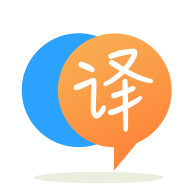
[英]How can I get selected value from mvc dropdown list as action link id in c#
[英]Bind Enum with dropdown and set selected value on get action in MVC C#
我有一个叫做CityType
的Enum
public enum CityType
{
[Description("Select City")]
Select = 0,
[Description("A")]
NewDelhi = 1,
[Description("B")]
Mumbai = 2,
[Description("C")]
Bangalore = 3,
[Description("D")]
Buxar = 4,
[Description("E")]
Jabalpur = 5
}
从枚举生成列表
IList<SelectListItem> list = Enum.GetValues(typeof(CityType)).Cast<CityType>().Select(x => new SelectListItem(){
Text = EnumHelper.GetDescription(x),
Value = ((int)x).ToString()
}).ToList();
int city=0;
if (userModel.HomeCity != null) city= (int)userModel.HomeCity;
ViewData["HomeCity"] = new SelectList(list, "Value", "Text", city);
绑定.cshtml
@Html.DropDownList("HomeCity",null,new { @style = "width:155px;", @class = "form-control" })
EnumHelper GetDescription类获取Enum的描述
我在博客上写了这个主题,我创建了一个EnumHelper
来帮助完成这项常见任务。
http://jnye.co/Posts/4/creating-a-dropdown-list-from-an-enum-in-mvc-and-c%23
在你的控制器中:
//If you don't have an enum value use the type
ViewBag.DropDownList = EnumHelper.SelectListFor<MyEnum>();
//If you do have an enum value use the value (the value will be marked as selected)
ViewBag.DropDownList = EnumHelper.SelectListFor(MyEnum.MyEnumValue);
在您的视图中:
@Html.DropDownList("DropDownList")
@* OR *@
@Html.DropDownListFor(m => m.Property, ViewBag.DropDownList as SelectList, null)
助手类:
public static class EnumHelper
{
// Get the value of the description attribute if the
// enum has one, otherwise use the value.
public static string GetDescription<TEnum>(this TEnum value)
{
var fi = value.GetType().GetField(value.ToString());
if (fi != null)
{
var attributes = (DescriptionAttribute[])fi.GetCustomAttributes(typeof(DescriptionAttribute), false);
if (attributes.Length > 0)
{
return attributes[0].Description;
}
}
return value.ToString();
}
/// <summary>
/// Build a select list for an enum
/// </summary>
public static SelectList SelectListFor<T>() where T : struct
{
Type t = typeof(T);
return !t.IsEnum ? null
: new SelectList(BuildSelectListItems(t), "Value", "Text");
}
/// <summary>
/// Build a select list for an enum with a particular value selected
/// </summary>
public static SelectList SelectListFor<T>(T selected) where T : struct
{
Type t = typeof(T);
return !t.IsEnum ? null
: new SelectList(BuildSelectListItems(t), "Text", "Value", selected.ToString());
}
private static IEnumerable<SelectListItem> BuildSelectListItems(Type t)
{
return Enum.GetValues(t)
.Cast<Enum>()
.Select(e => new SelectListItem { Value = e.ToString(), Text = e.GetDescription() });
}
}
这是我在下拉列表中用于枚举的代码。 然后只需使用@ Html.DropDown / For(); 并将此SelectList作为参数放入。
public static SelectList ToSelectList(this Type enumType, string selectedValue)
{
var items = new List<SelectListItem>();
var selectedValueId = 0;
foreach (var item in Enum.GetValues(enumType))
{
FieldInfo fi = enumType.GetField(item.ToString());
DescriptionAttribute[] attributes = (DescriptionAttribute[])fi.GetCustomAttributes(typeof(DescriptionAttribute), false);
var title = "";
if (attributes != null && attributes.Length > 0)
{
title = attributes[0].Description;
}
else
{
title = item.ToString();
}
var listItem = new SelectListItem
{
Value = ((int)item).ToString(),
Text = title,
Selected = selectedValue == ((int)item).ToString(),
};
items.Add(listItem);
}
return new SelectList(items, "Value", "Text", selectedValueId);
}
你也可以这样扩展DropDownFor:
public static MvcHtmlString EnumDropdownListFor<TModel, TProperty>(this HtmlHelper<TModel> htmlHelper, Expression<Func<TModel, TProperty>> expression, Type enumType, object htmlAttributes = null)
{
ModelMetadata metadata = ModelMetadata.FromLambdaExpression(expression, htmlHelper.ViewData);
SelectList selectList = enumType.ToSelectList(metadata.Model.ToString());
return htmlHelper.DropDownListFor(expression, selectList, htmlAttributes);
}
用法然后如下所示:
@Html.EnumDropdownListFor(model => model.Property, typeof(SpecificEnum))
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.