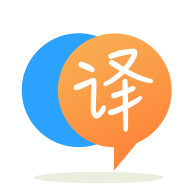
[英]How to add a new JPanel instance every time I click on JButton in JFrame in Java Swing
[英]Open new JFrame with JButton Click - Java Swing
我正在尝试使用按钮单击事件打开一个新的JFrame窗口。 这个网站上有很多信息,但没有任何帮助我,因为我认为它不是我的代码,而是它的执行顺序(但我不确定)。
这是包含我想要启动事件的按钮的框架的代码:
package messing with swing;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.*;
import javax.swing.border.EmptyBorder;
public class ReportGUI extends JFrame{
//Fields
private JButton viewAllReports = new JButton("View All Program Details");
private JButton viewPrograms = new JButton("View Programs and Majors Associated with this course");
private JButton viewTaughtCourses = new JButton("View Courses this Examiner Teaches");
private JLabel courseLabel = new JLabel("Select a Course: ");
private JLabel examinerLabel = new JLabel("Select an Examiner: ");
private JPanel panel = new JPanel(new GridLayout(6,2,4,4));
private ArrayList<String> list = new ArrayList<String>();
private ArrayList<String> courseList = new ArrayList<String>();
public ReportGUI(){
reportInterface();
allReportsBtn();
examinnerFileRead();
courseFileRead();
comboBoxes();
}
private void examinnerFileRead(){
try{
Scanner scan = new Scanner(new File("Examiner.txt"));
while(scan.hasNextLine()){
list.add(scan.nextLine());
}
scan.close();
}
catch (FileNotFoundException e){
e.printStackTrace();
}
}
private void courseFileRead(){
try{
Scanner scan = new Scanner(new File("Course.txt"));
while(scan.hasNextLine()){
courseList.add(scan.nextLine());
}
scan.close();
}
catch (FileNotFoundException e){
e.printStackTrace();
}
}
private void reportInterface(){
setTitle("Choose Report Specifications");
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
JPanel panel = new JPanel(new FlowLayout());
add(panel, BorderLayout.CENTER);
setSize(650,200);
setVisible(true);
setResizable(false);
setLocationRelativeTo(null);
}
private void allReportsBtn(){
JPanel panel = new JPanel(new GridLayout(1,1));
panel.setBorder(new EmptyBorder(70, 50, 70, 25));
panel.add(viewAllReports);
viewAllReports.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent e){
JFrame AllDataGUI = new JFrame();
new AllDataGUI();
}
});
add(panel, BorderLayout.LINE_END);
}
private void comboBoxes(){
panel.setBorder(new EmptyBorder(0, 5, 5, 10));
String[] comboBox1Array = list.toArray(new String[list.size()]);
JComboBox comboBox1 = new JComboBox(comboBox1Array);
panel.add(examinerLabel);
panel.add(comboBox1);
panel.add(viewTaughtCourses);
viewTaughtCourses.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JFrame ViewCourseGUI = new JFrame();
new ViewCourseGUI();
}
});
String[] comboBox2Array = courseList.toArray(new String[courseList.size()]);
JComboBox comboBox2 = new JComboBox(comboBox2Array);
panel.add(courseLabel);
panel.add(comboBox2);
panel.add(viewPrograms);
add(panel, BorderLayout.LINE_START);
}
如果您不想深入研究上面的代码,ActionListener按钮就在这里:
panel.add(viewTaughtCourses);
viewTaughtCourses.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JFrame ViewCourseGUI = new JFrame();
new ViewCourseGUI();
}
});
这是包含我想要打开的JFrame的类中的代码:
package messing with swing;
import java.awt.BorderLayout;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.border.EmptyBorder;
public class ViewCourseGUI extends JFrame{
private JButton saveCloseBtn = new JButton("Save Changes and Close");
private JButton closeButton = new JButton("Exit Without Saving");
private JFrame frame=new JFrame("Courses taught by this examiner");
private JTextArea textArea = new JTextArea();
public void ViewCoursesGUI(){
panels();
}
private void panels(){
JPanel panel = new JPanel(new GridLayout(1,1));
panel.setBorder(new EmptyBorder(5, 5, 5, 5));
JPanel rightPanel = new JPanel(new GridLayout(15,0,10,10));
rightPanel.setBorder(new EmptyBorder(15, 5, 5, 10));
JScrollPane scrollBarForTextArea=new JScrollPane(textArea,JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED,JScrollPane.HORIZONTAL_SCROLLBAR_AS_NEEDED);
panel.add(scrollBarForTextArea);
frame.add(panel);
frame.getContentPane().add(rightPanel,BorderLayout.EAST);
rightPanel.add(saveCloseBtn);
rightPanel.add(closeButton);
frame.setSize(1000, 700);
frame.setVisible(true);
frame.setLocationRelativeTo(null);
}
}
有人可以指点我正确的方向吗?
在actionListener中将要打开的JFrame的可见性设置为true:
ViewCourseGUI viewCourseGUI = new ViewCourseGUI();
viewCourseGUI.setVisible(true);
单击按钮后,这将打开新的JFrame窗口。
正如PM 77-3所指出的那样
我有:
public void ViewCoursesGUI(){
panels();
}
当我应该有:
public ViewCourseGUI(){
panels();
}
语法和拼写错误的组合。
让ReportGUI实现ActionListener。 然后,您将为按钮单击实现actionPerformed。 单击按钮,创建第二帧(如果它不存在)。 最后,将第二帧设置为可见(如果它当前不可见):
import java.awt.EventQueue;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
public class ReportGUI extends JFrame implements ActionListener {
private static final long serialVersionUID = 8679886300517958494L;
private JButton button;
private ViewCourseGUI frame2 = null;
public ReportGUI() {
//frame1 stuff
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300,200);
setLayout(new FlowLayout());
//create button
button = new JButton("Open other frame");
button.addActionListener(this);
add(button);
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
ReportGUI frame = new ReportGUI();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == button) {
if (frame2 == null)
frame2 = new ViewCourseGUI();
if (!frame2.isVisible())
frame2.setVisible(true);
}
}
}
这是一个简单的例子。 您必须在此处添加其余代码。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.