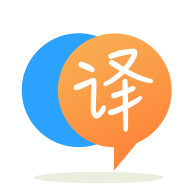
[英]Sorting hierarchical data in Javascript (Adjacency List/Flat table)
[英]Hierarchical Sorting of Categories in Javascript
我试图以一种分层的方式对下面给出的类别进行排序,然后将它们输出到网页上。 对于一个事实,我知道我需要使用某种形式的递归,因为类别可以具有任何深度。 我还希望能够按字母顺序在相同深度级别上对这些类别进行排序,我知道我可以使用someArray.sort()
方法来进行someArray.sort()
。
理想情况下,我将具有类似这样的结构,每个名称都是树中的一个对象,并且term_id是其在数组中的索引:
0 Computer Technology
0 --> Hardware
0 ----> Microprocessors
0 ----> Hard Drives
0 --> Software
0 ----> Firmware & Embedded Systems
0 ----> Operating Systems
0 ----> Web and Internet-Based Applications
0 Uncategorized
到目前为止,我已经浏览了网站上的许多问题,但仍无法根据我的需要明确地解决其中的任何一个问题。 到目前为止,这是我到达的地方:
function hierarchicalSort( categories, sorted, parentID ) {
for( var i = 0; i < categories.length; i++ ) {
if( categories[i] === null )
{
continue; // There may be more categories to sort
}
else if( categories[i].parent == parentID ) {
sorted.push( categories[i] ); // Same parent ( same level )
categories[i] = null; // Our "Already Sorted" flag
}
else {
sorted[categories[i].term_id].children = [];
// This category has a different parent
hierarchicalSort( categories, sorted, categories[i].term_id );
// Find this category's children
}
}
}
我的类别包含以下信息:
var categories = [
{
term_id: 1,
name: Uncategorized,
parent: 0,
count: 1
},
{
term_id: 2,
name: Hardware,
parent: 7,
count: 1
},
{
term_id: 3,
name: Software,
parent: 7,
count: 2
},
{
term_id: 7,
name: Computer Tech,
parent: 0,
count: 0
},
{
term_id: 8,
name: Operating Systems,
parent: 3,
count: 1
},
{
term_id: 9,
name: Firmware and Embedded Systems,
parent: 3,
count: 0
},
{
term_id: 10,
name: Web and Internet-Based Applications,
parent: 3,
count: 0
},
{
term_id: 11,
name: Hard Drives,
parent: 7,
count: 3
},
{
term_id: 23,
name: Microprocessors,
parent: 7,
count: 1
}
];
我建议的解决方案:
将类别数组用作队列,并在while循环运行时检查每个类别。
/**
* Creates a category tree structure from a list of objects.
*
* @param {array} categories: The list of categories to sort into a hierarchical tree.
* It is mainly accessed as a queue to ensure that no category is missed during each
* generation of the tree level.
*
* @param {array} sorted: The "treeified" structure of categories
* @param {int} parentID: The ID of the parent category
*
* @since 0.2.0
*
* @returns {undefined}
*/
function hierarchicalSort( categories, sorted, parentID )
{
/**
* Term Iterator var i: Goal is to go through each category at least once
*/
var i = categories.length;
// This loop completes a full cycle through categories for each depth level
while( --i >= 0 ) {
// Pull the category out from the sorting "queue"
var category = categories.shift();
if( category.parent == parentID ) {
sorted.push( category ); // Same parent
}
else {
categories.push( category ); // Uhh, this category has a different parent...
// Put it back on the queue for sorting at a later time
}
}
// Keep going until you hit the end of the array
for( var j = 0; typeof sorted[j] !== 'undefined'; j++ ) {
sorted[j].children = [];
/**
* Find this category's children, by importing the remaining queue,
* giving the current sorted category's children property, and the
* current sorted category's term_id
*/
hierarchicalSort( categories, sorted[j].children, sorted[j].term_id );
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.