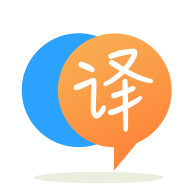
[英]How to create a String with String.format by taking parameters from a list
[英]How would I create the function for sending an email with the parameters set as String from, String to, String Subject, and String body?
我正在尝试创建一个Java批处理电子邮件程序,该程序会将电子邮件发送到带有excel报告附件的特定收件箱。 我有功能:
public void sendEmail(String to, String from, String subject, String body)
{
}
我正在尝试使用Spring,并且现在我试图坚持在appcontext文件中进行xml配置,而不是使用注释(出于学习目的)。 我想注入一个静态资源,它是一个excel文件,出于学习目的,我避免在我的导师/老师的附件中使用FileSystemResource。 我也不需要身体说什么。 出于虚假目的,主题行将为“报告”。 到目前为止,这就是我所需要的,只需要实际的电子邮件功能即可,因此我可以在主类中通过引用传递sendEmail的参数:
public class SendEmail
{
private JavaMailSender mailSender;
public SendEmail(JavaMailSender ms)
{
this.mailSender = ms;
}
public void sendEmail(String from, String to, String Subject, String body)
{
MimeMessage message = mailSender.createMimeMessage();
try
{
MimeMessageHelper helper = new MimeMessageHelper(message);
helper.setTo("whatever@xyz.com");
helper.setText("Test email!");
mailSender.send(message);
}
catch (MessagingException e)
{
throw new MailParseException(e);
}
}
public void setMailSender(JavaMailSender mailSender)
{
this.mailSender = mailSender;
}
}
这是applicationContext.xml代码:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation=`
"http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.2.xsd">
<context:component-scan base-package="com.transportation"/>
<bean id = "mailSender" class = "org.springframework.mail.javamail.JavaMailSenderImpl">
<property name = "host" value = "Whatever" />
<property name = "port" value = "25" />
</bean>
<bean id = "sendEmail" class = "com.transportation.email.util.SendEmail">
<constructor-arg ref="mailSender"/>
</bean>
</beans>
试试这个。
public void sendMail(final String messageStr, final boolean isHtml) throws MessagingException {
final MimeMessage message = mailSender.createMimeMessage();
final MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setFrom(simpleMailMessage.getFrom());
helper.setTo(simpleMailMessage.getTo());
helper.setCc(simpleMailMessage.getCc());
helper.setSubject(simpleMailMessage.getSubject());
helper.setText(messageStr, isHtml);
helper.addInline("myFile", ResourceUtil.loadResourceAsFileSystemResource("NameOfresource"));
mailSender.send(message);
}
public static FileSystemResource loadResourceAsFileSystemResource(final String fileRoute) {
File file = null;
FileSystemResource fileSystemResource;
try {
file = new ClassPathResource(fileRoute).getFile();
fileSystemResource = new FileSystemResource(file);
}
catch (final IOException e) {
fileSystemResource = null;
}
return fileSystemResource;
}
<bean id="mailSender" class="org.springframework.mail.javamail.JavaMailSenderImpl">
<property name="javaMailProperties">
<props>
<prop key="mail.smtp.host">${mail.smtp.host}</prop>
<prop key="mail.smtp.port">${mail.smtp.port}</prop>
</props>
</property>
</bean>
<bean id="sfErrorMailSender" class="XXX.MailSender">
<property name="mailSender" ref="mailSender" />
<property name="simpleMailMessage" ref="sfErrorNotificationMailMessage" />
</bean>
<bean id="sfErrorNotificationMailMessage" class="org.springframework.mail.SimpleMailMessage">
<property name="from" value="${mail.message.error.sf.to}" />
<property name="to" value="${mail.message.error.sf.from}" />
<property name="subject" value="${mail.message.error.sf.subject}" />
<property name="text" value="${mail.message.error.sf.body}" />
<property name="cc" value="${mail.message.error.sf.cc}" />
</bean>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.