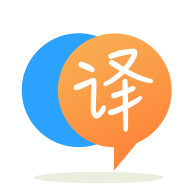
[英]Spring boot validation annotations @Valid and @NotBlank not working
[英]@Valid with spring annotations
我已经为我的项目启用了spring mvc注释驱动。 这个想法是将@Valid
注释与spring注释一起使用,以避免在控制器中出现以下行: validator.validate(form, errors)
我注意到,这些东西不适用于包中的spring注释:
org.springmodules.validation.bean.conf.loader.annotation.handle
经过调查,我发现我可以使用javax
或org.hibernate.validator.constraints
注释作为替代方法。
但是不幸的是,在某些特殊情况下,我无法实现此目标:
@MinSize(applyIf = "name NOT EQUALS 'default'", value = 1)
最好知道spring注释可以与@Valid
或其他任何替代方式一起使用,以避免与applyIf
属性相关的重构(将条件移至Java代码)。
这是如何创建自定义验证程序的示例。
首先,创建您自己的注释:
@Target({ ElementType.METHOD, ElementType.FIELD })
@Retention(RetentionPolicy.RUNTIME)
@Constraint(validatedBy = StringValidator.class)
public @interface ValidString {
String message() default "Invalid data";
int min() default -1;
int max() default -1;
String regex() default "";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
然后,您将需要一个自定义验证器:
public class StringValidator implements ConstraintValidator<ValidString, String> {
private int _min;
private int _max;
private String _regex;
private boolean _decode;
public void initialize(ValidString constraintAnnotation) {
_min = constraintAnnotation.min();
_max = constraintAnnotation.max();
_regex = constraintAnnotation.regex();
_decode = constraintAnnotation.decode();
}
public boolean isValid(String value, ConstraintValidatorContext context) {
if (value == null) {
return false;
}
String test = value.trim();
if (_min >= 0) {
if (test.length() < _min) {
return false;
}
}
if (_max > 0) {
if (test.length() > _max) {
return false;
}
}
if (_regex != null && !_regex.isEmpty()) {
if (!test.matches(_regex)) {
return false;
}
}
return true;
}
}
最后,您可以在Bean和Controller中使用它:
public class UserForm {
@ValidString(min=4, max=20, regex="^[a-z0-9]+")
private String name;
//...
}
// Method from Controller
@RequestMapping(method = RequestMethod.POST)
public String saveUser(@Valid UserForm form, BindingResult brResult) {
if (brResult.hasErrors()) {
//TODO:
}
return "somepage";
}
这样的事情可能会帮助你
public class UserValidator implements Validator {
@Override
public boolean supports(Class clazz) {
return User.class.equals(clazz);
}
@Override
public void validate(Object target, Errors errors) {
User user = (User) target;
if(user.getName() == null) {
errors.rejectValue("name", "your_error_code");
}
// do "complex" validation here
}
}
然后在您的控制器中,您将:
@RequestMapping(value="/user", method=RequestMethod.POST)
public createUser(Model model, @ModelAttribute("user") User user, BindingResult result){
UserValidator userValidator = new UserValidator();
userValidator.validate(user, result);
if (result.hasErrors()){
// do something
}
else {
// do something else
}
}
如果存在验证错误,则result.hasErrors()
将为true。
注意:您也可以使用“ binder.setValidator(...)”在控制器的@InitBinder
方法中设置验证器。 或者,您可以在控制器的默认构造函数中实例化它。 或者在控制器中插入一个@Component/@Service
UserValidato
r( @Autowired
):非常有用,因为大多数验证器都是单例+单元测试模拟变得更容易+验证器可以调用其他Spring组件。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.