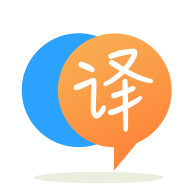
[英]Java - Get substring from 2nd last occurrence of a character in string
[英]How to split the string before the 2nd occurrence of a character in Java
我有:
1234 2345 3456 4567
当我尝试String.split(" ",2)
,我得到:
{1234} , {2345 3456 4567}
但是我需要:
{1234},{2345}
我只想要前两个元素。 我如何在 Java 中实现它?
提前致谢。
编辑:这只是庞大数据集的一行。
我假设您需要前两个字符串,然后执行以下操作:
String[] res = Arrays.copyOfRange(string.split(" "), 0, 2);
你可以这样做:-
String[] s=string.split("");
s[2]=null;
s[3]=null;
现在你只有 {1234},{2345}
或者更好的方法是,将字符串本身分开然后应用 split()
String s=string.substring(string.indexOf("1"),string.indexOf("5")+1);// +1 for including the 5
s.split(" ");
您有任何选择,一种是进行拆分并复制前 2 个结果。
下一个代码具有下一个输出:
前两个:
1234
2345
public static void main(String[] args) {
String input="1234 2345 3456 4567";
String[] parts= input.split(" ");
String[] firstTwoEntries = Arrays.copyOf(parts, 2);
System.out.println("First two: ");
System.out.println("----------");
for(String entry:firstTwoEntries){
System.out.println(entry);
}
System.out.println("----------");
}
另一种是用正则表达式替换原始字符串,然后进行拆分:
下一段代码的结果(我们用“:”替换空格:
过滤:1234:2345
前两个:
1234
2345
public static void main(String[] args) {
String input="1234 2345 3456 4567";
//we find first 2 and separate with :
String filteredInput= input.replaceFirst("^([^\\s]*)\\s([^\\s]*)\\s.*$", "$1:$2");
System.out.println("Filtered: "+filteredInput);
String[] parts= filteredInput.split(":");
System.out.println("First two: ");
System.out.println("--------");
for(String part:parts){
System.out.println(part);
}
System.out.println("--------");
}
indexOf
两次,找到包含您想要的内容的子字符串。split()
。 这是一种仅使用indexOf
和substring
替代解决方案。
public class Program{
public static void main(String[] args) {
String input = "1234 2345 3456 4567";
int firstIndex = input.indexOf(" ");
int secondIndex = input.indexOf(" ", firstIndex + 1);
String[] output = new String[] {input.substring(0, firstIndex),
input.substring(firstIndex+1, secondIndex)};
System.out.println(output[0]);
System.out.println(output[1]);
}
}
输出:
1234
2345
String input="1234 5678 9012 3456";
String[] result=Arrays.copyOf(input.split(" "),2);
应该管用
easiset(但不是最快的)是首先拆分字符串
String test = "123 2345 3456 4567"
String[] splitted = test.split(" ");
和切片结果数组:
String[] result = Arrays.copyOfRange(splitted, 2);
这在大多数情况下都会很好地工作。 唯一的问题可能是,当中间数组变得非常庞大时。 然后您将拥有大量中间内存,垃圾收集器必须清理这些内存。 但是使用现代优化(如逃逸分析),即使这可能不会对性能产生很大的影响。
另一个单行解决方案是:
假设你有这个:
String a="1234 2343545 356 88";
因此,单行命令将是:
String b[]=a.substring(0,a.indexOf(" ",a.indexOf(" ")+1)).split(" ");
现在你有“b”作为两个第一次出现的数组:
b[0] //1234
b[1] //2343545
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.