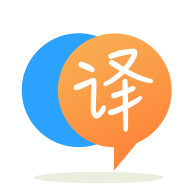
[英]Finding the Average in a sequence of number in c while ignoring the negative numbers in the sequence
[英]Average of # Sequence
我在查找平均函数中的循环时遇到了麻烦。 用户输入序列,如果序列中的数字大于0,则输出数字的平均值并重复另一个序列。 如果序列没有数字,退出程序。
我明白,当sum = 0时,我告诉平均函数返回0,这就是为什么它在第一个序列之后退出(我认为)。
有关如何避免这种情况的任何建议? 伪代码如果可能的话!
#include<stdio.h>
#include<stdlib.h>
double average();
int main ()
{
while( average() )
{
}
}//end main
double average()
{
double n, sum = 0;
int count = 0;
printf ( "Enter sequence: " );
while( scanf( "%lf", &n ) )
{
sum += n;
count++;
}
if( sum > 0 )
{
printf( "average is %.2f\n", sum/(double)count );
return 1;
}
else
return 0;
}
}
这是我的输出:
Enter sequence: 3 4 5 x
average: 4.00
Enter sequence: Press any key to continue . . .
#include <stdio.h>
#include <stdlib.h>
int average(void);
int main(void)
{
while (average())
;
return 0;
}
int average(void)
{
double n, sum = 0;
int count = 0;
printf("Enter sequence: ");
while (scanf( "%lf", &n ) == 1)
{
sum += n;
count++;
}
int c;
while ((c = getchar()) != EOF && c != '\n')
;
if (sum > 0)
{
printf("average is %.2f\n", sum / count);
return 1;
}
else
return 0;
}
这会在转换失败后读取换行符中的任何内容,从而为读取下一个序列做好准备。 scanf()
的循环测试得到改进; 它也会在EOF上退出。 分裂中的演员是不必要的; 编译器必须将count
转换为double
因为sum
是一个double
,即使你没有告诉它明确地这样做。 average()
的返回类型不是double; 它是一个布尔值,通常拼写为int
。 在C99或更高版本中(代码假设你有;如果你没有,你就被困在Windows上并且需要移动int c;
到函数的顶部),那么你可以#include <stdbool.h>
和使用返回类型的bool
并替换return 1;
通过return true;
并替换return 0;
通过return false;
。
我想你最初可以获得名为count的变量的输入,要求用户按顺序输入数字的总数,然后按顺序获取这些值。 如果计数为0,则退出程序..否则继续查找平均值。
这是因为在您的情况下,每次都必须输入一个非数字字符来结束序列。
好吧,我最终得到了这个并且它完美地工作:
#include<stdio.h>
#include<stdlib.h>
#include<ctype.h>
double average();
int main () {
while( average() ) {}
}//end main
double average() {
double n, sum = 0;
int count = 0;
printf ( "Enter sequence: " );
getchar();
while( scanf( "%lf", &n ) ){
sum += n;
count++;
}//end while
if( n > 0 ){
printf( " average: %.2f\n", sum/(double)count );
return 1;
}//end if
else
return 0;
}//end function
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.