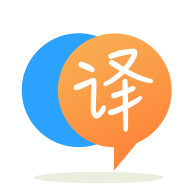
[英]Reading hex values from a String and storing them into an integer array in c/c++
[英]Reading in strings from a file and storing them in an array as an integer in C
我正在尝试读取包含数百个整数的文件,其中一些正数,一些负数并将它们存储在数组中。 但是,必须使用strtok将它们作为字符串读取。 我不断遇到细分错误,但不确定为什么。 计数是为了确定文件中有多少个整数。
/*Input file looks like this:
718321747 -1828022042
-1665405912 -175307986
-53757018 -1551069786 525902369
-1945908378 853648883
*/
int main(int argc, char* argv[])
{
char buffer[50];
char* token;
int count = 0;
int num = 0;
int arr[MAX_SIZE];
if (argc != 2)
{
printf("Invalid number of arguments\n");
return 0;
}
FILE* fptr = fopen(argv[1], "r");
//open file
if (fptr == NULL)
{
printf("Unable to open file\n");
return 0;
}
while(fgets(buffer, 50, fptr))
//to get the file line by line
{
token = strtok(buffer, "\n\t ");
//find first token
num = atoi(token);
//convert it to an int
arr[count] = num;
//store in array
count++;
while(token != NULL)
//get rest of tokens and convert to int
{
token = strtok(buffer, "\n\t ");
num = atoi(token);
arr[count] = num;
count++;
}
}
return 0;
}
您从不检查是否在字符串中找到了令牌,必须在尝试调用atoi()
之前检查strtok()
没有返回NULL
。
然后您继续使用strtok()
扫描同一字符串,并在每次迭代中传递该字符串,这也是错误的,您应该在第一次之后传递NULL
。
我还建议使用strtol()
代替atoi()
来检查转换是否成功。
检查此代码,我修复了它
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 1000 /* ? whatever value you think is good. */
int main(int argc, char* argv[])
{
char buffer[50];
int count = 0;
int arr[MAX_SIZE];
if (argc != 2)
{
printf("Invalid number of arguments\n");
return 0;
}
FILE* fptr = fopen(argv[1], "r");
//open file
if (fptr == NULL)
{
printf("Unable to open file\n");
return 0;
}
//to get the file line by line
while ((fgets(buffer, 50, fptr) != NULL) && (count < MAX_SIZE))
{
char *pointer;
char *token;
pointer = buffer;
while (((token = strtok(pointer, "\n\t ")) != NULL) && (count < MAX_SIZE))
{
char *endptr;
arr[count] = strtol(token, &endptr, 10);
printf("%d\n", arr[count]);
if (*endptr != '\0')
printf("error: could not convert %s to integer\n", token);
else
count++;
pointer = NULL;
}
}
return 0;
}
我不确定它是否对您有用,因为我还没有看到您输入数据的结构,但是我确定它不会导致分段错误。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.