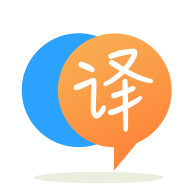
[英]How to calculate time complexity of a modified quick sort mixed with insertion sort?
[英]Using insertion sort with quick sort
为什么大多数人为什么对元素少于n的子数组使用插入排序来优化快速排序? 我写了一个插入排序函数和shell排序函数,并用几个具有10、50、100个元素的随机数组来调用它们。 似乎shell排序更快(我只用clock()测量了时间;我不知道这是不是一种好方法)。 如果它比插入排序快,为什么没有更多的人使用外壳排序? 插入排序功能是否有误?
我的代码:
double insertionSort(int *a, int size)
{
clock_t start, end;
int i, temp, pos;
start = clock();
for (i = 1; i < size; i++) {
temp = a[i];
pos = i - 1;
while (pos >= 0 && a[pos] > temp) {
a[pos + 1] = a[pos];
pos--;
}
a[pos + 1] = temp;
}
end = clock();
return (double)(end - start) / CLOCKS_PER_SEC * 1000;
}
double shellSort(int *a, int size)
{
int gaps[8] = {701, 301, 132, 57, 23, 10, 4, 1};
int i, k , temp, pos;
clock_t start, end;
start = clock();
for (i = 0; i < 8; i++)
for (k = gaps[i]; k < size; k++) {
temp = a[k];
pos = k - gaps[i];
while (pos >= 0 && a[pos] > temp) {
a[pos + gaps[i]] = a[pos];
pos -= gaps[i];
}
a[pos + gaps[i]] = temp;
}
end = clock();
return (double)(end - start) / CLOCKS_PER_SEC * 1000;
}
输出5:
Insertion Sort : Sorted in 0.002000 milliseconds
Shell Sort : Sorted in 0.001000 milliseconds
10个输出:
Insertion Sort : Sorted in 0.004000 milliseconds
Shell Sort : Sorted in 0.001000 milliseconds
50的输出:
Insertion Sort : Sorted in 0.007000 milliseconds
Shell Sort : Sorted in 0.007000 milliseconds
100的输出:
Insertion Sort : Sorted in 0.015000 milliseconds
Shell Sort : Sorted in 0.014000 milliseconds
200的输出:
Insertion Sort : Sorted in 0.040000 milliseconds
Shell Sort : Sorted in 0.028000 milliseconds
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.