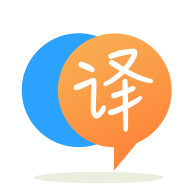
[英]Trying to workout how to use quaternions to rotate a camera that is moving along a path to look in new direction vector
[英]Trying to workout how to deal with an array
我正在自学JavaScript,尽管我遇到了障碍,但它仍在研究中。 作为练习,我试图构建一个税收计算器。
这是我构建的包含税项的对象:
var taxBracket = [
{bracket: 1, from:0, to:18200, percentage :0, amount:0},
{bracket: 2, from:18201, to:37000, percentage :19, over:18200, amount:0},
{bracket: 3, from:37001, to:80000, percentage :32.5, over:37000, amount:3752},
{bracket: 4, from:80001, to:180000, percentage :37, over:80000, amount:17547},
{bracket: 5, from:180001, to:0, percentage :45, over:180000, amount:54547}];
这是遍历对象以查找y的相应税级的函数
function returnTax (y){
for(var x in taxBracket){
if (y >= taxBracket[x].from && y <= taxBracket[x].to){
var z = taxBracket[x].amount + ((grossIncome-taxBracket[x].over) * (taxBracket[x].percentage/100));
return z;
}
};
问题是,如果y
超过18万,它的错误了作为to
0这是唯一的解决方案,以这样的一个else语句重复if语句的功能是什么? 谢谢您帮忙!
到目前为止,做得很好。 只需使用Infinity而不是最后一个括号中的默认值即可解决此问题。 我也对您的语法提出了一些建议,希望对您有所帮助。
var taxBracket = [
{bracket: 1, from: 0, to: 18200, percentage: 0, amount: 0},
{bracket: 2, from: 18201, to: 37000, percentage: 19, over: 18200, amount: 0},
{bracket: 3, from: 37001, to: 80000, percentage: 32.5, over: 37000, amount: 3752},
{bracket: 4, from: 80001, to: 180000, percentage: 37, over: 80000, amount: 17547},
// Use Infinity instead of 0 for the "to" value of bracket 5
{bracket: 5, from: 180001, to: Infinity, percentage: 45, over: 180000, amount: 54547}
];
// Variable names like 'x', 'y' and 'z' can cause problems with code readability.
// Descriptive names such as "income", or even "string" or "int" will help you out when you come to review your code later!
function returnTax(y){
// Using for..in with arrays can cause issues with enumerating over object properties.
// You don't have to worry about it in this case, but a standard for loop (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/for) is better practice.
for(var x = 0; x < taxBracket.length; x++){
if(y <= taxBracket[x].to){
// I find breaking up long calculations into separate variables can make it
// more readable. More preference than anything else though!
var amountOver = grossIncome - taxBracket[x].over;
var percent = taxBracket[x].percentage / 100;
return taxBracket[x].amount + (amountOver * percent);
}
}
}
您正在创建对象文字的数组文字。 您应该使用标准的for循环遍历数组。
一种解决方案是使最后一个“ to”字段的数量非常大。
您可以使用内置函数来循环对象数组
yourArray.forEach
( function (arrayItem)
{
var x =
arrayItem.prop1 + 2;
alert(x);
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.