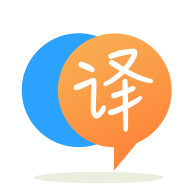
[英]How to start activities by swiping different listview items in android?
[英]Android item swiping on listview
几天以来,我一直在与Android Listview
上的这个主题苦苦挣扎,但似乎做得不好。 我只是不明白该怎么做。 我已经对Adapters
(特别是BaseAdapter
)学到了最好的知识,但仍然想不出办法。
我在网上搜索了一些信息,但并没有完全了解。
ListView
。 我只是不能做到这一点,所以请你帮忙。 非常感谢您的时间和精力
将这样的动画添加到ListView没问题,我在几分钟内为普通的非自定义ListView构建了此解决方案,通常这种情况适用于任何开箱即用的ListView,它们全都在适配器中。 我的答案中唯一缺少的是滑动检测,很遗憾,我现在没有时间对其进行测试。 但是滑动检测并不困难,如果您使用Google进行滑动检测,则有很多示例。 无论如何,如果您有任何疑问,请随时提出。
结果:
我使用的是带有ViewHolder模式的简单BaseAdapter,没什么特别的,无论如何我都会发布getView方法以进行说明:
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if(getItemId(position) == TEST_VIEW_ID) {
TestViewModel viewModel = (TestViewModel) getItem(position);
TestRow row;
if(convertView == null) {
convertView = this.inflater.inflate(TestRow.LAYOUT, parent, false);
row = new TestRow(convertView); // Here the magic happens
convertView.setTag(row);
}
row = (TestRow) convertView.getTag();
row.bind(viewModel);
}
return convertView;
}
在我的ViewHolder类(这里称为TestRow
我为动画创建了一些帮助器方法,下面我将进一步解释它们,但首先,我的代码来自TestRow
:
public class TestRow {
public static final int LAYOUT = R.layout.list_item_test;
public ImageView ivLogo;
public TextView tvFadeOut;
public TextView tvSlideIn;
public TestRow(View view) {
this.ivLogo = (ImageView) view.findViewById(R.id.ivLogo);
this.tvFadeOut = (TextView) view.findViewById(R.id.tvFadeOut);
this.tvSlideIn = (TextView) view.findViewById(R.id.tvSlideIn);
this.ivLogo.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// When the ImageView is clicked the animations are applied to the TextViews.
if(tvFadeOut.getVisibility() == View.VISIBLE) {
fadeOutView(tvFadeOut);
slideInView(tvSlideIn);
} else {
fadeInView(tvFadeOut);
slideOutView(tvSlideIn);
}
}
});
}
public void bind(TestViewModel viewModel) {
// Nothing to do here
}
}
这是我用于动画的辅助方法:
private void fadeOutView(View view) {
Animation fadeOut = AnimationUtils.loadAnimation(view.getContext(), R.anim.fade_out);
if (fadeOut != null) {
fadeOut.setAnimationListener(new ViewAnimationListener(view) {
@Override
protected void onAnimationStart(View view, Animation animation) {
}
@Override
protected void onAnimationEnd(View view, Animation animation) {
view.setVisibility(View.GONE);
}
});
view.startAnimation(fadeOut);
}
}
private void fadeInView(View view) {
Animation fadeIn = AnimationUtils.loadAnimation(view.getContext(), R.anim.fade_in);
if (fadeIn != null) {
fadeIn.setAnimationListener(new ViewAnimationListener(view) {
@Override
protected void onAnimationStart(View view, Animation animation) {
view.setVisibility(View.VISIBLE);
}
@Override
protected void onAnimationEnd(View view, Animation animation) {
}
});
view.startAnimation(fadeIn);
}
}
private void slideInView(View view) {
Animation slideIn = AnimationUtils.loadAnimation(view.getContext(), R.anim.slide_in_right);
if (slideIn != null) {
slideIn.setAnimationListener(new ViewAnimationListener(view) {
@Override
protected void onAnimationStart(View view, Animation animation) {
view.setVisibility(View.VISIBLE);
}
@Override
protected void onAnimationEnd(View view, Animation animation) {
}
});
view.startAnimation(slideIn);
}
}
private void slideOutView(View view) {
Animation slideOut = AnimationUtils.loadAnimation(view.getContext(), R.anim.slide_out_right);
if (slideOut != null) {
slideOut.setAnimationListener(new ViewAnimationListener(view) {
@Override
protected void onAnimationStart(View view, Animation animation) {
}
@Override
protected void onAnimationEnd(View view, Animation animation) {
view.setVisibility(View.GONE);
}
});
view.startAnimation(slideOut);
}
}
private abstract class ViewAnimationListener implements Animation.AnimationListener {
private final View view;
protected ViewAnimationListener(View view) {
this.view = view;
}
@Override
public void onAnimationStart(Animation animation) {
onAnimationStart(this.view, animation);
}
@Override
public void onAnimationEnd(Animation animation) {
onAnimationEnd(this.view, animation);
}
@Override
public void onAnimationRepeat(Animation animation) {
}
protected abstract void onAnimationStart(View view, Animation animation);
protected abstract void onAnimationEnd(View view, Animation animation);
}
这些是我使用的动画xml:
淡入:
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:shareInterpolator="false">
<alpha
android:fromAlpha="0"
android:toAlpha="1"
android:duration="700"/>
</set>
淡出:
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:shareInterpolator="false">
<alpha
android:fromAlpha="1"
android:toAlpha="0"
android:duration="700"/>
</set>
滑入:
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:shareInterpolator="false">
<translate
android:fromXDelta="100%" android:toXDelta="0%"
android:duration="700"/>
</set>
滑出:
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:shareInterpolator="false">
<translate
android:fromXDelta="0%" android:toXDelta="100%"
android:duration="700"/>
</set>
在res / anim /中使用此xml(用于动画目的)
这是从左到右的动画:
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:shareInterpolator="false">
<translate android:fromXDelta="-100%" android:toXDelta="0%"
android:fromYDelta="0%" android:toYDelta="0%"
android:duration="700"/>
</set>
这是从右到左的动画:
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:shareInterpolator="false">
<translate
android:fromXDelta="0%" android:toXDelta="100%"
android:fromYDelta="0%" android:toYDelta="0%"
android:duration="700" />
</set>
在编码中,请使用从左到右的意图:
this.overridePendingTransition(R.anim.animation_enter,
R.anim.animation_leave);
在编码中使用意图,例如从右到左
this.overridePendingTransition(R.anim.animation_leave,
R.anim.animation_enter);
对于自定义列表视图,您可以使用以下代码: http : //www.androidhive.info/2012/02/android-custom-listview-with-image-and-text/
只需捕捉手势事件并应用动画,然后制作一种布局即可在手势事件中消失。
如果您将其用于正常用途,而不是您想要的实际工作示例,请访问: https : //github.com/47deg/android-swipelistview
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.