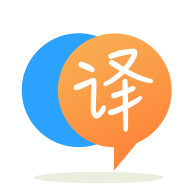
[英]How to save this image to MySQL database using HIbernate as BLOB?
[英]How to save images to mysql database using Hibernate
我正在做一个需要上传大量图像的项目,但是我不知道图像上传的实际过程。 我正在使用没有Spring MVC的Hibernate 3。 所有页面都是JSP页面。 主要问题是如何从请求中获取图像并将其保存到数据库。 我已经解析了请求并获取了所有参数值,这些值是表单字段。 我应该为图像文件做什么??
要将图像保存到数据库中,您需要将表列定义为MySQL中的blob数据类型,或其他数据库中的等效二进制类型。 在Hibernate端,您可以声明一个字节数组变量来存储图像数据。
首先,您需要下载以下jar文件:-
1)commons-fileupload-1.3.3
2)commons-fileupload-1.3.3-javadoc
3)commons-fileupload-1.3.3-源
4)commons-fileupload-1.3.3-测试
5)commons-fileupload-1.3.3-test-sources
6)commons-io-2.5
7)commons-io-2.5-javadoc
和休眠jar文件
您还必须设置项目的路径以在Web浏览器上显示图像:-
右键单击项目->属性->复制完整位置->打开web.xml
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<display-name>imghib</display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<context-param>
<description>Location to store uploaded file</description>
<param-name>file-upload</param-name>
<param-value>
C:\Users\mohammed lala\mdlala\imghib\webContent\
</param-value>
</context-param>
</web-app>
index.html
<html>
<head>
<title>File Uploading Form</title>
</head>
<body>
<h3>File Upload:</h3>
Select a file to upload: <br />
<form action = "UploadServlet.jsp" method = "post"
enctype = "multipart/form-data">
<input type = "file" name = "file" size = "50" />
<br />
<input type = "submit" value = "Upload File" />
</form>
</body>
</html>
UploadServlet.jsp
<%@page import="org.hibernate.cfg.AnnotationConfiguration"%>
<%@page import="img.Image"%>
<%@page import="org.hibernate.Transaction"%>
<%@page import="org.hibernate.Session"%>
<%@ page import = "java.io.*,java.util.*, javax.servlet.*" %>
<%@ page import = "javax.servlet.http.*" %>
<%@ page import = "org.apache.commons.fileupload.*" %>
<%@ page import = "org.apache.commons.fileupload.disk.*" %>
<%@ page import = "org.apache.commons.fileupload.servlet.*" %>
<%@ page import = "org.apache.commons.io.output.*" %>
<%
Session s=new AnnotationConfiguration().configure().buildSessionFactory().openSession();
Transaction tx=s.beginTransaction();
File file ;
int maxFileSize = 5000 * 1024;
int maxMemSize = 5000 * 1024;
ServletContext context = pageContext.getServletContext();
String filePath = context.getInitParameter("file-upload");
// Verify the content type
String contentType = request.getContentType();
if ((contentType.indexOf("multipart/form-data") >= 0)) {
DiskFileItemFactory factory = new DiskFileItemFactory();
// maximum size that will be stored in memory
factory.setSizeThreshold(maxMemSize);
// Location to save data that is larger than maxMemSize.
factory.setRepository(new File("c:\\temp"));
// Create a new file upload handler
ServletFileUpload upload = new ServletFileUpload(factory);
// maximum file size to be uploaded.
upload.setSizeMax( maxFileSize );
try {
// Parse the request to get file items.
List fileItems = upload.parseRequest(request);
// Process the uploaded file items
Iterator i = fileItems.iterator();
out.println("<html>");
out.println("<head>");
out.println("<title>JSP File upload</title>");
out.println("</head>");
out.println("<body>");
while ( i.hasNext () ) {
FileItem fi = (FileItem)i.next();
if ( !fi.isFormField () ) {
// Get the uploaded file parameters
String fieldName = fi.getFieldName();
String fileName = fi.getName();
boolean isInMemory = fi.isInMemory();
long sizeInBytes = fi.getSize();
// Write the file
if( fileName.lastIndexOf("\\") >= 0 ) {
file = new File( filePath +
fileName.substring( fileName.lastIndexOf("\\"))) ;
} else {
file = new File( filePath +
fileName.substring(fileName.lastIndexOf("\\")+1)) ;
}
fi.write( file ) ;
out.println("Uploaded Filename: " + filePath +
fileName + "<br>");
Image ig=new Image();
ig.setFileName(fileName);
s.saveOrUpdate(ig);
tx.commit();
s.close();
response.sendRedirect("show.jsp?name="+fileName+" ");
}
}
out.println("</body>");
out.println("</html>");
} catch(Exception ex) {
System.out.println(ex);
}
} else {
out.println("<html>");
out.println("<head>");
out.println("<title>Servlet upload</title>");
out.println("</head>");
out.println("<body>");
out.println("<p>No file uploaded</p>");
out.println("</body>");
out.println("</html>");
}
%>
show.jsp
<%
String name=request.getParameter("name");
out.print(name);
%>
<img src="<%=name %>">
图像.java
package img;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name="image")
public class Image {
@Id
@GeneratedValue
private int id;
private String fileName;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFileName() {
return fileName;
}
public void setFileName(String fileName) {
this.fileName = fileName;
}
}
hibernate.cfg.xml
<?xml version='1.0' encoding='UTF-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="connection.url">
jdbc:mysql://localhost:3306/db_name
</property>
<property name="connection.username">root</property>
<property name="connection.password">scott</property>
<property name="connection.driver_class">
com.mysql.jdbc.Driver
</property>
<property name="dialect">
org.hibernate.dialect.MySQLDialect
</property>
<property name="hbm2ddl.auto">update</property>
<mapping class="img.Image"></mapping>
</session-factory>
</hibernate-configuration>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.