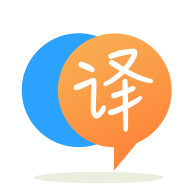
[英]How to write a test case for Jersey Rest resouce + Grizzly+mockito
[英]Mocking a Jersey REST Api running on Grizzly using Mockito
我正在测试我使用Jersey设置的RESTful api。 我想用JUnit测试它,同时用Grizzly运行它以提供Http容器。
使用服务器的一般测试工作正常,即发送请求和接收响应等。
api被称为CMSApi,它有一个名为skyService的依赖项,我想模拟出来,所以我只测试了api。 所以问题是我如何使用Mockito创建的mockSkyService对象注入CMSApi? 相关代码如下:
Grizzly Server启动:
public static HttpServer startServer() {
final ResourceConfig rc = new ResourceConfig().packages("com.sky");
rc.property(ServerProperties.BV_SEND_ERROR_IN_RESPONSE, true);
return GrizzlyHttpServerFactory.createHttpServer(URI.create(BASE_URI), rc);
}
我的JUnit调用上面的start方法:
@Before
public void setUpServer() throws Exception {
// start the server and create the client
server = Main.startServer();
Client client = ClientBuilder.newClient();
target = client.target(Main.BASE_URI);
}
我使用@RunWith(MockitoJUnitRunner.class)
运行测试。
以下是测试中的相关对象:
//System Under Test
private CMSApi cmsApi;
//Mock
@Mock private static SkyService mockSkyService;
这是调用的测试方法:
@Test
public void testSaveTile() {
//given
final Invocation.Builder invocationBuilder = target.path(PATH_TILE).request(MediaType.APPLICATION_JSON).accept(MediaType.APPLICATION_JSON);
Tile tile = new Tile(8, "LABEL", clientId, new Date(), null);
//when
Response response = invocationBuilder.post(Entity.entity(tile, MediaType.APPLICATION_JSON_TYPE));
//then
assertEquals(Status.OK.getStatusCode(), response.getStatus());
}
Maven依赖
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.sky</groupId>
<artifactId>sky-service</artifactId>
<packaging>jar</packaging>
<version>1.0-SNAPSHOT</version>
<name>sky-service</name>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.glassfish.jersey</groupId>
<artifactId>jersey-bom</artifactId>
<version>${jersey.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.test-framework.providers</groupId>
<artifactId>jersey-test-framework-provider-bundle</artifactId>
<type>pom</type>
<scope>test</scope>
<dependency>
<groupId>org.glassfish.jersey.ext</groupId>
<artifactId>jersey-bean-validation</artifactId>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.test-framework.providers</groupId>
<artifactId>jersey-test-framework-provider-grizzly2</artifactId>
</dependency>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-json</artifactId>
<version>1.16</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.10</version>
<scope>test</scope>
</dependency>
<!-- Dependency for Mockito -->
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-all</artifactId>
<version>1.9.5</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>2.5.1</version>
<inherited>true</inherited>
<configuration>
<source>1.7</source>
<target>1.7</target>
</configuration>
</plugin>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>exec-maven-plugin</artifactId>
<version>1.2.1</version>
<executions>
<execution>
<goals>
<goal>java</goal>
</goals>
</execution>
</executions>
<configuration>
<mainClass>com.sky.Main</mainClass>
</configuration>
</plugin>
</plugins>
</build>
<properties>
<jersey.version>2.15</jersey.version>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
您将需要使用ResourceConfig
,因为您需要显式注册CMSApi
,以便您可以在注册之前使用SkyService
注入它。 它基本上看起来像
// Sample interface
public interface SkyService {
public String getMessage();
}
@Path("...")
public class CMSApi {
private SkyService service;
public void setSkyService( SkyService service ) { this.service = service; }
}
// Somewhere in test code
CMSApi api = new CMSApi();
SkyServicer service = Mockito.mock(SkyService.class);
Mockito.when(server.getMessage()).thenReturn("Hello World");
api.setSkyService(service);
resourceConfig.register(api);
关键是要设置资源类以注入其依赖项。 更容易测试是依赖注入的好处之一。 您可以通过构造函数注入,或者像我一样通过setter注入,或者通过带有@Inject
注释的注入框架注入。
你可以在这里看到一个更完整的例子,它使用Jersey DI框架HK2 。 您还可以在此处查看有关泽西岛和HK2的更多信息。 然后示例还使用Jersey测试框架 ,我认为您已经将其作为依赖项,因此您可能希望利用它。 这是使用泽西1的另一个例子 ,但概念是相同的,你可以从中得到一些想法。
顺便说一句,看起来你正在使用我熟悉的Jersey原型。 因此,您显示的配置代码位于不同的类中。 你可能不想搞砸当前的应用程序配置,所以也许最好的办法是使用测试框架并创建一个新配置,如我链接的示例所示。 否则,您需要一些其他方法来从测试类访问资源配置。 或者,您可以在测试类中设置新服务器,而不是在Main
类中启动服务器。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.