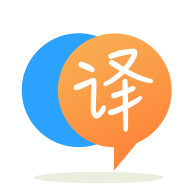
[英]Play/Ebean not fetching all attributes of ManyToOne related object
[英]Ebean @ManyToOne, finder doesn't retrieve all data of related object
我正在使用Ebean进行对象映射,并且我已经创建了我的SQL表
create table company (
id int auto_increment not null primary key,
name varchar(100)
);
create table employee (
id int auto_increment not null primary key,
name varchar(100) not null,
company_id int not null,
constraint foreign key (company_id) references company (id)
on delete restrict on update restrict
);
这是Ebean公司的模型
import javax.persistence.Entity;
import javax.persistence.Id;
import com.avaje.ebean.Model;
@Entity
public class Company extends Model
{
@Id
public Integer id;
public String name;
}
和员工模型
@Entity
public class Employee extends Model
{
@Id
public Integer id;
public String name;
@ManyToOne
public Company company;
public static Finder<Long, Employee> find = new Finder<Long, Employee>(Long.class, Employee.class);
}
当我运行以下
Company company = new Company();
company.name = "Microsoft";
company.save();
Employee employee = new Employee();
employee.name = "Mr John";
employee.company = company;
employee.save();
Employee mrJohn = Employee.find.where().eq("id", 1).findUnique();
System.out.println(mrJohn.company.id);
System.out.println(mrJohn.company.name);
第一个System.out.println给出1(这是分配给员工的公司的正确ID),但第二个显示为null(我预期应该具有值“Microsoft”),输出为
1
null
因此,问题是为什么只检索公司模型的ID,而不检索其他相关数据?
您可以使用fetch()来急切地获取图形的其他部分。 在这种情况下,获取公司名称,如:
Employee.find.fetch( “公司”, “名”),其中()EQ( “ID”,1).findUnique()。
简而言之,无法拦截现场访问(除非您增强调用者)。 因此,对company.name使用字段访问意味着customer.name是一个GETFIELD操作,并且它没有被Ebean拦截,因此没有调用延迟加载(因此返回了null)。
更改为使用getter / setter意味着在调用customer.getName()时调用延迟加载。
Java不支持属性(有getter和setter)。 您可以查看其他类似Groovy和Kotlin的JVM语言。
Groovy支持属性,使用带有@CompileStatic的Groovy的示例是: https : //github.com/ebean-orm/avaje-ebeanorm-examples/blob/master/e-groovy https://github.com/ebean-orm/ avaje-ebeanorm-实例/斑点/主/电子常规/ SRC /主/常规/组织/示例/域/ Customer.groovy
Kotlin支持属性,例如: https : //github.com/ebean-orm/avaje-ebeanorm-examples/tree/master/e-kotlin-maven
干杯,罗布。
我今天遇到了这个问题,并且我发现了当我们访问公共变量时懒惰地取消它的原因。
可以通过调用属性的getter
方法来调用lazily。 所以我需要使用getter
方法在我的模型获取数据中创建getter
。
@Entity
public class Company extends Model
{
@Id
public Integer id;
public String name;
public Integer getId() {
return id;
}
public String getName() {
return name;
}
}
然后取公司:
System.out.println(mrJohn.company.getName());
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.