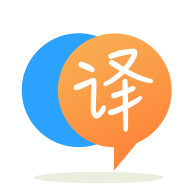
[英]Monty Hall lets make a deal Simulation in java (10000 iterations)
[英]Monty hall simulation not functioning as expected
import java.util.Scanner;
import static java.lang.System.*;
import static java.lang.Math.*;
import java.util.Random;
public class Montyhall
{
public static void main(String[] args)
{
Scanner keyboard = new Scanner(System.in);
System.out.print("Enter number of attempts:");
int attempts = keyboard.nextInt();/*Decide how many times you want this to
run. The larger the number, the more realistic an answer you will get.*/
int curtains[] = new int[3]; //like the game show, one of these will be a winner
Random rand = new Random(); /*sets the methods up with the same random seed, which
otherwise changes by the milisecond and could influence the response*/
withoutswitch(curtains, attempts, rand);
withswitch(curtains, attempts, rand);
}
public static void withoutswitch(int curtains[], int attempts, Random rand)
{
int nsCorrect=0;
for(int x=0; x < attempts; x++)
{
int winner = rand.nextInt(3);//Sets the winner to 1, leaving the other two at 0.
curtains[winner] = 1; //It then checks to see if they are the same,
int guess = rand.nextInt(3); //a 1/3 chance, roughly.
if(curtains[guess]==1){
nsCorrect++;
}
curtains[0]=0;
curtains[1]=0;
curtains[2]=0;
//the player never changes their decision, so it does not matter if a door is opened.
}
System.out.println("Number of successes with no switch: " + nsCorrect);
}
public static void withswitch(int curtains[], int attempts, Random rand)
{
int ysCorrect=0;
int goat = 0;
for(int x=0; x < attempts; x++)
{
int winner = rand.nextInt(3);
curtains[winner] = 1;
int guess = rand.nextInt(3);
goat = rand.nextInt(3);//one of the doors is opened
while(goat == winner || goat == guess)//the opened door is randomized until
goat = rand.nextInt(3); //it isn't the guess or the winner.
int guess2 = rand.nextInt(3);
while(guess2 == guess || guess2 == goat)
guess2 = rand.nextInt(3);//the second guess goes through a similar process
if(curtains[guess2]==1){
ysCorrect++;
}
curtains[0]=0;
curtains[1]=0;
curtains[2]=0;
}
System.out.println("Number of successes with a switch: " + ysCorrect);
}
}
抱歉,这有点混乱,在将近一年的中断之后,我正尝试重新进行编码。 第一部分运行正常,返回成功的几率约为1/3。 但是,第二个应该给我2/3的机会,但是我仍然能获得与没有开关时大致相同的数量。 我浏览了该网站,并发现了我不熟悉的Java之外的内容。 这个非常相似,但是似乎没有人真正帮助解决主要问题。
我该怎么做才能使赔率更现实? 清理代码的建议也将不胜感激。
编辑:代码现在起作用了,我现在正试图缩小它。
在你的方法withoutswitch
你需要改变
if(guess==1)
nsCorrect++;
至
if (curtains[guess] == 1)
nsCorrect++;
与withswitch
方法相同。 在每次运行for循环之后,您需要将curtains
重置为0。否则,先前的1
将位于其中,并且在运行几次之后, curtains
将仅包含1。
private static void resetCurtains(int[] curtains) {
for (int i = 0; i < curtains.length; i++) {
curtains[i] = 0;
}
}
在for循环中每次运行后调用该方法。
另外,即使语句是1-liner,我也建议使用{}
:
if (curtrains[guess] == 1) {
nsCorrect++;
}
您的两种方法均无法正常运行。
您的“ withoutswitch”方法仅从0、1或2中选择一个随机数,并在计数器的值为1时将其添加到计数器中。换句话说,我可以将您的“ withoutswitch”方法简化为:
public static void withoutswitch(int attempts, Random rand) {
int counter = 0;
for (int i = 0; i < attempts; i++) {
if (rand.nextInt(3) == 1) counter++;
}
System.out.println(counter);
}
不管您相信与否,您的“ withswitch”方法都会做同样的事情。 您开始使用一个变量进行猜测,然后完全忽略它,将其用于第二个变量,然后检查该变量是否为1。因此,它会产生完全相同的结果。
您的两种方法都使用“窗帘”数组,但使用不正确。 这个问题的关键是每次都将汽车放到随机的门后面,但是您永远不会将数组设置为全零,因此,经过几次运行,它就会变成全为1的数组,这绝对不是你要。
以下是一些伪代码可以帮助您入门:
number of switch wins = 0
number of stay wins = 0
scan in the number of attempts
loop through each attempt:
make an array {0, 0, 0}
pick a random number (0, 1, or 2), and set that array index to 1 (winning index)
pick a random number (0, 1, or 2), and set it to choice
pick a random number (0, 1, or 2), and set it to the shown door
loop while the door number isn't the choice number or the winning index:
pick a random number (0, 1, or 2) and set it to the shown door
increment stay wins if the choice is equal to the winning index
increment switch wins if (3 - choice - showndoor) is equal to the winning index
print stay wins and switch wins
注意:逻辑的最后一位决定已切换门的索引,因为您唯一可能的索引是0、1和2,(0 +1 + 2 = 3),然后3-您选择的门-您曾经是的门显示=最后一扇门。
希望这可以帮助!
我会备份并重组整个事情。 游戏的一个对象,两个后代,其中一个简单地捡起一扇门,其中一个简单地捡起然后切换。
我并没有完全遵循您的切换逻辑,但这绝对是错误的。 蒙蒂(Monty)的逻辑是,如果奖品在玩家的门后面,则您随机打开一只,否则用山羊打开一只。 只需要一个随机数。
同样,播放器切换。 此时只有一个窗帘,不需要随机数。
粗略了解逻辑(不是Java,不是整个程序):
MontyHaulGame
{
int[] Curtains = new int[3];
int Car = Random(3);
int Guess;
Pick();
if (Guess == Car) Wins++;
}
MontyHaulNoSwitch : MontyHaulGame
{
Pick()
{
Guess = Random(3);
}
}
MontyHaulSwitch : MontyHaulGame
{
Pick()
{
Guess = Random(3);
OpenOne();
Switch();
}
OpenOne()
{
if Guess == Car then
Repeat
Monty = Random(3);
Until Monty != Guess;
else
Monty = 1;
While (Monty == Guess) || (Monty == Car)
Monty++;
}
Switch()
{
NewGuess = 1;
While (NewGuess == Guess) || (NewGuess == Monty)
NewGuess++;
Guess == NewGuess;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.