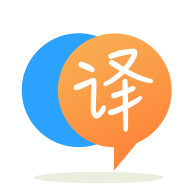
[英]C: qsort has a problem sorting unsigned long long members of a structure
[英]qsort not sorting list of unsigned long ints
在尝试排序一系列无符号长整数时,以下代码会产生奇怪的结果。 我不知道为什么。 它编译无警。 问题出在我使用qsort的某个地方但是我已经把这条线扼杀了几个小时了。 我认为我的比较功能还可以。 我试图半盲地尝试所有可以想到的排列,以确保我传递正确的论据,但仍然没有骰子。 任何帮助找到我的问题将不胜感激:
#include<stdio.h>
#include<stdlib.h>
#define LENGTH 10
static int cmpfunc (const void *a, const void *b) {
const unsigned long int x=*(const unsigned long int*)a, y=*(const unsigned long int*)b;
printf("x=%lu ",x); printf("y=%lu ",y);
if ( x==y ) {
puts("returning 0..."); return 0;
} else {
if ( x>y ) {
puts("returning 1..."); return 1;
} else {
puts("returning -1..."); return -1;
}
}
}
int main(void) {
/* declare the storage for our "array". Using malloc instead of [] because in
real program array size shall be dynamic */
unsigned long int *mystorage=malloc(LENGTH*sizeof(unsigned long int)); /* check for NULL */
/* fill up array with values in non-monotonic order and show the values */
for(unsigned long int counter=0;counter<LENGTH;counter++) {
*(mystorage+counter*sizeof(unsigned long int))=(unsigned long int)(counter*(LENGTH-counter));
printf("value is %lu\n",*(mystorage+counter*sizeof(unsigned long int)));
}
/* sort array */
qsort(mystorage, LENGTH, sizeof(unsigned long int), cmpfunc);
/* print out array again to see if it changed */
for(unsigned long int counter=0;counter<LENGTH;counter++) {
printf("value is %lu\n",*(mystorage+counter*sizeof(unsigned long int)));
}
exit(EXIT_SUCCESS);
}
*(mystorage+counter*sizeof(unsigned long int))
=
(unsigned long int)(counter*(LENGTH-counter));
... 是不正确的。 (我稍微重新安排了你的空白。)它应该是
mystorage[counter] =
counter*(LENGTH-counter); // the cast is redundant
以下三个是等效的:
mystorage[counter]
counter[mystorage] // yes, this weird thing is equivalent
*(mystorage+counter)
将最后一行与您的代码进行比较。 当您添加指针和整数时,编译器已经知道要移动正确的字节数。 因为你包含sizeof东西,你有两个等效的行(与上面三行不同)
*(mystorage + counter*sizeof(unsigned long int))
mystorage[counter*sizeof(unsigned long int)]
应该清楚的是,这两个将访问数组边界之外。
您的程序在该部分中是错误的
for(unsigned long int counter=0;counter<LENGTH;counter++) {
*(mystorage+counter*sizeof(unsigned long int))=(unsigned long int)(counter*(LENGTH-counter));
printf("value is %lu\n",*(mystorage+counter*sizeof(unsigned long int)));
}
当你向指针编译器添加一些整数时自动使用地址算术,所以你不应该使用*sizeof(unsigned long int)
。 打印循环中出现同样的错误。 所以使用简单的索引mystorage[counter]
或*(mystorage+counter)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.