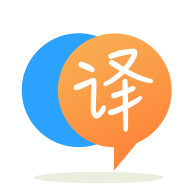
[英]How to print elements of a std::vector<std::any> vector in C++?
[英]Storing many elements in std::vector c++
对于我的一个应用程序,我需要生成大小为2 ^ 35的向量(我的RAM的大小为96 GB,因此该向量可以轻松放入RAM中)。
int main ()
{
int i;
/* initialize random seed: */
srand (time(NULL));
vector<int> vec;
do {
i = rand() % 10 + 1;
vec.push_back(i);
} while ((vec.size()*sizeof(int))<pow(2,35));
return 0;
}
但是,我注意到我的do while循环无限执行。 可能的原因之一是vec.size()
范围是long unsigned int,这远远小于插入的元素数量pow(2,35)
,因此我认为它会陷入无限循环。 我可能是错的。 如果我错了,请纠正我。 但是有人可以告诉我如何在vec中插入大于pow(2,35)
数字。
gcc版本:4.8.2
我将尝试通过一个简单的解决方案来解决您的一些问题:
您遇到的第一个问题是空间。 由于您只需要1-10之间的数字,因此int8_t将为您提供更好的服务。
第二是速度。 std::vector
在后台进行了大量的分配和重新分配。 由于您的尺寸固定,因此我认为不需要使用它。 知道了这一点,我们将使用一个简单的数组和线程来提高性能。
这是代码:
#include <array>
#include <random>
#include <thread>
#include <cstdint>
#include <memory>
#include <chrono>
// Since you only need numbers from 1-10, a single byte will work nicely.
const uint64_t size = UINT64_C(0x800000000); // Exactly 2^35
typedef std::array<int8_t, size> vec_t;
// start is first element, end is one-past the last. This is a template so we can generate multiple functions.
template<unsigned s>
void fill(vec_t::iterator start, vec_t::iterator end) {
static const int seed = std::chrono::system_clock::now().time_since_epoch().count()*(s+1);
static std::default_random_engine generator(seed);
static std::uniform_int_distribution<int8_t> distribution(1,10);
for(auto it = start; it != end; ++it) {
*it = distribution(generator); // generates number in the range 1..10
}
}
int main() {
auto vec = std::unique_ptr<vec_t>(new vec_t());
// Each will have its own generator and distribution.
std::thread a(fill<0>, vec->begin(), vec->begin() + size/4);
std::thread b(fill<1>, vec->begin() + size/4, vec->begin() + size/2);
std::thread c(fill<2>, vec->begin() + size/2, vec->begin() + (size/4)*3);
std::thread d(fill<3>, vec->begin() + (size/4)*3, vec->end());
a.join();
b.join();
c.join();
d.join();
return 0;
}
为什么不能使用构造函数?
std::vector<int> vec ( number_of_elements );
这样,您将保留内存,然后可以使用generate或其他方法随机化元素。
更新资料
正如Baum mit Augen所强调的那样,此帖子并未真正回答问题,因为在他的平台条件下4不成立( sizeof(std::size_t)
实际上是8
)。 但是,我将这篇文章留在此处以重点介绍移植代码时可能发生的问题。
原始帖子
我看到的一个问题如下。 让我们假设(大多数平台都满足这些假设)
1) vec.size
返回std::size_t
(不保证);
2) sizeof
返回std::size_t
(保证);
3) std::size_t
是无符号整数类型(保证);
4) sizeof(std::size_t) == 4
(不保证);
5) CHAR_BIT == 8
(不保证)。
(回想一下CHAR_BIT
是char
的位数。)
因此, vec.size()*sizeof(int)
为std::size_t
,其最大值为2^(sizeof(std::size_t)*CHAR_BIT) - 1 == 2^32 - 1 < 2^32 < 2^35
。 因此, vec.size()*sizeof(int)
始终小于2^35
。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.