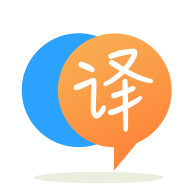
[英]Remove/change Internal styles sheet styles with JavaScript/jQuery
[英]Remove styles from internal style sheet using jQuery
<html>
<head>
<style>
.containerTitle {
background-color:silver;
text-align:center;
font-family:'Segoe UI';
font-size:18px;
font-weight:bold;
height:30px;
}
</style>
</head>
<body>
<div id="a"/>
</body>
</html>
如何使用jQuery删除应用于.containerTitle
的样式?
有两个选择:
如果您可以删除整个样式表 (通过删除style
或link
元素),则将删除该样式表定义的所有规则。
现场示例 :
$("input").on("click", function() { $("style").remove(); // Your selector would be more specific, presumably });
.red { color: red; } .green { color: green; } .blue { color: blue; }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <div class="red">red</div> <div class="green">green</div> <div class="blue">blue</div> <input type="button" value="Click to remove the stylesheet">
或者,如果您只需要删除一条规则,则可以,但是很痛苦:浏览styleSheets
集合以找到该规则的对象 ,然后在样式表的cssRules
列表中找到相关的规则(在旧版本中称为只是rules
IE),可能是通过查看每个CSSStyleRule
的selectorText
属性,然后调用deleteRule
将其删除。
// Loop through the stylesheets...
$.each(document.styleSheets, function(_, sheet) {
// Loop through the rules...
var keepGoing = true;
$.each(sheet.cssRules || sheet.rules, function(index, rule) {
// Is this the rule we want to delete?
if (rule.selectorText === ".containerTitle") {
// Yes, do it and stop looping
sheet.deleteRule(index);
return keepGoing = false;
}
});
return keepGoing;
});
现场示例 (查看评论) :
$("input").on("click", function() { // Loop through the stylesheets... $.each(document.styleSheets, function(_, sheet) { // Loop through the rules... var keepGoing = true; $.each(sheet.cssRules || sheet.rules, function(index, rule) { // Is this the rule we want to delete? if (rule.selectorText === ".green") { // Yes, do it and stop looping sheet.deleteRule(index); return keepGoing = false; } }); return keepGoing; }); });
.red { color: red; } .green { color: green; } .blue { color: blue; }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <div class="red">red</div> <div class="green">green</div> <div class="blue">blue</div> <input type="button" value="Click to remove the green rule">
就个人而言,如果我要执行类似的操作,则可以将CSS放入易于替换/移除的body元素中。 http://jsfiddle.net/oqno5643/更改为使用html()而不是append()进行设置以删除以前的值: http : //jsfiddle.net/oqno5643/1/
的HTML
<div id="test">Test text</div>
<input type="button" id="changer" value="Change to Blue">
<span id="styleContainer"></span>
Java脚本
$(document).ready(function(){
$('#styleContainer').html('<style>#test{ color: red; }</style>');
$('#changer').on('click', function() {
$('#styleContainer').html('<style>#test{ color: blue; }</style>');
});
});
抱歉,本来打算用html()代替append(),但是在我的快速演示中忘记了。
您不能删除样式本身,但是可以从样式所应用的任何元素中删除它。
$('.containerTitle').removeClass('containerTitle');
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.