
{
return *obj; //return the dereferenced object
}
template<typename T>
T& ensure_ref(T & obj) //when object is non-pointer
{
return obj; //simply return it
}
并将其用作:
auto & value = ensure_ref(it->second); //call ensure_ref to ensure reference!
value.Process(); //value is gauranteed to be NOT pointer!
//you might want to do this also!
value.xyz = abc;
希望有帮助!
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.