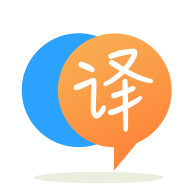
[英]Array of doubles — most frequent value method? (without hashmaps or sorting)
[英]Find the most frequent value in an array of double in Java (without hashmaps or sorting)
编写一个完整的Java程序,该程序执行以下操作:
创建一个由100个double组成的数组。
从名为values.txt的文件中读取未知数量的double。
文件中将至少有2个不同的值,并且不超过100个不同的值。 这些值将按未排序的顺序排列。 值将不小于0,且不大于99。
输出文件中最频繁出现的值。
输出文件中出现频率最低的值。 该值必须至少出现一次才能输出。
输出所有数组值的平均值。
您必须为#2-5中的每一项创建并使用单独的方法。
到目前为止,这就是我所拥有的。 我一生无法解决如何正确解决这个问题:
import java.util.*;
import java.io.*;
public class arrayProgram2 {
static Scanner console = new Scanner(System.in);
static final int ARRAY_SIZE = 100;
static int numOfElements = 0;
public static void main(String[] args) throws FileNotFoundException {
Scanner inFile = new Scanner(new FileReader("values.txt"));
double[] Arr1 = new double[ARRAY_SIZE];
while (inFile.hasNext()) {
Arr1[numOfElements] = inFile.nextDouble();
numOfElements++;
}
System.out.println("There are " + numOfElements + " values.");
System.out.printf("The average of the values is %.2f%n", avgArray(Arr1));
System.out.println("The sum is " + sumArray(Arr1));
inFile.close();
} //end main
//Method to calculate the sum
public static double sumArray(double[] list) {
double sum = 0;
for (int index = 0; index < numOfElements; index++) {
sum = sum + list[index];
}
return sum;
}
//Method to calculate the average
public static double avgArray(double[] list) {
double sum = 0;
double average = 0;
for (int index = 0; index < numOfElements; index++) {
sum = sum + list[index];
}
average = sum / numOfElements;
return average;
}
} //end program
请注意,即使没有必要,我也需要制作一个double数组。
如果所有值都是int
,则应使用int
数组而不是double
。 所有值都在0-99范围内。 因此,您可以增加输入值的频率。 看下面的逻辑:
int[] freqArr= new int[100];
while (inFile.hasNext()){
int value = inFile.nextInt();
freqArr[value]++; // count the frequency of selected value.
}
现在从freqArr
计算最大频率
int maxFreq=0;
for(int freq : freqArr){
if(maxFreq < freq){
maxFreq = freq;
}
}
注意:如果必须使用double array,则还可以使用double array,例如:
double[] freqArr= new double[100];
while (inFile.hasNext()){
freqArr[(int)inFile.nextDouble()]++;
}
无需这样排序就可以找到最常出现的值:
static int countOccurrences(double[] list, double targetValue) {
int count = 0;
for (int i = 0; i < list.length; i++) {
if (list[i] == targetValue)
count++;
}
}
static double getMostFrequentValue(double[] list) {
int mostFrequentCount = 0;
double mostFrequentValue = 0;
for (int i = 0; i < list.length; i++) {
double value = list[i];
int count = countOccurrences(list, value);
if (count > mostFrequentCount) {
mostFrequentCount = count;
mostFrequentValue = value;
}
}
return mostFrequentValue;
}
Pham Thung是正确的:-您读入infile.nextInt()整数,为什么需要使用双精度数组来存储它们? –范通
如果它是整数数组,则可以在n天内实现您的第一个功能。
您的问题是,
值将不小于0,且不大于99。
因此,1.制作一个大小为100.(Counter [])的数组。2.遍历当前数组的值,然后将计数添加到Counter数组。
例如:如果double数组包含2 3 2 5 0 0 0
我们的计数器阵列将像
位置:0 1 2 3 4 5 6 ...... 100
值:3 0 1 1 0 1 0 ..............
等等。
您可以为此使用以下算法
对于我从1到Arr1的长度
一世。 if(Arr1 [i] == num)
一种。 增加currentCount
ii。 其他
一种。 if(count> currentCount)
A.分配currentCount进行计数
B.将num分配给mostCommon
C.将Arr1 [i]分配给num
D.为currentCount分配1
在此循环结束时,您将在mostCommon变量中拥有最常见的数字,它是出现的次数。
注意:我不知道如何格式化算法
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.