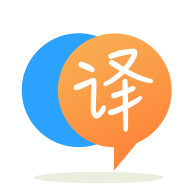
[英]ServerSocket accept method - How can I down cast to a subclass type?
[英]How can I test if a Method will accept a parameter type?
说我有以下代码......
@FunctionalInterface
static interface MessageFunction<T> {
void send(T obj);
}
static @interface Message {
Class<?> value();
}
static class Foo {
@Message(String.class)
MessageFunction<String> bass = (string) -> {
// Do Stuff
};
}
static class MessageManager {
Map<Class<?>, MessageFunction<?>> messages = new HashMap<>();
public void register(Object obj) {
for (Field field : obj.getClass().getDeclaredFields()) {
Message message = field.getAnnotation(Message.class);
if (message != null) {
MessageFunction<?> function;
try {
function = (MessageFunction<?>) field.get(obj);
} catch (IllegalArgumentException | IllegalAccessException e) {
e.printStackTrace();
return;
}
Method sendMethod;
try {
// Will this work?
sendMethod = function.getClass().getDeclaredMethod("send", Object.class);
} catch (NoSuchMethodException | SecurityException e) {
e.printStackTrace();
return;
}
// How do I do something like this?
/*if (sendMethod.testParamaters(message.value())) {
this.messages.put(message.value(), function);
}*/
}
}
}
}
public static void main(String[] args) {
MessageManager manager = new MessageManager();
manager.register(new Foo());
}
我正在反映一个引用泛型类型的@FunctionalInterface
的字段。 因为方法参数也是通用的,所以我无法知道它接受哪些参数,因此我必须通过其他方式(注释)传递它。
问题是有注释值,泛型类型不必匹配,似乎没有办法检查。 如果注释中列出的类型不会被send方法接受,我就不会注册失败。
如果不实际调用方法,我将如何处理这个问题。 有办法吗? 更好的是,虽然我知道它很可能是不可能的,但有没有办法知道没有注释的参数类型是什么?
试试TypeTools 。 首先,你需要获得一个对你在register
方法中获得的bass
声明类的引用:
Class<?> bassClass = field.getDeclaringClass();
然后,您可以使用TypeTools来解析它为MessageFunction提供的类型参数T
:
Class<?> msgType = TypeResolver.resolveRawArgument(MessageFunction.class, bassClass);
以下只是一个建议,我在我的项目中使用过它。 但对于这个问题,它并不是一个完美的解决方案。 也许你可以下载GenericHibernateDao框架的源代码并查看方法“getTypeArguments”的源代码。 我觉得它真酷!
// get a class object for your entity
Class clazz = ...
Type type = clazz.getGenericSuperclass();
if (type instanceof ParameterizedType) {
Type trueType = ((ParameterizedType)type).getActualTypeArguments()[0];
Class modelClass = (Class) trueType;
// Now you can creat an Instance in you generic parameterType
Object entity = modelClass.forInstance();
}
我在我的一些代码中做了类似的事情。这是一个片段。
Method[] meths = actionClass.getMethods();
for (Method meth : meths) {
Class<?>[] pTypes = meth.getParameterTypes();
/*
* Filter out all methods that do not meet correct
* signature. The correct signature for an action method
* is: String actionName(HttpServletRequest request)
*/
//...check for the correct number of params and the correct param type
if (pTypes.length != 1 || !HttpServletRequest.class.toString().equals(pTypes[0].toString())) {
continue;
} else {
//...check for return type
if (!String.class.toString().equals(meth.getReturnType().toString())) {
continue;
}
}
//If you make it here than that means the method
//meets the requirements to be a full fledged action.
//...
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.