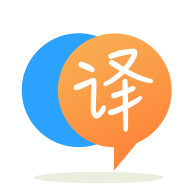
[英]decltype does not resolve nested vectors. How can I use templates for nested vectors?
[英]Can i use nested loops with vectors in cpp?
我有一个CPP问题,我不知道怎么了..也许您可以帮助我:)。 我正在尝试为图形实现数据结构。 在此图中,我将连接一些具有短欧几里得距离的节点,但是在第二次迭代中,我的迭代器将指向0x0。 仅当我将这两个节点的距离指定为std :: cout时,才会出现这种情况。 这是我的代码:
for(vector<Node*>::iterator n1 = g->getNodes().begin(); n1 != g->getNodes().end(); ++n1)
{
for(vector<Node*>::iterator n2 = g->getNodes().begin(); n2 != g->getNodes().end(); ++n2)
{
if(*n2 == 0)
{
// This will be entered after the first iteration of n2.
cout << "n2 null" << endl;
continue;
}
double distance = (*n1)->getDistance(*n2); // just euclidean distance
if(distance <= minDistance)
{
// This works fine:
cout << "(" << *n1 << "," << *n2 << ") << endl;
// This brings me a "Segmentation fault"
cout << "(" << *n1 << " , " << *n2 << ") -> " << distance << endl;
}
}
}
这是嵌套循环所欠吗? 有人能告诉我我的错吗? 非常感谢!
编辑:这是更多代码: node.h
#ifndef NODE_H_
#define NODE_H_
#include <vector>
#include <iostream>
#include <limits>
#include <math.h>
using namespace std;
class Node
{
private:
int x, y, z;
public:
Node(int x, int y, int z) : x(x), y(y), z(z)
{
}
inline int getX() { return x; }
inline int getY() { return y; }
inline int getZ() { return z; }
inline double getDistance(Node* other)
{
return sqrt(pow(x-other->getX(), 2) + pow(y-other->getY(), 2) + pow(z-other->getZ(), 2));
}
};
#endif
图
#ifndef GRAPH_H_
#define GRAPH_H_
#include <vector>
#include "node.h"
using namespace std;
class Graph
{
private:
vector<Node*> nodes;
public:
~Graph()
{
while(!nodes.empty())
{
delete nodes.back(), nodes.pop_back();
}
}
inline vector<Node*> getNodes() { return nodes; }
inline int getCountNodes() { return nodes.size(); }
bool createNode(int x, int y, int z)
{
nodes.push_back(new Node(x, y, z));
return true;
};
#endif
main.cc
#include <iostream>
#include <vector>
#include <algorithm>
#include <math.h>
#include "model/graph.h"
using namespace std;
int main()
{
Graph *g = new Graph();
int nodeDistance = 100;
for(int z = 0; z <= 300; z += nodeDistance)
{
for(int x = 0; x <= 500; x += nodeDistance)
{
for(int y = 0; y <= 300; y += nodeDistance)
{
g->createNode(x, y, z);
}
}
}
for(vector<Node*>::iterator n1 = g->getNodes().begin(); n1 != g->getNodes().end(); ++n1)
{
for(vector<Node*>::iterator n2 = g->getNodes().begin(); n2 != g->getNodes().end(); ++n2)
{
if(*n2 == 0)
{
// This will be entered after the first iteration of n2.
cout << "n2 null" << endl;
continue;
}
double distance = (*n1)->getDistance(*n2); // just euclidean distance
if(distance <= nodeDistance)
{
// This works fine:
cout << "(" << *n1 << "," << *n2 << ") << endl;
// This brings me a "Segmentation fault"
cout << "(" << *n1 << " , " << *n2 << ") -> " << distance << endl;
}
}
}
delete g;
return 0;
}
一个主要问题是您的getNodes
函数返回向量的副本,而不是原始向量。 因此,您在循环中使用的迭代器不会在同一向量上进行迭代。
相反,您在嵌套循环中使用的迭代器将迭代4个不同(但等效)的向量,而不是所讨论对象的实际向量。
通常,返回向量的副本没有什么错。 但是,当您执行此操作时,如果您确实想要一个copy而不是相同的vector,则必须确保调用此函数。 就您要完成的工作而言,使用getNodes
函数不是一种有效用法。
错误在这里:
inline vector<Node*> getNodes() { return nodes; }
解决方法:
inline vector<Node*>& getNodes() { return nodes; }
后者确保返回对所讨论的实际向量的引用,而不是实际向量的副本。 如果您仍想使用可用的功能,则可以添加一个附加功能,以将向量作为副本返回。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.