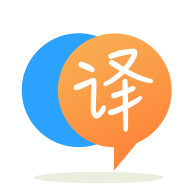
[英]Java locks: How equality check for Monitor locks is done in synchronized block?
[英]How to atomically check TWO AtomicBooleans in Java in one safe operation without a synchronized block (i.e. low cost locks)?
所以我有两个AtomicBoolean,我需要检查两个。 像这样:
if (atomicBoolean1.get() == true && atomicBoolean2.get() == false) {
// ...
}
但是在两者之间有一个竞争条件:(
有没有一种方法可以在不使用同步的情况下将两个原子布尔检查合并为一个检查(即同步块)?
好吧,我可以想到几种方法,但这取决于您所需的功能。
一种方法是“作弊”并使用AtomicMarkableReference <Boolean> :
final AtomicMarkableReference<Boolean> twoBooleans = (
new AtomicMarkableReference<Boolean>(true, false)
);
void somewhere() {
boolean b0;
boolean[] b1 = new boolean[1];
b0 = twoBooleans.get(b1);
b0 = false;
b1[0] = true;
twoBooleans.set(b0, b1);
}
但这有点痛苦,只能让您获得两个价值。
因此,您可以将AtomicInteger与位标志一起使用:
static final int FLAG0 = 1;
static final int FLAG1 = 1 << 1;
final AtomicInteger intFlags = new AtomicInteger(FLAG0);
void somewhere() {
int flags = intFlags.get();
int both = FLAG0 | FLAG1;
if((flags & both) == FLAG0) { // if FLAG0 has a 1 and FLAG1 has a 0
something();
}
flags &= ~FLAG0; // set FLAG0 to 0 (false)
flags |= FLAG1; // set FLAG1 to 1 (true)
intFlags.set(flags);
}
也有点痛苦,但它可以为您提供32个值。 如果您确实需要,可以围绕它创建一个包装器类。 例如:
public class AtomicBooleanArray {
private final AtomicInteger intFlags = new AtomicInteger();
public void get(boolean[] arr) {
int flags = intFlags.get();
int f = 1;
for(int i = 0; i < 32; i++) {
arr[i] = (flags & f) != 0;
f <<= 1;
}
}
public void set(boolean[] arr) {
int flags = 0;
int f = 1;
for(int i = 0; i < 32; i++) {
if(arr[i]) {
flags |= f;
}
f <<= 1;
}
intFlags.set(flags);
}
public boolean get(int index) {
return (intFlags.get() & (1 << index)) != 0;
}
public void set(int index, boolean b) {
int f = 1 << index;
int current, updated;
do {
current = intFlags.get();
updated = b ? (current | f) : (current & ~f);
} while(!intFlags.compareAndSet(current, updated));
}
}
很好 也许在get中复制数组时执行了一个设置,但要点是您可以原子获取或设置所有32个。 (compare和set的do-while循环很丑陋,但这是原子类自身如何处理诸如getAndAdd之类的东西的方法 。)
在这里,AtomicReference似乎不切实际。 它允许原子获取和设置,但是一旦您使用内部对象,就不再需要原子更新。 您每次都必须创建一个全新的对象。
final AtomicReference<boolean[]> booleanRefs = (
new AtomicReference<boolean[]>(new boolean[] { true, true })
);
void somewhere() {
boolean[] refs = booleanRefs.get();
refs[0] = false; // not atomic!!
boolean[] copy = booleanRefs.get().clone(); // pretty safe
copy[0] = false;
booleanRefs.set(copy);
}
如果要自动对数据执行临时操作(获取->更改->设置,而不会产生干扰),则必须使用锁定或同步。 我个人会使用锁定或同步,因为通常情况下,整个更新就是您要保留的内容。
不要这样!
这可以(可能)通过sun.misc.Unsafe
完成。 这是一个使用不安全的类来写两个易变的长牛仔风格的类。
public class UnsafeBooleanPair {
private static final Unsafe UNSAFE;
private static final long[] OFFS = new long[2];
private static final long[] MASKS = new long[] {
-1L >>> 32L, -1L << 32L
};
static {
try {
UNSAFE = getTheUnsafe();
Field pair = UnsafeBooleanPair.class.getDeclaredField("pair");
OFFS[0] = UNSAFE.objectFieldOffset(pair);
OFFS[1] = OFFS[0] + 4L;
} catch(Exception e) {
throw new RuntimeException(e);
}
}
private volatile long pair;
public void set(int ind, boolean val) {
UNSAFE.putIntVolatile(this, OFFS[ind], val ? 1 : 0);
}
public boolean get(int ind) {
return (pair & MASKS[ind]) != 0L;
}
public boolean[] get(boolean[] vals) {
long p = pair;
vals[0] = (p & MASKS[0]) != 0L;
vals[1] = (p & MASKS[1]) != 0L;
return vals;
}
private static Unsafe getTheUnsafe()
throws Exception {
Field theUnsafe = Unsafe.class.getDeclaredField("theUnsafe");
theUnsafe.setAccessible(true);
return (Unsafe)theUnsafe.get(null);
}
}
重要的是, Open JDK源代码中的fieldOffset
的Javadoc表示不要对偏移量进行算术运算。 但是,对它进行算术运算似乎很有效,因为我不会得到垃圾。
这样可以对整个单词进行一次易失性读取,但是(可能)对其中任何一半进行易失性写入。 潜在的putByteVolatile
可以用于将很长的段分成8个段。
我不建议任何人使用此功能(不要使用此功能!),但是这很有趣。
我只能想到两种方式:使用AtomicInteger
的低两位或使用自旋锁。 我认为Hotspot可以自行优化某些锁,直至自旋锁。
使用锁 :
Lock l = ...;
l.lock();
try {
// access the resource protected by this lock
} finally {
l.unlock();
}
从技术上讲,它不是一个同步块,即使它是一种同步形式,我认为您要的是同步的定义,所以我认为“不进行同步”是不可能的。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.