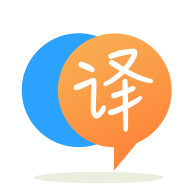
[英]javascript array - what is the quickest most efficient way to create an array with a starting index and a default value
[英]What is the quickest and most efficient way of testing one array of items against another and then sorting the matches in javascript?
我最近创建了一个脚本,该脚本可以查看一个电子表格并创建所需的项目列表。 然后,它将在另一个电子表格中查找那些项目,并创建匹配项的对象数组。 然后,对象按其name属性排序。 最终,它们被输出到另一张纸上,但这与这里无关。
在JS中执行此操作的最佳方法是什么? 有没有比我用过的方法更有效的方法(我敢肯定有)。
//get basic information about the spreadsheet
var sheets = SpreadsheetApp.getActiveSpreadsheet().getSheets();
var rows = new Array();
var numRows = new Array();
for (var i = 0; i < sheets.length; i++) {
rows[i] = sheets[i].getDataRange();
numRows[i] = rows[i].getNumRows();
}
//Create an object for each company with suppressed and total statements variables
//object will have properties name, supressed integer, total integer, and a percentage calculated through a percentage() method
function companySupressionStat(name, supressed, total) {
this.name = name;
this.supressed = supressed;
this.total = total;
}
var objectNames = new Array();
// find the column with the supression data
function findSupression() {
var supressedColumn;
// check every column on the 3rd row for the word supressed column
for (var l = 1; l < sheets[1].getLastColumn(); l++) {
if (sheets[1].getRange(3, l).getValue() == "Supr.ed Stmts") {
supressedColumn = l;
break;
}
}
return supressedColumn;
}
//find company name column
function findCompany() {
var companyColumn;
for (var l = 1; l < sheets[1].getLastColumn(); l++) {
if (sheets[1].getRange(1, l).getValue() == "SLA Legend") {
companyColumn = l;
break;
}
}
return companyColumn;
}
// check if we have missing value, then identify them in an array of strings called listErrors
function listErrorChecker(listLength, objectLength, objectNames, list) {
var listErrors = new Array(); // create a variable to keep track of our missing company's
if (listLength > objectLength) { //if our list is longer than our array of objects
for (var l = 0; l < list.length; l++) {
Logger.log(objectNames.indexOf(list[l]));
if (objectNames.indexOf(list[l]) == -1) {
listErrors.push("The company " + list[l] + " is missing from our output! Please check the spelling and case of " + list[l].toUpperCase() + " and make sure it is the same as the report we are looking in.");
}
}
return listErrors;
}
else {
listErrors = "no errors";
return listErrors;
}
}
//Create an array list of all the company's we want to track
var list = new Array();
for (var k = 1; k <= numRows[0]; k++) {
list.push(sheets[0].getRange(k, 1).getValue().toLowerCase()); //assumes that our list is in our first spreadsheet, in the first column
}
//Go down the spreadsheet on the column w/ the word "suppression". start creating new objects with the the correct properties if the company name is on our list of company names.
var arrayOfObjects = new Array();
var companyColumn = findCompany();
var supressedColumn = findSupression();
var lastRow = sheets[1].getLastRow();
for (var m = 1; m <= lastRow; m++) {
if (sheets[1].getRange(m, companyColumn).getValue() !== "" && list.indexOf(sheets[1].getRange(m, companyColumn).getValue().toLowerCase()) !== -1) { //if the company name is not blank and if the company name is in our list, create a new instance of the compression stat object
arrayOfObjects.push(new companySupressionStat(
sheets[1].getRange(m, companyColumn).getValue(),
sheets[1].getRange(m, supressedColumn).getValue(),
sheets[1].getRange(m, supressedColumn + 2).getValue()));
objectNames.push(sheets[1].getRange(m, companyColumn).getValue().toLowerCase()); //keep track of the object's names in a simple arrray of strings for error checking later
}
}
// sort our array of objects by name of company alphabetically
function sortOn(property) {
return function(a, b) {
if (a[property] < b[property]) {
return -1;
}
else if (a[property] > b[property]) {
return 1;
}
else {
return 0;
}
};
}
arrayOfObjects.sort(sortOn("name")); // call the sorting function
我肯定会将list和objectNames列表更改为对象,然后使用对象属性(如C#中的HashSet)。
例如,我将列表更改为:
var list = {};
for (var k = 1; k <= numRows[0]; k++) {
list[sheets[0].getRange(k, 1).getValue().toLowerCase()]=1;
}
....
....
....
for (var m = 1; m <= lastRow; m++) {
if (sheets[1].getRange(m, companyColumn).getValue() !== "" && list[sheets[1].getRange(m, companyColumn).getValue().toLowerCase()]) {
通常,搜索数组比查找对象属性要慢。
在此处查看: 阵列性能
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.