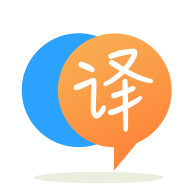
[英]ASP.NET MVC / JQuery/ Javascript: view will not refresh after searching (and trying to load) new data
[英]ASP.NET MVC4 Refresh view after adding a comment
我正在编写ASP.NET Web应用程序。 我正在使用Visual Studio生成的视图(创建/删除/详细信息/编辑/索引)。 在我的应用程序中,我有可以被登录用户评论的文章。 一切正常(添加并查看评论),但是要查看新添加的评论,我必须手动刷新页面。
Article / Details视图的结构如下:
@if (Request.IsAuthenticated)
{
using (Ajax.BeginForm("Create", "Comment", new { articleId = Model._article.ArticleId }, new AjaxOptions
{
HttpMethod = "post",
InsertionMode = InsertionMode.Replace,
UpdateTargetId = "myDisplayID"
}))
{
@Html.ValidationSummary(true)
<fieldset>
<h4>Dodaj komentarz</h4>
<p>
@Html.TextArea("komentarz", new { @class = "form-control" })
</p>
<p>
<input type="submit" value="Dodaj" class="btn btn-primary btn-large" />
</p>
</fieldset>
}
}
else
{
<p>
<a href="~/Account/Login">Zaloguj się</a> aby dodać komentarz.
</p>
}
<hr />
<div id="myDisplayID">
@Html.Partial("_Comment", Model._comment)
</div>
编辑注释/创建GET方法
public ActionResult Create(int articleId)
{
var komentarz = new Comment();
komentarz.ArticleId = articleId;
return View(komentarz);
}
评论/创建POST
[HttpPost]
public ActionResult Create(Comment comment, string komentarz, int articleId)
{
if (komentarz != String.Empty)
{
if (ModelState.IsValid)
{
comment.UserId = (int)WebSecurity.GetUserId(User.Identity.Name);
comment.Content = komentarz;
comment.ArticleId = articleId;
db.Comments.Add(comment);
db.SaveChanges();
}
}
ArticleViewModel widok = new ArticleViewModel();
widok._comment = (from b in db.Comments where b.ArticleId == articleId select b).ToList();
return PartialView("_Comment", widok._comment);
}
文章/详细信息GET方法
public ActionResult Details(int id = 0)
{
ArticleViewModel widok = new ArticleViewModel();
widok._comment = (from b in db.Comments where b.ArticleId == id select b).ToList();
widok._article = (from t in db.Articles where t.ArticleId == id select t).FirstOrDefault();
if (Request.IsAjaxRequest())
{
return PartialView("_Comment", widok._comment);
}
if (widok == null)
{
return HttpNotFound();
}
return View(widok);
}
如果要使用Ajax.BeginFrom,请参见所示。 (但有一些修改)
using (Ajax.BeginForm(new AjaxOptions {
HttpMethod= "get",
InsertionMode=InsertionMode.Replace,
UpdateTargetId = "myDisplayID"
}))
,您需要3件事。
。
public ActionResult Details()
{
//what ever the data retrieve code you have
return View(alldata);
}
[HttpPost]
public ActionResult Create(Comment comment, string komentarz)
{
if (komentarz != String.Empty)
{
if (ModelState.IsValid)
{
comment.UserId = (int)WebSecurity.GetUserId(User.Identity.Name);
comment.Content = komentarz;
db.Comments.Add(comment);
db.SaveChanges();
}
}
ArticleViewModel widok = new ArticleViewModel();
widok._comment = (from b in db.Comments where b.ArticleId == id select b).ToList();
return PartialView("_Comments",widok._comment);
}
提交评论-查看
using (Ajax.BeginForm("Create", new AjaxOptions
{
HttpMethod = "POST",
InsertionMode = InsertionMode.Replace,
UpdateTargetId = "myDisplayID"
}))
{
@Html.ValidationSummary(true)
<fieldset>
<h4>Dodaj komentarz</h4>
<p>
@Html.TextArea("komentarz", new { @class = "form-control" })
</p>
<p>
<input type="submit" value="Dodaj" class="btn btn-primary btn-large" />
</p>
</fieldset>
}
在您看来而不是
<h4>Comments</h4>
<table>
// table with comments for this article
</table>
放
<div id="myDisplayID">
@Html.Partial("_Comments", Model.Comments)
</div>
_评论部分视图
@model IEnumerable<Comments>
<h4>Comments</h4>
<table>
// table with comments for this article
</table>
我认为,如果您也碰巧使用Ajax添加新评论,以这种方式进行操作将改善用户体验
您可以使用window.location.href = window.location.href在javascript中刷新。 重新加载再次发布数据。
最好的选择是发送带有ajax调用的帖子请求,如下所示:
var comment = $("#txtComment).val(); // change this id with your textarea id
var id = @Model._article.ArticleId;
$.ajax({
url: "/Comment/Create",
type: "POST",
dataType: "json",
data: JSON.stringify({ content: comment, articleId: id }),
contentType: "application/json; charset=utf-8",
success: function (data) {
// if you returning all your comment use this
$("table").html(data.allContent);
// or if you return just a row with this comment
$("table").prepend(data.Comment);
}
我假设您的Action方法是这样的:
[HttpPost]
public ActionResult Create(string content,int articleId)
{
...
return Json(new { allContent = comments }); // just for example
}
通过改变解决问题
return View()
在POST中创建操作以
ControllerContext.HttpContext.Response.Redirect(ControllerContext.HttpContext.Request.Url.ToString());
没有任何局部视图,AJAX和Jquery
尝试这个 :
return RedirectToAction("Index", "Comments");
或但是您的控制器被调用。 但..
对我来说最好的解决方案是使用jquery ajax异步添加评论。 Comment/Create
应该返回html作为响应(partialview),并且您应该用新表替换旧表。
$.ajax({
url: "Comments/Create",
data: {comment : "bla bla", articleId : 1},
success: function (response) {
if (response == 'True') {
$('table').html(response);
}
}
});
查看一些jquery ajax教程,这对于此类事情非常有用。 您还可以在触发操作时使用javascript,这将准备一些带有另一行的html代码,并将其附加到表中。 这两种方式都很不错,因为您不必强制重新加载页面。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.