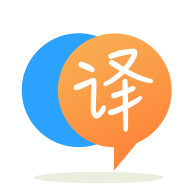
[英]Why does my algorthim for insertion sort return different values , when I use the same value in condition statement?
[英]Why my insertion sort function does not change the array that I passed from int main?
简短版本(使用硬连线输入)
#include <iostream>
#include <string>
#include <vector>
using namespace std;
void insertionSort(string theArray[], int n)
{
//unsorted = first index of the unsorted region,
//loc = index of insertion in the sorted region,
//nextItem = next item in the unsorted region,
//Initially, sorted region is theArray[0]
// unsorted region is theArray [1..n-1].
//In general, sorted region is theArray[0..unsorted-1],
// unsorted region theArray[unsorted.. n-1]
for(int unsorted = 1; unsorted< n; unsorted++)
{
//At this point, theArray[0..unsorted-1] is sorted.
//Find the right position (loc) in theArray[0..unsorted]
//for theArray[unsorted], which is the first entry in the
//unsorted region; shift, if necessary, to make room
string nextItem = theArray[unsorted];
int loc = unsorted;
while( (loc > 0) && (theArray[loc-1] > nextItem) )
{
//shift theArray [loc -1] to the right
theArray[loc] = theArray[loc-1];
}
// At this point, theArray[loc] is where nexItem belongs
theArray[loc] = nextItem; // Insert nextItem into sorted region
loc--;
}// end for
}
int main()
{
vector<string> token;
int countToken;
string input= "I,love,doing,nothing,at,all";
int count =0;
for(int i=0; i< input.length(); i++)
{
if(input[i] == ',')
count++;
}
countToken = count +1;
for(int i=0; i< countToken; i++)
{
int x= input.find(',');
token.push_back(input.substr(0,x));
input = input.substr(x+1);
}
cout << endl << "Current String: ";
for(int i =0; i< countToken; i++)
{
cout << token[i] <<" " ;
}
cout << endl;
string theArray[countToken];
for(int i =0; i< countToken; i++)
{
theArray[i] = token[i];
}
insertionSort(theArray, countToken);
cout << "SORTED: " ;
for(int i =0; i< countToken; i++)
{
cout << theArray[i] << " ";
}
return 0;
}// main
完整版本
#include <iostream>
#include <string>
#include <vector>
using namespace std;
void insertionSort(string theArray[], int n)
{
//unsorted = first index of the unsorted region,
//loc = index of insertion in the sorted region,
//nextItem = next item in the unsorted region,
//Initially, sorted region is theArray[0]
// unsorted region is theArray [1..n-1].
//In general, sorted region is theArray[0..unsorted-1],
// unsorted region theArray[unsorted.. n-1]
for(int unsorted = 1; unsorted< n; unsorted++)
{
//At this point, theArray[0..unsorted-1] is sorted.
//Find the right position (loc) in theArray[0..unsorted]
//for theArray[unsorted], which is the first entry in the
//unsorted region; shift, if necessary, to make room
string nextItem = theArray[unsorted];
int loc = unsorted;
while( (loc > 0) && (theArray[loc-1] > nextItem) )
{
//shift theArray [loc -1] to the right
theArray[loc] = theArray[loc-1];
}
// At this point, theArray[loc] is where nexItem belongs
theArray[loc] = nextItem; // Insert nextItem into sorted region
loc--;
}// end for
}
int main()
{
vector<string> token;
int countToken;
while(1)
{
int answer;
cout << "MENU: " << endl;
cout << "1. Input String " << endl;
cout << "2. Sort " << endl;
cout << "3. Quit " << endl;
cout << endl;
cout << "Response: ";
cin >> answer;
cin.ignore(1000, 10);
if( answer == 1) // case 1. Input String
{
string input;
cout << endl << "Default delimiter is ','"<< endl << "Enter String: " ;
getline(cin, input);
// find delimiter
int count =0;
for(int i=0; i< input.length(); i++)
{
if(input[i] == ',')
count++;
}
//cout << "Count: "<< count <<endl;
//clear previous vector
token.clear();
countToken = count +1;
for(int i=0; i< countToken; i++)
{
int x= input.find(',');
token.push_back(input.substr(0,x));
input = input.substr(x+1);
}
//cout << "countToken: " << countToken << endl;
cout << endl << "Current String: ";
for(int i =0; i< countToken; i++)
{
cout << token[i] <<" " ;
}
cout << endl;
}
else if(answer == 2) // case 2. Sort
{
string theArray[countToken];
for(int i =0; i< countToken; i++)
{
theArray[i] = token[i];
}
//cout << "COUNTTOKEN: "<< countToken;
insertionSort(theArray, countToken);
cout << "SORTED: " ;
for(int i =0; i< countToken; i++)
{
cout << theArray[i] << " ";
}
}
else if(answer == 3)
{
break;
}
else
{
cout << endl << "Invalid input !" << endl << endl;
}
cout << endl;
}// while
return 0;
}// main
嗨,我的程序的简要说明。 我要求用户输入一组带有','的字符串作为分隔符,以在每次找到','时将其切断,并将其推入向量,然后将其传递给数组,然后将数组传递给插入排序功能。 (nb:如果字符串中包含任何空格,程序将无法正确剪切)
但是,我的插入排序功能似乎并没有更改我正确传递的数组。 当我调用该函数时,我的程序甚至崩溃了。 我读了一篇文章,并说默认情况下数组是通过引用传递的。因此,我认为函数的参数中的&符号不必更改main中的数组。
我希望有人能弄清楚我的程序中缺少什么。
谢谢
主要问题是您没有在循环中更改loc
的状态。
while( (loc > 0) && (theArray[loc-1] > nextItem) )
{
//shift theArray [loc -1] to the right
theArray[loc] = theArray[loc-1];
//add this
loc--; // move to the left so that the shift can go on
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.