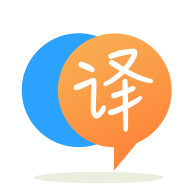
[英]Change color of line segment in HTML5 Canvas with jQuery Colorpicker plugin
[英]select and change color of a line in html5 canvas?
我写了这段代码来绘制随机图。 我一直试图找到如何在图中选择一条线以便我可以应用prim的算法,因为我选择了一行并查看它们是否找到了最小的树。
function draw(n,rep){
var cvs=document.getElementsByTagName('canvas')[0];
/**
* @type CanvasRenderingContext2D
**/
var ctx=cvs.getContext('2d');
ctx.beginPath();
var randomX=[];
var randomY=[];
ctx.lineWidth=2;
ctx.font = '3'+' Arial';
var weights=[];
var lastRandomx=Math.random()*200;
var lastRandomy=Math.random()*200;
for (var i = 0; i <n ; i++) {
var cwidth = cvs.width;
var cheight = cvs.height;
randomX[i] = Math.random()*cwidth*2/3;
randomY[i] = Math.random()*cheight*2/3;
weights[i]=Math.round(Math.random()*20);
ctx.fillRect(randomX[i],randomY[i],5,5);
ctx.moveTo(lastRandomx,lastRandomy);
ctx.lineTo(randomX[i],randomY[i]);
lastRandomx=randomX[i];
lastRandomy=randomY[i];
}
for (var i = 0; i < rep; i++) {
var rand=Math.round(rep*Math.random());
ctx.lineTo(randomX[rand],randomY[rand]);
}
ctx.closePath();
ctx.stroke();
};
我在stackoverflow中发现了这个并没有多大帮助。 如何选择在HTML5 Canvas上绘制的线条? 。 我想知道是否有预编写的代码,所以我不需要从头开始编写代码。
我在想是否可以在移动时找到鼠标的位置,每次检查鼠标的位置是否在线上,如此处查找点是否在一条线上 。 请帮忙并建议是否有任何预先编写的代码,因为我受到时间的限制。 先感谢您。
你必须遍历你的线阵列,并为每个线段做:
核心原则是在路径中添加一行,然后测试if(x,y)是否在该行上:
ctx.beginPath();
ctx.moveTo(x1, y1); // start of line
ctx.lineTo(x2, y2); // end of line
// this will test the point against the line (lineWidth matters)
if (ctx.isPointInStroke(x, y)) {
// draw line segment in f.ex. different color here
ctx.strokeStyle = "red";
ctx.stroke(); // we already have a line segment on the path
}
没有必要实际划线,只需重建路径。 根据需要采用。
这是一个完整的例子:
var ctx = canvas.getContext("2d"), lines = [], // store line segments for demo count = 10, // max 10 lines for demo i = 0; for(; i < count; i++) { var x = Math.random() * canvas.width; // random point for end points var y = Math.random() * canvas.height; if (i) ctx.lineTo(x, y); // if not first line, add lineTo else ctx.moveTo(x, y); // start point lines.push({ // store point to create a poly-line x: x, y: y }); } ctx.lineWidth = 5; ctx.lineJoin = "round"; ctx.strokeStyle = "blue"; ctx.stroke(); // ..and draw line // here we use the principle canvas.onclick = function(e) { var r = canvas.getBoundingClientRect(), // adjust to proper mouse position x = e.clientX - r.left, y = e.clientY - r.top, i = 0 // for each line segment, build segment to path and check for(; i < count - 1; i++) { ctx.beginPath(); // new segment ctx.moveTo(lines[i].x, lines[i].y); // start is current point ctx.lineTo(lines[i+1].x, lines[i+1].y); // end point is next if (ctx.isPointInStroke(x, y)) { // x,y is on line? ctx.strokeStyle = "red"; // stroke red for demo ctx.stroke(); break; } } }
<canvas id=canvas width=500 height=500></canvas>
要提高灵敏度,可以将lineWidth
调整为更大的值(无需重绘)。
您可以使用数学来确定哪条线最接近鼠标。
这是示例代码和演示:
// canvas related variables var canvas=document.getElementById("canvas"); var ctx=canvas.getContext("2d"); var cw=canvas.width; var ch=canvas.height; var $canvas=$("#canvas"); var canvasOffset=$canvas.offset(); var offsetX=canvasOffset.left; var offsetY=canvasOffset.top; ctx.lineWidth=2; // linear interpolation -- needed in setClosestLine() var lerp=function(a,b,x){ return(a+x*(ba)); }; // vars to track which line is closest to the mouse var closestLineIndex=-1; var closestX,closestY; // make some random lines and save them in lines[] var n=5; var lines=[]; var randomX=function(){return(Math.random()*cw*.67);} var randomY=function(){return(Math.random()*ch*.67);} var lastX=randomX(); var lastY=randomY(); for(var i=0;i<n;i++){ var x=Math.random()*cw*.67; var y=Math.random()*ch*.67; var dx=x-lastX; var dy=y-lastY; var line={ x0:lastX, y0:lastY, x1:x, y1:y, weight:Math.round(Math.random()*20), // precalc often used values dx:dx, dy:dy, dx2dy2:dx*dx+dy*dy, }; lines.push(line); lastX=x; lastY=y; } redraw(); $("#canvas").mousedown(function(e){handleMouseDown(e);}); $("#canvas").mousemove(function(e){handleMouseMove(e);}); ////////////////////////////// function setClosestLine(mx,my) { closestLineIndex=-1; var minDistanceSquared=100000000; // examine each line & // determine which line is closest to the mouse (mx,my) for(var i=0;i<lines.length;i++){ var line=lines[i]; var dx=line.x1-line.x0; var dy=line.y1-line.y0; var t=((mx-line.x0)*line.dx+(my-line.y0)*line.dy)/line.dx2dy2; var x=lerp(line.x0, line.x1, t); var y=lerp(line.y0, line.y1, t); var dx1=mx-x; var dy1=my-y; var distSquared=dx1*dx1+dy1*dy1; if(distSquared<minDistanceSquared){ minDistanceSquared=distSquared; closestLineIndex=i; closestX=x; closestY=y; } } }; function redraw(){ // clear the canvas ctx.clearRect(0,0,cw,ch); // draw all lines ctx.strokeStyle='black'; for(var i=0;i<lines.length;i++){ var line=lines[i]; ctx.beginPath(); ctx.moveTo(line.x0,line.y0); ctx.lineTo(line.x1,line.y1); ctx.stroke(); } // draw the line closest to the mouse in red if(closestLineIndex<0){return;} var line=lines[closestLineIndex]; ctx.strokeStyle='red'; ctx.beginPath(); ctx.moveTo(line.x0,line.y0); ctx.lineTo(line.x1,line.y1); ctx.stroke(); ctx.fillText("Index:"+closestLineIndex+", weight:"+line.weight,10,15); } function handleMouseMove(e){ e.preventDefault(); e.stopPropagation(); mouseX=parseInt(e.clientX-offsetX); mouseY=parseInt(e.clientY-offsetY); setClosestLine(mouseX,mouseY); redraw(); }
body{ background-color: ivory; } #canvas{border:1px solid red;}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script> <h4>Closest line is drawn in red<br>Closest line's weight is reported top-left</h4> <canvas id="canvas" width=300 height=300></canvas>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.