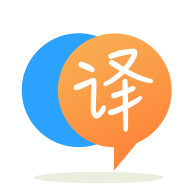
[英]How to update backing bean when deselecting the last slected item from a p:selectCheckboxMenu with a primefaces ajax event “change”?
[英]Resetting Bean when using Ajax events in PrimeFaces
我有一个对话框,其中包含复选框和一个输入字段。 选中此复选框后,应禁用输入。 复选框值存储在Bean中,输入值是所选对象值之一。
当用户单击列表上的链接时,会弹出此对话框。 Dialog的输入正在填充模型中的值。
我还有两个按钮:保存和取消。 在保存时,根据复选框值,我正在对模型进行不同的操作并更新它。 取消时我想什么都不做。
问题是:当有复选框连接的ajax事件时,Bean中的值会自动更新。 因此,在单击取消并重新打开对话框后,我获得了最后状态,但我想要初始状态。
这是代码:
名单
<h:form id="termPanelForm">
<p:dataTable id="termPanelTable"
value="#{termRightPanelController.terms}" var="term" emptyMessage="">
<p:column>
<p:commandLink value="#{term.subject}"
action="#{termRightPanelController.setTerm(term)}"
oncomplete="termRealizeDlg.show();"
update=":termRealizeForm:termRealizeDialog" />
</p:column>
<p:column width="50">
<h:outputText value="#{term.dateRealization}">
<f:convertDateTime pattern="dd-MM-yy HH:mm" />
</h:outputText>
</p:column>
</p:dataTable>
</h:form>
对话
<h:form id="termRealizeForm">
<p:dialog id="termRealizeDialog" widgetVar="termRealizeDlg"
modal="true" resizable="false" closeOnEscape="true"
header="#{termRightPanelController.selectedTerm.subject}">
<p:messages autoUpdate="true" showDetail="true"></p:messages>
<p:panelGrid columns="2" styleClass="border-none">
<p:outputLabel for="realized" value="#{i18n['Term.MarkRealized']}" />
<p:selectBooleanCheckbox id="realized"
value="#{termRightPanelController.termRealized}">
<p:ajax process="@this" update="dateRealization" />
</p:selectBooleanCheckbox>
<p:outputLabel for="dateRealization"
value="#{i18n['Term.ChangeDateRealization']}" />
<p:inputText id="dateRealization"
value="#{termRightPanelController.selectedTerm.dateRealization}"
pattern="dd-MM-yyyy HH:mm"
disabled="#{termRightPanelController.termRealized}" >
</p:inputText>
</p:panelGrid>
<h:panelGroup layout="block" styleClass="buttons-group">
<p:commandButton value="#{i18n['Common.Save']}"
oncomplete="hideDialogWhenValid(termRealizeDlg, xhr, status, args);"
action="#{termRightPanelController.editTerm()}"
update=":termPanelForm:termPanelTable" icon="ui-icon-check"></p:commandButton>
<p:commandButton onclick="termRealizeDlg.hide();"
value="#{i18n['Common.cancel']}"
icon="ui-icon-cancel" update=":termRealizeForm:termRealizeDialog">
</p:commandButton>
</h:panelGroup>
</p:dialog>
</h:form>
豆角,扁豆
@SuppressWarnings("rawtypes")
@ManagedBean
@ViewScoped
public class TermRightPanelController extends MainController implements Serializable {
private static final long serialVersionUID = 6283828584670862366L;
private TermServiceLocal termService = ServiceLocator.locateService(
TermService.class, TermServiceLocal.class);
private List<Term> terms;
private Term selectedTerm;
private boolean termRealized;
@PostConstruct
public void init() {
loadTerms();
}
public void loadTerms() {
terms = termService.getTermsNotRealized(getLoggedUser().getId());
}
public String expiredTerm(Term term) {
long time = new Date().getTime();
if (term.getDateRealization().getTime() > time) {
return "";
}
return "expired";
}
public void editTerm() {
if (termRealized) {
selectedTerm.setDateRead(new Date());
} else {
selectedTerm.setDateRead(null);
}
termService.merge(selectedTerm);
loadTerms();
}
public void setTerm(Term term) {
this.selectedTerm = term;
}
public List<Term> getTerms() {
return terms;
}
public void setTerms(List<Term> terms) {
this.terms = terms;
}
public Term getSelectedTerm() {
return selectedTerm;
}
public void setSelectedTerm(Term selectedTerm) {
this.selectedTerm = selectedTerm;
}
public boolean isTermRealized() {
return termRealized;
}
public void setTermRealized(boolean termRealized) {
this.termRealized = termRealized;
}
@Override
public Class getModelClass() {
return this.getClass();
}
}
在这个特定场景中,当我单击取消时,我总是可以将termRealized
设置为false,但在其他情况下,我有从模型加载的值。 因此,在重新打开对话框后,我想拥有与模型中相同的值,而不是由Bean存储。
我们在团队中讨论了一些可能的解决方案,但对我们来说,似乎都很讨厌:
selectedTerm
并在取消时从克隆重新加载它。 这很讨厌,因为我们需要在我们想要编辑的每个元素上实现可克隆的接口。 selectedTerm
,然后在terms
列表中替换它。 但为什么我们不得不再次从DB获得一些东西? terms
列表。 这比第三种解决方案更糟糕。 我们还尝试了内置的重置选项(resetInput标签和其他),但没有用。 有没有任何优雅的方法来解决这个问题,或者我们的一个建议是否正确?
我会用以下方式做到这一点。
为Term创建一个复制构造函数 :
public class Term {
private String dummy;
public Term() {
}
//copy constructor
public Term(Term another) {
this.dummy = another.dummy; // and set the rest of the values
}
}
dataTable中的按钮调用select方法。
<p:commandLink value="#{term.subject}"
action="#{termRightPanelController.selectTerm(term)}"
oncomplete="termRealizeDlg.show();"
update=":termRealizeForm:termRealizeDialog" />
selectTerm(术语)
public void selectTerm(Term term) {
selectedTerm = new Term(term);
}
对话onHide
<p:dialog id="termRealizeDialog" widgetVar="termRealizeDlg"
modal="true" resizable="false" closeOnEscape="true"
header="#{termRightPanelController.selectedTerm.subject}"
onHide="restDialog()">
restDialog() remoteCommand
<p:remoteCommand name="restDialog"
actionListener="#{termRightPanelController.restDialogValues()}" />
restDialogValues()
public void restDialogValues() {
setTermRealized(false);
}
保存按钮
<p:commandButton value="#{i18n['Common.Save']}"
action="#{termRightPanelController.editTerm()}" />
editTerm()
public void editTerm() {
....
terms.remove(selectedTerm);//assuming equals and hashcode works on ID
terms.add(selectedTerm);
// Or loop over the terms and find your selectedTerm by id
// if the equals and hashcode are not implemented on the ID,
//then remove it and add the new one
....
}
希望这可以帮助。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.