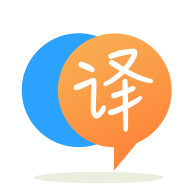
[英]How can I define an interface for an array of destructured objects inside a map function?
[英]How can I define an interface for an array of objects?
我有以下界面和代码。 我以为我在做正确的定义,但我收到一个错误:
interface IenumServiceGetOrderBy { id: number; label: string; key: any }[];
和:
getOrderBy = (entity): IenumServiceGetOrderBy => {
var result: IenumServiceGetOrderBy;
switch (entity) {
case "content":
result =
[
{ id: 0, label: 'CId', key: 'contentId' },
{ id: 1, label: 'Modified By', key: 'modifiedBy' },
{ id: 2, label: 'Modified Date', key: 'modified' },
{ id: 3, label: 'Status', key: 'contentStatusId' },
{ id: 4, label: 'Status > Type', key: ['contentStatusId', 'contentTypeId'] },
{ id: 5, label: 'Title', key: 'title' },
{ id: 6, label: 'Type', key: 'contentTypeId' },
{ id: 7, label: 'Type > Status', key: ['contentTypeId', 'contentStatusId'] }
];
break;
}
return result;
};
错误:
Error 190 Cannot convert '{}[]' to 'IenumServiceGetOrderBy':
Type '{}[]' is missing property 'id' from type 'IenumServiceGetOrderBy'
您不需要使用索引器(因为它的类型安全性稍差)。 你有两个选择:
interface EnumServiceItem {
id: number; label: string; key: any
}
interface EnumServiceItems extends Array<EnumServiceItem>{}
// Option A
var result: EnumServiceItem[] = [
{ id: 0, label: 'CId', key: 'contentId' },
{ id: 1, label: 'Modified By', key: 'modifiedBy' },
{ id: 2, label: 'Modified Date', key: 'modified' },
{ id: 3, label: 'Status', key: 'contentStatusId' },
{ id: 4, label: 'Status > Type', key: ['contentStatusId', 'contentTypeId'] },
{ id: 5, label: 'Title', key: 'title' },
{ id: 6, label: 'Type', key: 'contentTypeId' },
{ id: 7, label: 'Type > Status', key: ['contentTypeId', 'contentStatusId'] }
];
// Option B
var result: EnumServiceItems = [
{ id: 0, label: 'CId', key: 'contentId' },
{ id: 1, label: 'Modified By', key: 'modifiedBy' },
{ id: 2, label: 'Modified Date', key: 'modified' },
{ id: 3, label: 'Status', key: 'contentStatusId' },
{ id: 4, label: 'Status > Type', key: ['contentStatusId', 'contentTypeId'] },
{ id: 5, label: 'Title', key: 'title' },
{ id: 6, label: 'Type', key: 'contentTypeId' },
{ id: 7, label: 'Type > Status', key: ['contentTypeId', 'contentStatusId'] }
]
我个人推荐选项 A(使用类而不是接口时迁移更简单)。
您可以使用indexer定义接口:
interface EnumServiceGetOrderBy {
[index: number]: { id: number; label: string; key: any };
}
您可以通过简单地扩展 Array 接口将接口定义为数组。
export interface MyInterface extends Array<MyType> { }
这样,任何实现MyInterface
的对象都需要实现数组的所有函数调用,并且只能存储MyType
类型的对象。
额外的简单选项:
interface simpleInt {
id: number;
label: string;
key: any;
}
type simpleType = simpleInt[];
不使用
interface EnumServiceGetOrderBy {
[index: number]: { id: number; label: string; key: any };
}
您将收到所有 Arrays 属性和方法(例如 splice 等)的错误。
解决方案是创建一个接口,该接口定义另一个接口的数组(它将定义对象)
例如:
interface TopCategoriesProps {
data: Array<Type>;
}
interface Type {
category: string;
percentage: number;
}
像这样使用!
interface Iinput {
label: string
placeholder: string
register: any
type?: string
required: boolean
}
// This is how it can be done
const inputs: Array<Iinput> = [
{
label: "Title",
placeholder: "Bought something",
register: register,
required: true,
},
]
在 Angular 中,使用“扩展”来定义对象“数组”的接口。
使用索引器会给您一个错误,因为它不是 Array 接口,因此不包含属性和方法。
例如
错误 TS2339:类型 'ISelectOptions2' 上不存在属性 'find'。
// good
export interface ISelectOptions1 extends Array<ISelectOption> {}
// bad
export interface ISelectOptions2 {
[index: number]: ISelectOption;
}
interface ISelectOption {
prop1: string;
prop2?: boolean;
}
如果您不想创建全新的类型,这是一个内联版本:
export interface ISomeInterface extends Array<{
[someindex: string]: number;
}> { };
没有 tslint 错误的简单选项...
export interface MyItem {
id: number
name: string
}
export type MyItemList = [MyItem]
你也可以这样做。
interface IenumServiceGetOrderBy {
id: number;
label: string;
key: any;
}
// notice i am not using the []
var oneResult: IenumServiceGetOrderBy = { id: 0, label: 'CId', key: 'contentId'};
//notice i am using []
// it is read like "array of IenumServiceGetOrderBy"
var ArrayOfResult: IenumServiceGetOrderBy[] =
[
{ id: 0, label: 'CId', key: 'contentId' },
{ id: 1, label: 'Modified By', key: 'modifiedBy' },
{ id: 2, label: 'Modified Date', key: 'modified' },
{ id: 3, label: 'Status', key: 'contentStatusId' },
{ id: 4, label: 'Status > Type', key: ['contentStatusId', 'contentTypeId'] },
{ id: 5, label: 'Title', key: 'title' },
{ id: 6, label: 'Type', key: 'contentTypeId' },
{ id: 7, label: 'Type > Status', key: ['contentTypeId', 'contentStatusId'] }
];
编程很简单。 使用简单的用例:
interface IenumServiceGetOrderBy { id: number; label: string; key: any }
// OR
interface IenumServiceGetOrderBy { id: number; label: string; key: string | string[] }
// use interface like
const result: IenumServiceGetOrderBy[] =
[
{ id: 0, label: 'CId', key: 'contentId' },
{ id: 1, label: 'Modified By', key: 'modifiedBy' },
{ id: 4, label: 'Status > Type', key: ['contentStatusId', 'contentTypeId'] }
];
这是适合您的示例的一种解决方案:
interface IenumServiceGetOrderByAttributes {
id: number;
label: string;
key: any
}
interface IenumServiceGetOrderBy extends Array<IenumServiceGetOrderByAttributes> {
}
let result: IenumServiceGetOrderBy;
使用此解决方案,您可以使用 Array 的所有属性和方法(例如: length, push(), pop(), splice()
...)
我会使用以下结构:
interface arrayOfObjects extends Array<{}> {}
然后更容易定义:
let myArrayOfObjects: arrayOfObjects = [
{ id: 0, label: "CId", key: "contentId" },
{ id: 1, label: "Modified By", key: "modifiedBy" },
{ id: 2, label: "Modified Date", key: "modified" },
{ id: 3, label: "Status", key: "contentStatusId" },
{ id: 4, label: "Status > Type", key: ["contentStatusId", "contentTypeId"] },
{ id: 5, label: "Title", key: "title" },
{ id: 6, label: "Type", key: "contentTypeId" },
{ id: 7, label: "Type > Status", key: ["contentTypeId", "contentStatusId"] },
];
假设您想要一个充满Person
的Workplace
类型。 你需要这样的东西:
interface Workplace {
name: string
age: number
}[]
// that [] doesn't work!! ;)
这里的问题是接口用于指定类/对象形状..仅此而已。 所以你可以使用type
而不是 witch 更灵活
type
而不是interface
type Workplace = {
name: string
age: number
}[]
// This works :D
interface
定义内部对象Person
,然后将Workplace
定义为由人员数组创建的type
-> Person[]
interface Person {
name: string
age: number
}
type Workplace = Person[]
// nice ;)
您可以通过简单地扩展接口将类型定义为对象数组。 下面是一个例子:
// type of each item in the Service list
interface EnumServiceItem {
id: string;
label: string;
}
// type of the Service
interface ServiceType {
id: string,
label: string,
childList?: Array<EnumServiceItem>
}
// type of the Service list
type ServiceListType = Array<ServiceType>
let draggableList:ServiceListType = [
{
id: "1",
label: 'Parent Item 1',
childList: [
{
id: "11",
label: 'Child Item 1',
},
{
id: "12",
label: 'Child Item 2',
}
,
{
id: "13",
label: 'Child Item 3',
}
]
},
{
id: "2",
label: 'Parent Item 2',
childList: [
{
id: "14",
label: 'Child Item 4',
},
{
id: "15",
label: 'Child Item 5',
}
,
{
id: "16",
label: 'Child Item 6',
}
]
},
{
id: "3",
label: 'Parent Item 3',
childList: [
{
id: "17",
label: 'Child Item 7',
},
{
id: "18",
label: 'Child Item 8',
}
,
{
id: "19",
label: 'Child Item 9',
}
]
},
]
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.