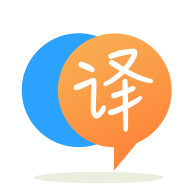
[英]Java Replace a substring after a certain number of repeats of that substring
[英]How do I replace a substring that occurs after a certain number of spaces in a string?
我正在以这种格式从文件中提取数据:
`1111 1.0 2222 53.5 3333 0.3 4444 541.1 5555 0.3'
现在假设我想用32.2
代替0.3
。 我如何最好地用Java实现它?
我想到了使用str.replaceAll(str.substring(beginIndex, endIndex), replacement)
。 但是,它需要一个开始索引和结束索引,我无法轻易提供。
Java中最好的方法是什么?
编辑:可能有多个0.3的实例。 我只希望更改确定的编号后出现的编号。 的空间。 例如,这发生在5个空格之后。
以下内容简洁明了且可动态适应。 我实例化了变量以提供示例,但关键部分是代码的第一行和最后几行:
String regex = "(?<=\\s{%s})%s(?=\\s{%s})";
String numSpaces = "1";
String number = "0.3";
String replacement = "32.2";
String yourString = "1111 1.0 2222 53.5 3333 0.3 4444 541.1";
yourString = yourString.replaceAll(String.format(regex, numSpaces, number, numSpaces),
replacement);
System.out.println(yourString);
//prints 1111 1.0 2222 53.5 3333 32.2 4444 541.1
在此示例中, "(?<=\\\\s{%s})%s(?=\\\\s{%s})"
将变为: (?<=\\\\s{1})0.3(?=\\\\s{1})
这意味着"0.3"
前后带有一个空格标记,它利用前后前后的正面外观,因此只替换了数字,而空格则保持不变。
另外,请注意, replaceAll()
仅应在处理正则表达式时使用。 如果您不使用正则表达式,请改用.replace()
。 .replace()
也会替换所有实例,名称有点误导。
请注意,如果假定前后的空格数不同,则只需将String.format()
的最终参数更改为该数字后的空格数即可。
编辑 :
显然,我们对空间的定义不同。 我假设一定数量的空格从字面上意味着在所讨论的编号之前和之后的一定数量的空格。
尝试,
String str="1111 1.0 2222 53.5 3333 0.3 4444 541.1 ";
str=str.replaceAll(" 0.3 ", " 32.2 ");
System.out.println(str);
假设您要替换String中所有0.3
s(已测试并且可以正常运行 ):
String s = "1111 1.0 2222 53.5 3333 0.3 4444 541.1";
// replace "0.3 " at the beginning of the String
if (s.substring(0,4).equals("0.3 "))
s = "32.2" + s.substring(3);
// replace " 0.3" at the end of the String
if (s.substring(s.length() - 4).equals(" 0.3"))
s = s.substring(0, s.length() - 4) + " 32.2";
// replace all the rest of the "0.3" in the String
s = s.replaceAll(" 0.3 ", " 32.2 ");
输入:
“ 1111 1.0 2222 53.5 3333 0.3 4444 541.1”
输出:
“ 1111 1.0 2222 53.5 3333 32.2 4444 541.1”
输入:
“ 0.3 1111 1.0 2222 53.5 3333 0.3 4444 541.1 0.3”
输出:
“ 32.2 1111 1.0 2222 53.5 3333 32.2 4444 541.1 32.2”
输入:
“ 0.35 1111 1.0 2222 53.5 3333 0.3 4444 540.3”
输出:
“ 0.35 1111 1.0 2222 53.5 3333 32.2 4444 540.3”
尝试使用此正则表达式\\b0\\.3\\b
。
\\b
是单词边界,可将0.3视为单词。
0是0
\\.
代表点文字。 您必须对点进行转义,因为正则表达式中的点将匹配任何字符。
3是文字3。
请记住,在Java中,您必须转义反斜杠,因此最终的正则表达式是这样。
String regexp = "\\\\b0\\\\.3\\\\b";
Junit测试示例
package demo;
import static org.junit.Assert.*;
import org.junit.Test;
public class Matchy {
String regexp = "\\b0\\.3\\b";
@Test
public void test() {
String str = "0.3 0.2 123.4 0.3 0.3 013";
String result = str.replaceAll(this.regexp, "32.2");
String expect = "32.2 0.2 123.4 32.2 32.2 013";
assertEquals(str, expect, result);
}
@Test
public void test2() {
String str = "1111 1.0 2222 53.5 3333 0.3 4444 541.1";
String result = str.replaceAll(this.regexp, "32.2");
String expect = "1111 1.0 2222 53.5 3333 32.2 4444 541.1";
assertEquals(str, expect, result);
}
}
我认为这段代码应该做您想要的。
String str = "1111 1.0 2222 53.5 3333 0.3 4444 541.1 5555 0.3";
int numSpaces = 5;
String replacement = "32.2";
Matcher m = Pattern.compile("((?:\\S+\\s+){" + numSpaces + "})(\\S+)(.*)").matcher(str);
m.find();
System.out.println("replaced " + m.group(2) + " with " + replacement + " at index " + m.start(2));
System.out.println(m.group(1) + replacement + m.group(3));
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.