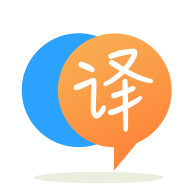
[英]Why ConnectionString fails for SqlConnection using MSN example in C#?
[英]Why is a C# EventHandler Equal to an Event in MSN example?
以下是来自MSN网站的示例。 这是一个很好的例子,我只是不明白这行是什么:
EventHandler<CustomEventArgs> handler = RaiseCustomEvent;
是在做?
RaiseCustomEvent
是否不是基于程序顶部定义的事件?
为什么将事件等同于EventHandler? 这是两种不同的类型。
RaiseCustomEvent
在哪里初始化? 如果未初始化,该如何复制?为什么要将未初始化的内容复制到其他内容?
handler
变量有什么用? 那是事件还是事件处理程序?
我很困惑,并且正在努力使此事件/事件处理程序/委托问题得到理解。
这是来自MSN的示例代码
namespace DotNetEvents
{
using System;
using System.Collections.Generic;
// Define a class to hold custom event info
public class CustomEventArgs : EventArgs
{
public CustomEventArgs(string s)
{
message = s;
}
private string message;
public string Message
{
get { return message; }
set { message = value; }
}
}
// Class that publishes an event
class Publisher
{
// Declare the event using EventHandler<T>
public event EventHandler<CustomEventArgs> RaiseCustomEvent;
public void DoSomething()
{
// Write some code that does something useful here
// then raise the event. You can also raise an event
// before you execute a block of code.
OnRaiseCustomEvent(new CustomEventArgs("Did something"));
}
// Wrap event invocations inside a protected virtual method
// to allow derived classes to override the event invocation behavior
protected virtual void OnRaiseCustomEvent(CustomEventArgs e)
{
// Make a temporary copy of the event to avoid possibility of
// a race condition if the last subscriber unsubscribes
// immediately after the null check and before the event is raised.
EventHandler<CustomEventArgs> handler = RaiseCustomEvent;
// Event will be null if there are no subscribers
if (handler != null)
{
// Format the string to send inside the CustomEventArgs parameter
e.Message += String.Format(" at {0}", DateTime.Now.ToString());
// Use the () operator to raise the event.
handler(this, e);
}
}
}
//Class that subscribes to an event
class Subscriber
{
private string id;
public Subscriber(string ID, Publisher pub)
{
id = ID;
// Subscribe to the event using C# 2.0 syntax
pub.RaiseCustomEvent += HandleCustomEvent;
}
// Define what actions to take when the event is raised.
void HandleCustomEvent(object sender, CustomEventArgs e)
{
Console.WriteLine(id + " received this message: {0}", e.Message);
}
}
class Program
{
static void Main(string[] args)
{
Publisher pub = new Publisher();
Subscriber sub1 = new Subscriber("sub1", pub);
Subscriber sub2 = new Subscriber("sub2", pub);
// Call the method that raises the event.
pub.DoSomething();
// Keep the console window open
Console.WriteLine("Press Enter to close this window.");
Console.ReadLine();
}
}
}
对于委托类型而言,事件就是对其他类型而言的属性。
如果您具有这样的属性:
public string Name {get;set;}
显然,您可以执行以下操作:
string name = Name;
该属性具有由属性访问/修改的基础字符串值。
同样,一个事件在后台具有一个委托,并且该委托具有事件声明中定义的类型。 它是一个事件,仅定义如何从该基础委托中添加/删除该事件的处理程序。
在声明类型内(这是关键点;您不能在外部执行此操作),可以访问该基础委托以进行调用。 这就是执行您看到的代码的原因 。 他们正在访问基础委托,以便他们可以验证其中是否包含一些处理程序,如果有,则调用它们。
因此,要明确回答问题:
RaiseCustomEvent
是否不是基于程序顶部定义的事件?
RaiseCustomEvent
是事件包装的基础委托的类型。
为什么将事件等同于EventHandler? 这是两种不同的类型。
这不是严格意义上的平等。 它从事件内部拉出了底层委托。
RaiseCustomEvent
在哪里初始化? 如果未初始化,该如何复制?为什么要将未初始化的内容复制到其他内容?
在这种情况下,它将使用框架将提供的自动添加/删除实现,而不是手动定义它们。 如果当前为空,则自动定义的添加处理程序将初始化基础委托。 如果事件声明定义了自定义add
处理程序,则需要处理这种情况。
handler
变量有什么用? 那是事件还是事件处理程序?
它是一个代表所有事件处理程序组合的委托。 它的定义中将包含组成该委托的所有单个方法的调用列表。 因此,它不是单个事件处理程序,而是所有事件处理程序的集合。 由于已退出事件,因此不再严格代表该事件; 它是过去某个时刻事件的副本。 您不能使用退出事件的委托来更改事件(即添加新的处理程序)。
我将尝试您提出的四个问题:
1)RaiseCustomEvent是否不是基于程序顶部定义的事件?
CustomEventArgs
类为我们要声明的事件保存一些数据(参数)。 在EventHandler<CustomEventArgs>
类型中用作类型参数。 最后一种类型是委托类型,这意味着它表示一个或多个具有相同签名和返回类型的方法。 (零方法将作为null
的值成为null
引用。)
事件RaiseCustomEvent
的类型是该委托类型EventHandler<CustomEventArgs>
。
2)为什么一个事件等于一个事件处理程序? 这是两种不同的类型
一个事件由一对特殊的方法,访问器,一个add
访问器和一个remove
访问器组成。 两者都有一个相同类型的参数,称为事件的类型。 该类型必须是委托类型。 在这种情况下,该类型为EventHandler<CustomEventArgs>
。
在此示例中,事件是所谓的类似领域的事件。 它将生成相同类型的后备字段 ,即委托类型EventHandler<CustomEventArgs>
。 该字段与事件本身具有相同的名称!
当他们这样做时:
// Make a temporary copy of the event to avoid possibility of
// a race condition if the last subscriber unsubscribes
// immediately after the null check and before the event is raised.
EventHandler<CustomEventArgs> handler = RaiseCustomEvent;
他们将支持字段的当前值复制到局部变量handler
。 注释描述了原因。 他们希望在调用委托之前检查null
。
3)RaiseCustomEvent在哪里初始化? 如果未初始化,我们该如何复制,或者为什么要将未初始化的内容复制到其他对象。
人们通常使用事件的add
访问器来初始化它。 这是通过特殊的C#语法+=
。 这称为订阅事件。 请参阅Subscriber
类。
实际上是pub.RaiseCustomEvent += HandleCustomEvent;
转换为pub.add_RaiseCustomEvent(HandleCustomEvent);
,因此它是对add
访问器的调用。 add
访问器由编译器生成(在类似字段的事件中),并且它初始化后备字段。
4)我不知道什么是处理程序变量? 是事件还是事件处理程序?
是代表。 这不是一个事件。 它是某个时刻的类字段事件的后备字段的副本。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.