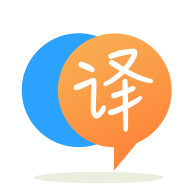
[英]java.net.SocketException: Connection reset when trying to pass an object from a client to a server
[英]Receiving “java.net.SocketException: Connection reset” when connecting from dotnet client to a java server
我有一台服务器(用Java编写),用于侦听特定端口上的连接。 我有一个与Java服务器连接的客户端(用C#编写),尝试发送一些数据,但连接重新设置,服务器端出现以下错误。 “ java.net.SocketException:连接重置”
以下是客户端代码:-
public void ConnectServer()
{
try
{
if (Connect())
{
Broadcast("check");
}
}
catch (Exception ex)
{
Logger.Text = ex.Message;
}
}
private bool Connect()
{
bool flag = false;
try
{
if (!clientSocket.Connected)
{
clientSocket.Connect(ConfigurationManager.AppSettings["SMSRequestNotifierServer"].ToString(), Convert.ToInt32(ConfigurationManager.AppSettings["SMSRequestNotifierPort"].ToString()));
clientSocket.LingerState = new LingerOption(true,10);
isConnected = true;
flag = true;
}
else
{
flag = true;
}
}
catch (Exception ex)
{
Logger.Text = ex.Message;
flag = false; }
return flag;
}
private void Broadcast(String msg)
{
using (NetworkStream serverStream = clientSocket.GetStream())
{
byte[] outStream = System.Text.Encoding.ASCII.GetBytes(msg);
serverStream.Write(outStream, 0, outStream.Length);
serverStream.Flush();
serverStream.Close();
serverStream.Dispose();
clientSocket.Close();
}
}
谁能指出我在此代码中做错了什么,我的连接已重置?
在代码中的另一个发现是,当我调试此行时,它应该将数据写入流中,服务器应该接收它,但是什么也没发生:
serverStream.Write(outStream, 0, outStream.Length);
执行以下行将在服务器端引发“ java.net.SocketException:连接重置”。
serverStream.Flush();
serverStream.Close();
发送时而不是连接时出现此错误。 当您发送到已被对等方关闭的连接时,就会发生这种情况。
例如:
要么
检查应用程序协议。
最初的问题是在My Dotnet Client代码中,套接字在建立后立即关闭。 为此,我使用了http://www.codeproject.com/Articles/19071/Quick-tool-A-minimalistic-Telnet-library
下面是解决我的问题的代码
TelnetConnection oTelnetConnection = new TelnetConnection(ConfigurationManager.AppSettings["SMSRequestNotifierServer"].ToString(), Convert.ToInt32(ConfigurationManager.AppSettings["SMSRequestNotifierPort"].ToString()));
Logger.Text += oTelnetConnection.Read();
oTelnetConnection.WriteLine("check");
// minimalistic telnet implementation
// conceived by Tom Janssens on 2007/06/06 for codeproject
//
// http://www.corebvba.be
using System;
using System.Collections.Generic;
using System.Text;
using System.Net.Sockets;
namespace MinimalisticTelnet
{
enum Verbs {
WILL = 251,
WONT = 252,
DO = 253,
DONT = 254,
IAC = 255
}
enum Options
{
SGA = 3
}
class TelnetConnection
{
TcpClient tcpSocket;
int TimeOutMs = 100;
public TelnetConnection(string Hostname, int Port)
{
tcpSocket = new TcpClient(Hostname, Port);
}
public string Login(string Username,string Password,int LoginTimeOutMs)
{
int oldTimeOutMs = TimeOutMs;
TimeOutMs = LoginTimeOutMs;
string s = Read();
if (!s.TrimEnd().EndsWith(":"))
throw new Exception("Failed to connect : no login prompt");
WriteLine(Username);
s += Read();
if (!s.TrimEnd().EndsWith(":"))
throw new Exception("Failed to connect : no password prompt");
WriteLine(Password);
s += Read();
TimeOutMs = oldTimeOutMs;
return s;
}
public void WriteLine(string cmd)
{
Write(cmd + "\n");
}
public void Write(string cmd)
{
if (!tcpSocket.Connected) return;
byte[] buf = System.Text.ASCIIEncoding.ASCII.GetBytes(cmd.Replace("\0xFF","\0xFF\0xFF"));
tcpSocket.GetStream().Write(buf, 0, buf.Length);
}
public string Read()
{
if (!tcpSocket.Connected) return null;
StringBuilder sb=new StringBuilder();
do
{
ParseTelnet(sb);
System.Threading.Thread.Sleep(TimeOutMs);
} while (tcpSocket.Available > 0);
return sb.ToString();
}
public bool IsConnected
{
get { return tcpSocket.Connected; }
}
void ParseTelnet(StringBuilder sb)
{
while (tcpSocket.Available > 0)
{
int input = tcpSocket.GetStream().ReadByte();
switch (input)
{
case -1 :
break;
case (int)Verbs.IAC:
// interpret as command
int inputverb = tcpSocket.GetStream().ReadByte();
if (inputverb == -1) break;
switch (inputverb)
{
case (int)Verbs.IAC:
//literal IAC = 255 escaped, so append char 255 to string
sb.Append(inputverb);
break;
case (int)Verbs.DO:
case (int)Verbs.DONT:
case (int)Verbs.WILL:
case (int)Verbs.WONT:
// reply to all commands with "WONT", unless it is SGA (suppres go ahead)
int inputoption = tcpSocket.GetStream().ReadByte();
if (inputoption == -1) break;
tcpSocket.GetStream().WriteByte((byte)Verbs.IAC);
if (inputoption == (int)Options.SGA )
tcpSocket.GetStream().WriteByte(inputverb == (int)Verbs.DO ? (byte)Verbs.WILL:(byte)Verbs.DO);
else
tcpSocket.GetStream().WriteByte(inputverb == (int)Verbs.DO ? (byte)Verbs.WONT : (byte)Verbs.DONT);
tcpSocket.GetStream().WriteByte((byte)inputoption);
break;
default:
break;
}
break;
default:
sb.Append( (char)input );
break;
}
}
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.