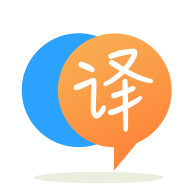
[英]FileNotFoundException (Access is denied) when trying to write to a new file
[英]Receiving FileNotFoundException (Access is denied) when using JAXB to marshal to File
当我编组到system.out。 我的列表出现在控制台中没问题。 当我尝试将其发送到文件时,出现错误提示
javax.xml.bind.JAXBException
- with linked exception:
[java.io.FileNotFoundException: c:\computerparts.xml (Access is denied)]
at javax.xml.bind.helpers.AbstractMarshallerImpl.marshal(Unknown Source)
at com.cooksys.assessment.List$2.doSaveCommand(List.java:201)
at com.cooksys.assessment.List$2.actionPerformed(List.java:179).....ETC
在我的资料中,我没有任何错误。 下面是我的save(marshall)方法
JMenuItem mntmSave = new JMenuItem("Save");
mntmSave.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
doSaveCommand();
} catch (JAXBException e1) {
e1.printStackTrace();
}
}
private void doSaveCommand() throws JAXBException {
ArrayList<String> save = new ArrayList<>();
for (int i = 0; i < destination.size(); i++) {
save.add((String)destination.getElementAt(i));
}
ListWrapper Assembledparts = new ListWrapper();
Assembledparts.setAssembledparts (save);
File file = new File ("c:\\computerparts.xml");
JAXBContext context = JAXBContext.newInstance(ListWrapper.class);
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
marshaller.marshal(Assembledparts, System.out);
marshaller.marshal(Assembledparts, file);
}
});
我希望如果我可以将列表显示在控制台中,那么我应该能够编组到一个文件。 我想念什么? 下面是我的getter setter课程
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
@XmlAccessorType(XmlAccessType.FIELD)
public class ListWrapper {
@XmlElement(name = "part")
private List<String> save;
public List<String> save() {
if (save == null) {
save = new ArrayList<String>();
}
return this.save;
}
public void setAssembledparts (ArrayList<String> save) {
this.save = save;
}
如果需要,主要代码如下
import javax.swing.*;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import java.awt.*;
import java.awt.event.*;
import java.io.File;
import java.util.ArrayList;
public class List implements ActionListener{
JList destinationList, sourceList;
JButton buttonin, buttonout;
DefaultListModel source;
static DefaultListModel destination;
public JPanel createContentPane (){
JPanel totalGUI = new JPanel();
source = new DefaultListModel();
destination = new DefaultListModel();
String shoppingItems[] = {"Case", "Motherboard", "CPU", "RAM", "GPU",
"HDD", "PSU"};
for(int i = 0; i < shoppingItems.length; i++)
{
source.addElement(shoppingItems[i]);
}
destinationList = new JList(source);
destinationList.setVisibleRowCount(10);
destinationList.setFixedCellHeight(20);
destinationList.setFixedCellWidth(140);
destinationList.setSelectionMode(ListSelectionModel.MULTIPLE_INTERVAL_SELECTION);
JScrollPane list1 = new JScrollPane(destinationList);
sourceList = new JList(destination);
sourceList.setVisibleRowCount(10);
sourceList.setFixedCellHeight(20);
sourceList.setFixedCellWidth(140);
sourceList.setSelectionMode(ListSelectionModel.MULTIPLE_INTERVAL_SELECTION);
JScrollPane list2 = new JScrollPane(sourceList);
JPanel buttonPanel = new JPanel();
buttonin = new JButton(">>");
buttonin.setHorizontalAlignment(SwingConstants.RIGHT);
buttonin.addActionListener(this);
buttonPanel.add(buttonin);
JPanel bottomPanel = new JPanel();
bottomPanel.setLayout(new BoxLayout(bottomPanel, BoxLayout.LINE_AXIS));
bottomPanel.add(Box.createRigidArea(new Dimension(10,0)));
bottomPanel.add(list1);
bottomPanel.add(Box.createRigidArea(new Dimension(5,0)));
bottomPanel.add(buttonPanel);
buttonout = new JButton("<<");
buttonout.addActionListener(this);
buttonPanel.add(buttonout);
bottomPanel.add(Box.createRigidArea(new Dimension(5,0)));
bottomPanel.add(list2);
bottomPanel.add(Box.createRigidArea(new Dimension(10,0)));
totalGUI.add(bottomPanel);
totalGUI.setOpaque(true);
return totalGUI;
}
private JPanel createSquareJPanel(Color color, int size) {
JPanel tempPanel = new JPanel();
tempPanel.setBackground(color);
tempPanel.setMinimumSize(new Dimension(size, size));
tempPanel.setMaximumSize(new Dimension(size, size));
tempPanel.setPreferredSize(new Dimension(size, size));
return tempPanel;
}
public void actionPerformed(ActionEvent e)
{
int i = 0;
if(e.getSource() == buttonin)
{
int[] fromindex = destinationList.getSelectedIndices();
Object[] from = destinationList.getSelectedValues();
for(i = 0; i < from.length; i++)
{
destination.addElement(from[i]);
}
for(i = (fromindex.length-1); i >=0; i--)
{
source.remove(fromindex[i]);
}
}
else if(e.getSource() == buttonout)
{
Object[] to = sourceList.getSelectedValues();
int[] toindex = sourceList.getSelectedIndices();
for(i = 0; i < to.length; i++)
{
source.addElement(to[i]);
}
for(i = (toindex.length-1); i >=0; i--)
{
destination.remove(toindex[i]);
}
}
}
private static void createAndShowGUI() {
JFrame.setDefaultLookAndFeelDecorated(true);
JFrame frame = new JFrame("PC Parts Builder");
JMenu file = new JMenu ("File");
List demo = new List();
frame.setContentPane(demo.createContentPane());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
JMenuBar menuBar = new JMenuBar();
frame.setJMenuBar(menuBar);
JMenu mnFile = new JMenu("File");
menuBar.add(mnFile);
JMenuItem item;
file.add(item = new JMenuItem("Load"));
item.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
doOpenCommand();
} catch (JAXBException e1) {
e1.printStackTrace();
}
}
private void doOpenCommand() throws JAXBException{
File file = new File ("c:\\computerparts.xml" );
JAXBContext context = JAXBContext.newInstance(ListWrapper.class);
Unmarshaller unmarshal = context.createUnmarshaller();
ListWrapper assembledparts = (ListWrapper) unmarshal.unmarshal(file);
System.out.println(assembledparts);
}
});
mnFile.add(item);
JMenuItem mntmSave = new JMenuItem("Save");
mntmSave.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
doSaveCommand();
} catch (JAXBException e1) {
e1.printStackTrace();
}
}
private void doSaveCommand() throws JAXBException {
ArrayList<String> save = new ArrayList<>();
for (int i = 0; i < destination.size(); i++) {
save.add((String)destination.getElementAt(i));
}
ListWrapper Assembledparts = new ListWrapper();
Assembledparts.setAssembledparts (save);
File file = new File ("c:\\computerparts.xml");
JAXBContext context = JAXBContext.newInstance(ListWrapper.class);
Marshaller marshaller = context.createMarshaller();
marshaller.setProperty (Marshaller.JAXB_FORMATTED_OUTPUT, true);
marshaller.marshal(Assembledparts, System.out);
marshaller.marshal(Assembledparts, file);
}
});
mnFile.add(mntmSave);
JMenuItem mntmNewMenuItem = new JMenuItem("Exit");
mntmNewMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.exit(0);
}
});
mnFile.add(mntmNewMenuItem);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}
[java.io.FileNotFoundException:c:\\ computerparts.xml(拒绝访问)]
尝试避免c:并将源文件放在另一个文件夹中,即。 C:/temp/FileName.xml
这是要注意的行: [java.io.FileNotFoundException: c:\\computerparts.xml (Access is denied)]
要么computerparts.xml不存在,要么您没有写权限。 将该文件放在其他位置,然后更新“ File file = new File ("c:\\\\computerparts.xml");
行File file = new File ("c:\\\\computerparts.xml");
指向新文件的位置。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.