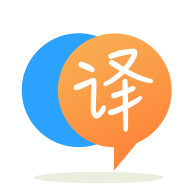
[英]Sorting a list of constructed objects alphabetically using collections.sort
[英]Using Collections.sort() method to sort objects alphabetically
我试过使用Collections.sort(shapes)
但它给了我这个错误:
Bound mismatch: The generic method sort(List<T>) of type Collections is not applicable for the
arguments (ArrayList<CreateShape>). The inferred type CreateShape is not a valid substitute for the
bounded parameter <T extends Comparable<? super T>>
我该怎么解决这个问题?
CreateSpace类
public class CreateSpace implements Space{
private int height;
private int width;
private String layout;
private char[][] space;
private Shape originalShape;
private ArrayList<CreateShape> shapes = new ArrayList<CreateShape>();
public CreateSpace(int height, int width, char[][] space, String layout)
{
this.height = height;
this.width = width;
this.space = space;
this.layout = layout;
}
public void placeShapeAt(int row, int col, Shape shape)
{
int sHeight = shape.getHeight();
int sWidth = shape.getWidth();
if(shape.getHeight() + row > space.length || shape.getWidth() + col > space[0].length)
throw new FitItException("Out of bounds!");
char [][] spaceWithShapes = space;
if(shapeFitsAt(row, col, shape) == true)
{
for(int r = 0; r < sHeight; r++)
{
for(int c = 0; c < sWidth; c++)
{
if(spaceWithShapes[r+row][c+col] == '.' && shape.isFilledAt(r, c) == false)
spaceWithShapes[r+row][c+col] = (((CreateShape)shape).getShape()[r][c]);
}
// shapes.add((CreateShape)shape);
Collections.sort(shapes); // <<------- getting an error here
}
spaceWithShapes = space;
shapes.add((CreateShape)shape);
Collections.sort(shapes); // <<------- getting an error here
}
}
您收到错误是因为当您调用Collections.sort()
仅传递List<T>
作为参数时,它期望list元素实现Comparable
接口。 由于CreateShape
不是这种情况,因此sort()
无法知道应如何对这些对象进行排序。
您应该考虑以下两个选项:
CreateShape
可以实现Comparable<CreateShape>
:如果您认为CreateShape
实例具有应对其进行排序的自然顺序,请执行此操作。 如果要按char
字段排序,例如:
class CreateShape implements Comparable<CreateShape> { private char displayChar; public char getDisplayChar() { return displayChar; } @Override public int compareTo(CreateShape that) { return Character.compare(this.displayChar, that.displayChar); } }
然后你可以简单地调用Collections.sort()
:
Collections.sort(shapes);
创建自定义Comparator<CreateShape>
:如果要任意排序CreateShape
实例,请执行此操作。 你可以有一个按名称排序的Comparator
,另一个按id排序的Comparator
等。示例:
enum DisplayCharComparator implements Comparator<CreateShape> { INSTANCE; @Override public int compare(CreateShape s1, CreateShape s2) { return Character.compare(s1.getDisplayChar(), s2.getDisplayChar()); } }
然后你应该调用Collections.sort()
传递比较器作为参数:
Collections.sort(shapes, DisplayCharComparator.INSTANCE);
注意我将DisplayCharComparator
实现为单例。 那是因为它没有状态,所以不需要有这个比较器的多个实例。 另一种方法是使用静态变量:
class CreateShape {
static final Comparator<CreateShape> DISPLAY_CHAR_COMPARATOR =
new DisplayCharComparator();
static class DisplayCharComparator implements Comparator<CreateShape> { ... }
// rest of the code
}
或者,如果您使用的是Java 8,则可以使用Comparator.comparing
:
shapes.sort(Comparator.comparing(CreateShape::getDisplayChar));
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.