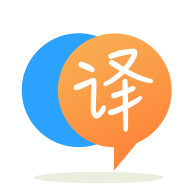
[英]How do I have a program create its own variables to match (up to an infinite) amount of user inputs?
[英]How do I have a program create its own variables with Java?
我想首先说,如果这是常识,请原谅我并有耐心。 我对Java有些新意。 我正在尝试编写一个程序,它将在一种缓冲区中存储许多变量值。 我想知道是否有办法让程序“创建”自己的变量,并将它们分配给值。 以下是我要避免的示例:
package test;
import java.util.Scanner;
public class Main {
public static void main(String args[]) {
int inputCacheNumber = 0;
//Text File:
String userInputCache1 = null;
String userInputCache2 = null;
String userInputCache3 = null;
String userInputCache4 = null;
//Program
while (true) {
Scanner scan = new Scanner(System.in);
System.out.println("User Input: ");
String userInput;
userInput = scan.nextLine();
// This would be in the text file
if (inputCacheNumber == 0) {
userInputCache1 = userInput;
inputCacheNumber++;
System.out.println(userInputCache1);
} else if (inputCacheNumber == 1) {
userInputCache2 = userInput;
inputCacheNumber++;
} else if (inputCacheNumber == 2) {
userInputCache3 = userInput;
inputCacheNumber++;
} else if (inputCacheNumber == 3) {
userInputCache4 = userInput;
inputCacheNumber++;
}
// And so on
}
}
}
所以只是为了总结一下,我想知道程序是否有办法将无限数量的用户输入值设置为String值。 我想知道是否有一种方法可以避免预定义它可能需要的所有变量。 感谢您的阅读,以及您的耐心和帮助! 〜莱恩
您可以使用Array List
数据结构。
ArrayList类扩展了AbstractList并实现了List接口。 ArrayList支持可根据需要增长的动态数组。
例如:
List<String> userInputCache = new ArrayList<>();
当你想将每个输入添加到你的数组中时
if (inputCacheNumber == 0) {
userInputCache.add(userInput); // <----- here
inputCacheNumber++;
}
如果要遍历数组列表,可以执行以下操作:
for (int i = 0; i < userInputCache.size(); i++) {
System.out.println(" your user input is " + userInputCache.get(i));
}
或者你可以使用enhanced for loop
for(String st : userInputCache) {
System.out.println("Your user input is " + st);
}
注意:最好将您的Scanner
放在try catch block with resource
这样您就不会担心它是否在最后关闭。
例如:
try(Scanner input = new Scanner(System.in)) {
/*
**whatever code you have you put here**
Good point for MadProgrammer:
Just beware of it, that's all. A lot of people have multiple stages in their
programs which may require them to create a new Scanner AFTER the try-block
*/
} catch(Exception e) {
}
有关ArrayList的更多信息
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.