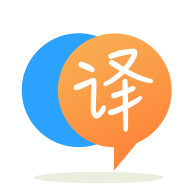
[英]How should I implement Comparable when equals() and hashCode() are not defined?
[英]How to implement equals and comparable in this TreeMap
如何在此树形图中实现可比较和等于方法,以便我的包含值返回true。
怎么做?
如何实施?
import java.util.*;
class a
{
public static void main(String arr[])
{
TreeMap<String,Emp> map=new TreeMap<String,Emp>();
map.put("HEllo",new Emp("ada",23));
map.put("aehqn",new Emp("rewr",343));
map.put("rffewrf",new Emp("saerfwe",893743));
Set<Map.Entry<String,Emp>> x=map.entrySet();
Iterator<Map.Entry<String,Emp>> itr =x.iterator();
while(itr.hasNext())
{
Map.Entry<String,Emp> m=itr.next();
System.out.println(m.getKey());
Emp e=m.getValue();
e.display();
System.out.println();
}
System.out.println("NOw the value we will finid is"+map.containsValue(new Emp("ada",23)));
}
}
class Emp
{
String n;
int i;
public Emp(String n,int i)
{
this.n=n;
this.i=i;
}
public void display()
{
System.out.println("there are string "+n+" int"+i);
}
}
提前致谢
您的代码看起来像
class Emp implements Comparable<Emp>
{
String n;
int i;
public Emp(String n,int i)
{
this.n=n;
this.i=i;
}
public void display()
{
System.out.println("there are string "+n+" int"+i);
}
public boolean equals(Object o){
if(o instanceof Emp){
Emp d = (Emp)o;
return ((d.n.equals(n)) && (d.i==i));
}
return false;
}
public int hashCode(){
return i/2 + 17;
}
public int compareTo(Emp d){
if(this.i>d.i)
return 1;
else if(this.i<d.i)
return -1;
return this.n.compareTo(d.n);
}
}
如果有任何语法错误,请忽略,您也可以改进方法实现。
Map接口的containsValue()方法使用Object类的equals()方法。在你的情况下,你必须覆盖equals()方法,当你重写equals()方法时,建议覆盖hashCode( )方法也是。 以下是正确的代码: -
public class a
{
public static void main(String arr[])
{
TreeMap<String,Emp> map=new TreeMap<String,Emp>();
map.put("HEllo",new Emp("ada",23));
map.put("aehqn",new Emp("rewr",343));
map.put("rffewrf",new Emp("saerfwe",893743));
Set<Map.Entry<String,Emp>> x=map.entrySet();
Iterator<Map.Entry<String,Emp>> itr =x.iterator();
while(itr.hasNext())
{
Map.Entry<String,Emp> m=itr.next();
System.out.println(m.getKey());
Emp e=m.getValue();
e.display();
}
System.out.println("Now the value we will find is"+map.containsValue(new Emp("ada",23)));
}
}
class Emp
{
String n;
int i;
public Emp(String n,int i)
{
this.n=n;
this.i=i;
}
public void display()
{
System.out.println("there are string "+n+" int"+i);
}
@Override
public boolean equals(Object obj) {
if(obj==null)
{
return false;
}
if(this.getClass().equals(obj.getClass()))
return true;
else
return false;
}
@Override
public int hashCode() {
return super.hashCode();
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.