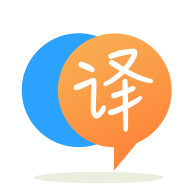
[英]How to reference a system property within a user-defined .properties file?
[英]How to retrieve a user-defined type from a for loop?
我定义了一个Attribute
类型,并且有一个Vec<Attribute>
,我正在遍历该Vec<Attribute>
来检索“最佳”类型。 这类似于我的第一次尝试:
#[derive(Debug)]
struct Attribute;
impl Attribute {
fn new() -> Self {
Self
}
}
fn example(attrs: Vec<Attribute>, root: &mut Attribute) {
let mut best_attr = &Attribute::new();
for a in attrs.iter() {
if is_best(a) {
best_attr = a;
}
}
*root = *best_attr;
}
// simplified for example
fn is_best(_: &Attribute) -> bool {
true
}
我遇到以下编译错误:
error[E0507]: cannot move out of borrowed content
--> src/lib.rs:17:13
|
17 | *root = *best_attr;
| ^^^^^^^^^^ cannot move out of borrowed content
在寻找解决方案后,我通过执行以下操作解决了该错误:
Attribute
结构中添加#[derive(Clone)]
属性 *root = best_attr.clone();
代替最终语句*root = best_attr.clone();
我不完全理解为什么这样做,我觉得这是我遇到的问题的粗略解决方案。 这如何解决错误,这是解决此问题的正确方法吗?
您正在体验Rust内存模型的基础:
Copy
类型) 以下面的代码为例:
let x = String::new();
let y = x;
println!("{}", x);
它产生错误:
error[E0382]: borrow of moved value: `x`
--> src/main.rs:4:20
|
3 | let y = x;
| - value moved here
4 | println!("{}", x);
| ^ value borrowed here after move
|
= note: move occurs because `x` has type `std::string::String`, which does not implement the `Copy` trait
类型为String
x
不可隐式复制,因此已移入 y
。 x
不能再使用。
在代码中,当您编写*root = *best_attr
,首先要取消引用best_attr
的引用,然后将已取消引用的值分配给*root
。 您的Attribute
类型不是Copy
,因此此分配应该是可移动的 。
然后,编译器抱怨:
cannot move out of borrowed content
事实上, best_attr
是一个不变的参考,它不允许你把它背后的价值的所有权(它甚至不允许修改它)。 允许这样的移动会使拥有值的对象处于未定义状态的引用后面,这正是Rust旨在避免的情况。
在这种情况下,最好的选择确实是创建一个与第一个对象具有相同值的新对象,这正是Clone
特性的用途。
#[derive(Clone)]
允许您将结构标记为可Clone
,只要它们的所有字段均为Clone
。 在更复杂的情况下,您必须手动实现该特征。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.