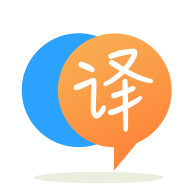
[英]reloadData of UITableView with Dynamic cell heights unwanted scroll
[英]reloadData() of UITableView with Dynamic cell heights causes jumpy scrolling
我觉得这可能是一个常见问题,想知道是否有任何通用的解决方案。
基本上,我的 UITableView 具有每个单元格的动态单元格高度。 如果我不在 UITableView 的顶部并且我不是tableView.reloadData()
,向上滚动就会变得跳跃。
我相信这是因为我重新加载了数据,当我向上滚动时,UITableView 正在重新计算每个单元格的高度。 我该如何缓解这种情况,或者如何仅将数据从某个 IndexPath 重新加载到 UITableView 的末尾?
此外,当我设法一直滚动到顶部时,我可以向下滚动然后向上滚动,没有跳跃的问题。 这很可能是因为 UITableViewCell 的高度已经计算出来了。
为了防止跳跃,您应该在加载时保存单元格的高度,并在tableView:estimatedHeightForRowAtIndexPath
给出精确值:
迅速:
var cellHeights = [IndexPath: CGFloat]()
func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) {
cellHeights[indexPath] = cell.frame.size.height
}
func tableView(_ tableView: UITableView, estimatedHeightForRowAt indexPath: IndexPath) -> CGFloat {
return cellHeights[indexPath] ?? UITableView.automaticDimension
}
目标 C:
// declare cellHeightsDictionary
NSMutableDictionary *cellHeightsDictionary = @{}.mutableCopy;
// declare table dynamic row height and create correct constraints in cells
tableView.rowHeight = UITableViewAutomaticDimension;
// save height
- (void)tableView:(UITableView *)tableView willDisplayCell:(UITableViewCell *)cell forRowAtIndexPath:(NSIndexPath *)indexPath {
[cellHeightsDictionary setObject:@(cell.frame.size.height) forKey:indexPath];
}
// give exact height value
- (CGFloat)tableView:(UITableView *)tableView estimatedHeightForRowAtIndexPath:(NSIndexPath *)indexPath {
NSNumber *height = [cellHeightsDictionary objectForKey:indexPath];
if (height) return height.doubleValue;
return UITableViewAutomaticDimension;
}
Swift 3 版本的已接受答案。
var cellHeights: [IndexPath : CGFloat] = [:]
func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) {
cellHeights[indexPath] = cell.frame.size.height
}
func tableView(_ tableView: UITableView, estimatedHeightForRowAt indexPath: IndexPath) -> CGFloat {
return cellHeights[indexPath] ?? 70.0
}
跳跃是因为估计的高度错误。 估计的行高度与实际高度的差异越大,表格在重新加载时可能会跳得越多,尤其是滚动得越远。 这是因为表的估计大小与其实际大小完全不同,迫使表调整其内容大小和偏移量。 所以估计的高度不应该是一个随机值,而是接近你认为的高度。 如果您的单元格类型相同,我在设置UITableViewAutomaticDimension
时也遇到过
func viewDidLoad() {
super.viewDidLoad()
tableView.estimatedRowHeight = 100//close to your cell height
}
如果您在不同部分有各种单元格,那么我认为更好的地方是
func tableView(tableView: UITableView, estimatedHeightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat {
//return different sizes for different cells if you need to
return 100
}
@Igor 的回答在这种情况下工作正常,它的Swift-4
代码。
// declaration & initialization
var cellHeightsDictionary: [IndexPath: CGFloat] = [:]
在UITableViewDelegate
以下方法中
func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) {
// print("Cell height: \(cell.frame.size.height)")
self.cellHeightsDictionary[indexPath] = cell.frame.size.height
}
func tableView(_ tableView: UITableView, estimatedHeightForRowAt indexPath: IndexPath) -> CGFloat {
if let height = self.cellHeightsDictionary[indexPath] {
return height
}
return UITableView.automaticDimension
}
我已经尝试了上述所有解决方法,但没有任何效果。
在花了几个小时并经历了所有可能的挫折之后,找到了解决这个问题的方法。 这个解决方案简直就是救命稻草! 像魅力一样工作!
斯威夫特 4
let lastContentOffset = tableView.contentOffset
tableView.beginUpdates()
tableView.endUpdates()
tableView.layer.removeAllAnimations()
tableView.setContentOffset(lastContentOffset, animated: false)
我添加了它作为扩展,使代码看起来更干净,避免每次我想重新加载时都写这些行。
extension UITableView {
func reloadWithoutAnimation() {
let lastScrollOffset = contentOffset
beginUpdates()
endUpdates()
layer.removeAllAnimations()
setContentOffset(lastScrollOffset, animated: false)
}
}
最后 ..
tableView.reloadWithoutAnimation()
或者你实际上可以在你的UITableViewCell
awakeFromNib()
方法中添加这些行
layer.shouldRasterize = true
layer.rasterizationScale = UIScreen.main.scale
并做正常的reloadData()
我使用更多方法来解决它:
对于视图控制器:
var cellHeights: [IndexPath : CGFloat] = [:]
func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) {
cellHeights[indexPath] = cell.frame.size.height
}
func tableView(_ tableView: UITableView, estimatedHeightForRowAt indexPath: IndexPath) -> CGFloat {
return cellHeights[indexPath] ?? 70.0
}
作为 UITableView 的扩展
extension UITableView {
func reloadSectionWithoutAnimation(section: Int) {
UIView.performWithoutAnimation {
let offset = self.contentOffset
self.reloadSections(IndexSet(integer: section), with: .none)
self.contentOffset = offset
}
}
}
结果是
tableView.reloadSectionWithoutAnimation(section: indexPath.section)
我今天遇到了这个并观察到:
cellForRowAtIndexPath
没有帮助。修复实际上非常简单:
覆盖estimatedHeightForRowAtIndexPath
并确保它返回正确的值。
有了这个,我的 UITableViews 中所有奇怪的抖动和跳跃都停止了。
注意:我实际上知道我的单元格的大小。 只有两个可能的值。 如果你的细胞是真正的可变大小,那么你可能想缓存cell.bounds.size.height
从tableView:willDisplayCell:forRowAtIndexPath:
实际上,您可以使用reloadRowsAtIndexPaths
仅重新加载某些行,例如:
tableView.reloadRowsAtIndexPaths(indexPathArray, withRowAnimation: UITableViewRowAnimation.None)
但是,一般来说,您还可以为表格单元格高度的变化设置动画,如下所示:
tableView.beginUpdates()
tableView.endUpdates()
用高值覆盖estimatedHeightForRowAtIndexPath 方法,例如300f
这应该可以解决问题:)
这是一个更短的版本:
func tableView(_ tableView: UITableView, estimatedHeightForRowAt indexPath: IndexPath) -> CGFloat {
return self.cellHeightsDictionary[indexPath] ?? UITableViewAutomaticDimension
}
我相信有一个错误是在 iOS11 中引入的。
那是当您reload
tableView contentOffSet
时意外更改。 事实上contentOffset
不应该在重新加载后改变。 它往往是由于UITableViewAutomaticDimension
错误计算而发生的
您必须保存contentOffSet
并在重新加载完成后将其设置回您保存的值。
func reloadTableOnMain(with offset: CGPoint = CGPoint.zero){
DispatchQueue.main.async { [weak self] () in
self?.tableView.reloadData()
self?.tableView.layoutIfNeeded()
self?.tableView.contentOffset = offset
}
}
你如何使用它?
someFunctionThatMakesChangesToYourDatasource()
let offset = tableview.contentOffset
reloadTableOnMain(with: offset)
这个答案来自here
这个在 Swift4 中对我有用:
extension UITableView {
func reloadWithoutAnimation() {
let lastScrollOffset = contentOffset
reloadData()
layoutIfNeeded()
setContentOffset(lastScrollOffset, animated: false)
}
}
我发现解决这个问题的方法之一是
CATransaction.begin()
UIView.setAnimationsEnabled(false)
CATransaction.setCompletionBlock {
UIView.setAnimationsEnabled(true)
}
tableView.reloadSections([indexPath.section], with: .none)
CATransaction.commit()
这些解决方案都不适合我。 这是我使用Swift 4 和 Xcode 10.1所做的......
在 viewDidLoad() 中,声明表格动态行高并在单元格中创建正确的约束...
tableView.rowHeight = UITableView.automaticDimension
同样在 viewDidLoad() 中,将所有 tableView 单元格笔尖注册到 tableview,如下所示:
tableView.register(UINib(nibName: "YourTableViewCell", bundle: nil), forCellReuseIdentifier: "YourTableViewCell")
tableView.register(UINib(nibName: "YourSecondTableViewCell", bundle: nil), forCellReuseIdentifier: "YourSecondTableViewCell")
tableView.register(UINib(nibName: "YourThirdTableViewCell", bundle: nil), forCellReuseIdentifier: "YourThirdTableViewCell")
在 tableView heightForRowAt 中,在 indexPath.row 处返回等于每个单元格高度的高度...
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
if indexPath.row == 0 {
let cell = Bundle.main.loadNibNamed("YourTableViewCell", owner: self, options: nil)?.first as! YourTableViewCell
return cell.layer.frame.height
} else if indexPath.row == 1 {
let cell = Bundle.main.loadNibNamed("YourSecondTableViewCell", owner: self, options: nil)?.first as! YourSecondTableViewCell
return cell.layer.frame.height
} else {
let cell = Bundle.main.loadNibNamed("YourThirdTableViewCell", owner: self, options: nil)?.first as! YourThirdTableViewCell
return cell.layer.frame.height
}
}
现在为 tableViewestimatedHeightForRowAt 中的每个单元格提供一个估计的行高。 尽可能准确...
func tableView(_ tableView: UITableView, estimatedHeightForRowAt indexPath: IndexPath) -> CGFloat {
if indexPath.row == 0 {
return 400 // or whatever YourTableViewCell's height is
} else if indexPath.row == 1 {
return 231 // or whatever YourSecondTableViewCell's height is
} else {
return 216 // or whatever YourThirdTableViewCell's height is
}
}
那应该工作...
调用 tableView.reloadData() 时不需要保存和设置 contentOffset
我有 2 个不同的单元格高度。
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
let cellHeight = CGFloat(checkIsCleanResultSection(index: indexPath.row) ? 130 : 160)
return Helper.makeDeviceSpecificCommonSize(cellHeight)
}
在我添加了EstimatedHeightForRowAt 之后,就没有更多的跳跃了。
func tableView(_ tableView: UITableView, estimatedHeightForRowAt indexPath: IndexPath) -> CGFloat {
let cellHeight = CGFloat(checkIsCleanResultSection(index: indexPath.row) ? 130 : 160)
return Helper.makeDeviceSpecificCommonSize(cellHeight)
}
尝试在返回单元格之前调用cell.layoutSubviews()
func cellForRowAtIndexPath(_ indexPath: NSIndexPath) -> UITableViewCell?
. 这是 iOS8 中的已知错误。
您可以在ViewDidLoad()
使用以下内容
tableView.estimatedRowHeight = 0 // if have just tableViewCells <br/>
// use this if you have tableview Header/footer <br/>
tableView.estimatedSectionFooterHeight = 0 <br/>
tableView.estimatedSectionHeaderHeight = 0
我有这种跳跃行为,我最初能够通过设置精确的估计标题高度来缓解它(因为我只有 1 个可能的标题视图),但是跳跃然后开始发生在标题内,不再影响整个表格。
按照这里的答案,我知道它与动画有关,所以我发现表格视图在堆栈视图内,有时我们会在动画块内调用stackView.layoutIfNeeded()
。 我的最终解决方案是确保除非“真正”需要,否则不会发生此调用,因为即使“不需要”布局“如果需要”在该上下文中也具有视觉行为。
我遇到过同样的问题。 我在没有动画的情况下进行了分页和重新加载数据,但这并没有帮助滚动防止跳跃。 我有不同尺寸的 iPhone,滚动条在 iphone8 上不跳动,但在 iphone7+ 上跳动
我对viewDidLoad函数应用了以下更改:
self.myTableView.estimatedRowHeight = 0.0
self.myTableView.estimatedSectionFooterHeight = 0
self.myTableView.estimatedSectionHeaderHeight = 0
我的问题解决了。 我希望它也能帮助你。
对我来说,它与“heightForRowAt”一起工作
extension APICallURLSessionViewController: UITableViewDelegate {
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
print("Inside heightForRowAt")
return 130.50
}
}
对我来说,工作解决方案是
UIView.setAnimationsEnabled(false)
tableView.performBatchUpdates { [weak self] in
self?.tableView.reloadRows(at: [indexPath], with: .none)
} completion: { [weak self] _ in
UIView.setAnimationsEnabled(true)
self?.tableView.scrollToRow(at: indexPath, at: .top, animated: true) // remove if you don't need to scroll
}
我有可扩展的单元格。
实际上我发现如果您使用reloadRows
导致跳转问题。 那么你应该尝试像这样使用reloadSections
:
UIView.performWithoutAnimation {
tableView.reloadSections(NSIndexSet(index: indexPath.section) as IndexSet, with: .none)
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.