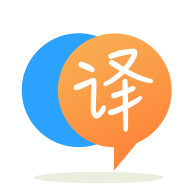
[英]SOLVED How to write a method to sort numbers from an array from low to high
[英]How to sort an array when it is filled with numbers from a get method
Arrays.sort()给出以下错误:
FiveDice.java:19: error: no suitable method found for sort(int)
Arrays.sort(compNums);
如果我从for循环中取出任何东西,它会认为您只有1个数字或给出错误。 还有哪些其他排序选项可用?
import java.util.*;
public class FiveDice {
public static void main(String[] args) {
int x;
int compNums = 0;
int playerNums;
Die[] comp = new Die[5];
Die[] player = new Die[5];
System.out.print("The highest combination wins! \n5 of a kind, 4 of a kind, 3 of a kind, or a pair\n");
//computer
System.out.print("Computer rolled: ");
for(x = 0; x < comp.length; ++x) {
comp[x] = new Die();
compNums = comp[x].getRoll();
//Arrays.sort(compNums); <--does not work
System.out.print(compNums + " ");
}
//player
System.out.print("\nYou rolled: \t ");
for(x = 0; x < player.length; ++x) {
player[x] = new Die();
playerNums = player[x].getRoll();
System.out.print(playerNums + " ");
}
}
}
死班
public class Die {
int roll;
final int HIGHEST_DIE_VALUE = 6;
final int LOWEST_DIE_VALUE = 1;
public Die()
{ }
public int getRoll() {
roll = ((int)(Math.random() * 100) % HIGHEST_DIE_VALUE + LOWEST_DIE_VALUE);
return roll; }
public void setRoll()
{ this.roll = roll; }
}
最简单的方法是实现与Die类相当,不在getter方法中在Die的构造函数中设置roll的值,即可解决您的问题。
public class Die implements Comparable<Die> {
private int roll;
final int HIGHEST_DIE_VALUE = 6;
final int LOWEST_DIE_VALUE = 1;
public Die() {
roll = ((int)(Math.random() * 100) % HIGHEST_DIE_VALUE + LOWEST_DIE_VALUE);
}
public int getRoll() {
return roll;
}
public void setRoll(int roll) {
this.roll = roll;
}
public int compareTo(Die d) {
return new Integer(d.getRoll()).compareTo(new Integer(this.getRoll()));
}
}
现在, Arrays.sort(Die[])
将对Die的数组进行排序。
您不能对compNums
执行sort()
,因为它是单个值。 您将其声明为int
值,而不是整数数组。
相反,您应该使compNums
一个数组,并用Die
roll值填充它,然后对结果数组执行排序操作。 我相信以下将实现您的追求。
int[] compNums = new int[5];
...
for(x = 0; x < comp.length; ++x) {
comp[x] = new Die();
compNums[x] = comp[x].getRoll();
}
Arrays.sort(compNums);
// print results
您不必使用2个数组进行排序。 如果实现Comparable<T>
接口,则可以通过Java Collections API对类进行排序。 在您的情况下, Die
类可以实现Comparable<T>
并为Java框架提供一种比较骰子值的方法。
看一下Java API: http : //docs.oracle.com/javase/7/docs/api/java/lang/Comparable.html
在您的情况下:
public class Die implements Comparable<Die>{
int roll;
final int HIGHEST_DIE_VALUE = 6;
final int LOWEST_DIE_VALUE = 1;
public Die() { }
public int computeRoll() {
roll = ((int)(Math.random() * 100) % HIGHEST_DIE_VALUE + LOWEST_DIE_VALUE);
return roll;
}
// I also changed this to store the value
public int getRoll() {
return roll;
}
public void setRoll() { this.roll = roll; }
// This is the method you have to implement
public int compareTo(Die d) {
if(getRoll() < d.getRoll()) return -1;
if(getRoll() > d.getRoll()) return +1;
if(getRoll() == d.getRoll()) return 0;
}
}
只要您拥有骰子Collection
(如ArrayList或LinkedList),就可以对集合本身进行排序。 以下是示例代码。
ArrayList<Die> myCollection = new ArrayList<Die>();
myCollection.add(die1);
// more array population code
// ...
Collections.sort(myCollection);
您必须将数组传递给Array.Sort(arr)
而不是参数,您必须执行类似Array.Sort(int[]compNums);
如果您使用的是像comp[]compNums
这样的匿名类型,则必须这样
java.util.Arrays.sort(comp[]compNums, new java.util.Comparator<Object[]>() {
public int compare(Object a[], Object b[]) {
if(something)
return 1;
return 0;
}
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.