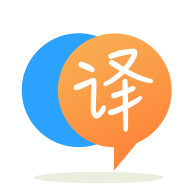
[英]How to write this SQL query as a LINQ statement in .NET Core (C#)?
[英]How to add if statement to a LINQ query in C#?
考虑以下查询方法:
internal List<Product> productInStockQuery(InStock inStock1)
{
var inStock =
from p in products
where p.INStock == inStock1
select p;
return inStock.ToList<Product>();
}
我希望该方法执行以下操作:
如果inStock1 == InStock.needToOrder
我想搜索两者(类似):( (instock1==InStock.needToOrder && instock1==InStock.False)
我怎样才能做到这一点?
我还想知道是否可以创建一种方法,使我可以在一个方法中搜索所有“产品”字段。
编辑部分 :(我有第二个问题)试图更好地解释自己:我的课有几个字段,现在对于每个字段我都有一个如上所示的方法,我想知道是否有可能创建一个允许我使用的特殊变量访问产品中的每个字段而无需实际输入字段名称或getter而不是p.price / p.productName,我只输入p.VARIABLE,并根据此变量,我将访问所需的字段/ getter
如果存在这样的选择,如果您能告诉我要在网络上搜索什么,我将不胜感激。
ps非常感谢所有快速响应,我正在全部检查它们。
您是说使用枚举之类的意思吗?
internal enum InStock
{
NeedToOrder,
NotInStock,
InStock
}
internal class Product
{
public string Name { get; set; }
public InStock Stock { get; set; }
}
然后将一个集合传递给该方法...
internal static List<Product> ProductInStockQuery(List<Product> products)
{
var inStock =
from p in products
where p.Stock != InStock.NeedToOrder && p.Stock != InStock.NotInStock
select p;
return inStock.ToList();
}
创建列表并将其传递给...
var prods = new List<Product>
{
new Product
{
Name = "Im in stock",
Stock = InStock.InStock
},
new Product
{
Name = "Im in stock too",
Stock = InStock.InStock
},
new Product
{
Name = "Im not in stock",
Stock = InStock.NotInStock
},
new Product
{
Name = "need to order me",
Stock = InStock.NotInStock
},
};
var products = ProductInStockQuery(prods);
您可以根据输入变量来编写查询,如下所示:
var inStock =
from p in products
select p;
if(inStock1 == InStock.needToOrder)
{
inStock = (from p in inStock where p.INStock == InStock.needToOrder || instock1 == InStock.False select p);
}
else
{
inStock = (from p in inStock where p.INStock == inStock1 select p);
}
return inStock.ToList<Product>();
我相信您可以使用WhereIf LINQ扩展方法来使其更清晰和可重用。 这是扩展的例子
public static class MyLinqExtensions
{
public static IQueryable<T> WhereIf<T>(this IQueryable<T> source, Boolean condition, System.Linq.Expressions.Expression<Func<T, Boolean>> predicate)
{
return condition ? source.Where(predicate) : source;
}
public static IEnumerable<T> WhereIf<T>(this IEnumerable<T> source, Boolean condition, Func<T, Boolean> predicate)
{
return condition ? source.Where(predicate) : source;
}
}
在下面,您可以看到一个如何使用此方法的示例
class Program
{
static void Main(string[] args)
{
var array = new List<Int32>() { 1, 2, 3, 4 };
var condition = false; // change it to see different outputs
array
.WhereIf<Int32>(condition, x => x % 2 == 0) // predicate will be applied only if condition TRUE
.WhereIf<Int32>(!condition, x => x % 3 == 0) // predicate will be applied only if condition FALSE
.ToList() // don't call ToList() multiple times in the real world scenario
.ForEach(x => Console.WriteLine(x));
}
}
q = (from p in products);
if (inStock1 == InStock.NeedToOrder)
q = q.Where(p => p.INStock == InStock.False || p.InStock == InStock.needToOrder);
else
q = q.Where(p => p.INStock == inStock1);
return q.ToList();
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.